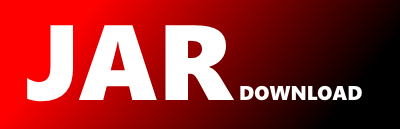
com.github.hengboy.job.schedule.ScheduleFactoryBean Maven / Gradle / Ivy
package com.github.hengboy.job.schedule;
import com.github.hengboy.job.core.http.MicroJobRestTemplate;
import com.github.hengboy.job.core.http.RestUrlConstants;
import com.github.hengboy.job.core.http.RestUrlTools;
import com.github.hengboy.job.core.thread.JobThread;
import com.github.hengboy.job.core.tools.InetAddressTools;
import com.github.hengboy.job.schedule.http.jersey.ScheduleJerseyResourceConfig;
import com.github.hengboy.job.schedule.runnable.MicroJobConsumerStateRunnable;
import com.github.hengboy.job.schedule.resource.MicroJobScheduleResource;
import com.github.hengboy.job.schedule.runnable.MicroJobExecuteQueueRunnable;
import com.github.hengboy.job.schedule.runnable.MicroJobRegistryScheduleHeartRunnable;
import com.github.hengboy.job.schedule.store.JobStore;
import lombok.Setter;
import org.glassfish.jersey.servlet.ServletContainer;
import org.glassfish.jersey.servlet.ServletProperties;
import org.quartz.Scheduler;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.InitializingBean;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.web.servlet.ServletRegistrationBean;
import org.springframework.context.SmartLifecycle;
import org.springframework.http.HttpHeaders;
import java.util.Arrays;
/**
* 微服务任务调度器工厂实体
* 该实体对外提供,用于实例化调度中心
*
* @author:于起宇
* ================================
* Created with IDEA.
* Date:2019-01-25
* Time:14:18
* 个人博客:http://blog.yuqiyu.com
* 简书:http://www.jianshu.com/u/092df3f77bca
* 码云:https://gitee.com/hengboy
* GitHub:https://github.com/hengyuboy
* ================================
*
*/
public class ScheduleFactoryBean extends ServletRegistrationBean implements InitializingBean, SmartLifecycle {
/**
* logger instance
*/
static Logger logger = LoggerFactory.getLogger(ScheduleFactoryBean.class);
/**
* 调度器负载的权重
*/
@Setter
private int loadBalanceWeight = 1;
/**
* 任务注册中心监听端口号
*/
@Setter
private int registryPort;
/**
* 任务注册中心ip地址
*/
@Setter
private String registryIpAddress;
/**
* 调度器绑定的端口号
*/
@Setter
private int port;
/**
* 心跳同步执行间隔时间,单位:秒
*/
@Setter
private int heartDelaySeconds = 5;
/**
* 消费者状态检查执行间隔时间,单位:秒
*/
@Setter
private int consumerDelaySeconds = 5;
/**
* 设置最大重试次数
*/
@Setter
private int maxRetryTimes = 2;
/**
* 任务数据源对象
*/
@Autowired
private JobStore jobStore;
/**
* quartz 调度对象
*/
@Autowired
private Scheduler scheduler;
/**
* restTemplate实例
*/
@Autowired
private MicroJobRestTemplate restTemplate;
/**
* 调度器运行状态
*/
private boolean running;
/**
* 构造函数初始化相关配置
*/
public ScheduleFactoryBean() {
// 设置SpringMvc排除路径前缀
setUrlMappings(Arrays.asList(RestUrlConstants.PREFIX + "/*"));
// 设置servlet
setServlet(new ServletContainer());
// 添加初始化参数,根据ScheduleJerseyResourceConfig资源ws配置类进行实例化
addInitParameter(ServletProperties.JAXRS_APPLICATION_CLASS, ScheduleJerseyResourceConfig.class.getName());
}
/**
* 初始化方法
* - 动态创建任务上报的RPC Server服务
* - 开启注册中心心跳检查线程
*/
@Override
public void start() {
// 开启心跳检查线程
JobThread.scheduleWithFixedDelay(new MicroJobRegistryScheduleHeartRunnable(), 1, heartDelaySeconds);
// 开启任务执行线程
JobThread.execute(new MicroJobExecuteQueueRunnable());
// 开启任务消费者状态监听
JobThread.scheduleWithFixedDelay(new MicroJobConsumerStateRunnable(), 1, consumerDelaySeconds);
logger.info("Job scheduling center start successfully");
running = true;
}
/**
* 调度器被销毁时的处理
* - 上报任务注册中心、任务下线、断开调度连接
*/
@Override
public void stop() {
// 格式化调度器下线路径
String downInstanceUrl = RestUrlTools.formatter(registryIpAddress, registryPort, RestUrlConstants.REGISTRY_DOWN_FULL_URL);
// 执行调度器下线
restTemplate.postJsonEntity(downInstanceUrl, "", HttpHeaders.EMPTY, Void.class, InetAddressTools.formatterAddress(MicroJobScheduleResource.getIpAddress(), MicroJobScheduleResource.getPort()));
running = false;
}
@Override
public boolean isRunning() {
return running;
}
/**
* 设置全局的资源信息
*
* @throws Exception 异常信息
*/
@Override
public void afterPropertiesSet() throws Exception {
// restTemplate setter
MicroJobScheduleResource.setRestTemplate(restTemplate);
// 设置注册中心ip地址
MicroJobScheduleResource.setRegistryIpAddress(registryIpAddress);
// 设置注册中心port
MicroJobScheduleResource.setRegistryPort(registryPort);
// 任务存储数据源实例
MicroJobScheduleResource.setJobStore(jobStore);
// quartz 调度器实例
MicroJobScheduleResource.setScheduler(scheduler);
// 当前任务调度器负载均衡权重
MicroJobScheduleResource.setScheduleLbWeight(loadBalanceWeight);
// 设置任务执行最大重试次数
MicroJobScheduleResource.setMaxRetryTimes(maxRetryTimes);
// 设置调度器当前ip地址
MicroJobScheduleResource.setIpAddress(InetAddressTools.getLocalIp());
// 调度器绑定的端口号
MicroJobScheduleResource.setPort(port);
logger.info("Global resource setup completed");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy