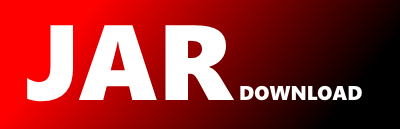
com.github.hengboy.job.schedule.quartz.QuartzJobContext Maven / Gradle / Ivy
/*
* Copyright 2019 恒宇少年
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.github.hengboy.job.schedule.quartz;
import com.alibaba.fastjson.JSON;
import com.github.hengboy.job.core.constants.QuartzJobConstants;
import com.github.hengboy.job.core.wrapper.JobWrapper;
import com.github.hengboy.job.core.wrapper.support.CronJobWrapper;
import com.github.hengboy.job.core.wrapper.support.LoopJobWrapper;
import com.github.hengboy.job.core.wrapper.support.OnceJobWrapper;
import com.github.hengboy.job.schedule.quartz.bean.MicroJobExecuteBean;
import org.quartz.*;
import org.springframework.scheduling.quartz.QuartzJobBean;
/**
* quartz job 生成工具类
*
* @author:于起宇
* ================================
* Created with IDEA.
* Date:2019-01-14
* Time:13:30
* 个人博客:http://blog.yuqiyu.com
* 简书:http://www.jianshu.com/u/092df3f77bca
* 码云:https://gitee.com/hengboy
* GitHub:https://github.com/hengyuboy
* ================================
*
*/
public class QuartzJobContext {
/**
* 默认的任务执行类的类型
* 用于quartz的分组以及执行类
*/
private static Class extends QuartzJobBean> DEFAULT_JOB_CLASS = MicroJobExecuteBean.class;
/**
* 创建cron类型的quartz任务
*
* @param scheduler 任务调度器
* @param wrapper cron任务封装对象
* @param jobQueueId 任务队列主键
* @throws SchedulerException 调度器异常
*/
public static void newCronJob(Scheduler scheduler, CronJobWrapper wrapper, String jobQueueId) throws SchedulerException {
// 获取任务的执行详情
JobDetail jobDetail = getJobDetail(wrapper, jobQueueId);
// 触发器key
TriggerKey triggerKey = TriggerKey.triggerKey(jobQueueId, wrapper.getJobKey());
// cron触发器
Trigger trigger = TriggerBuilder.newTrigger().withIdentity(triggerKey).withSchedule(CronScheduleBuilder.cronSchedule(wrapper.getCron())).build();
// 任务添加到调度中心
scheduler.scheduleJob(jobDetail, trigger);
}
/**
* 创建 loop 类型的quartz任务
*
* @param scheduler 任务调度器
* @param wrapper loop任务封装对象
* @param jobQueueId 任务队列主键
* @throws SchedulerException 调度器异常
*/
public static void newLoopJob(Scheduler scheduler, LoopJobWrapper wrapper, String jobQueueId) throws SchedulerException {
JobDetail jobDetail = getJobDetail(wrapper, jobQueueId);
// 触发器key
TriggerKey triggerKey = TriggerKey.triggerKey(jobQueueId, wrapper.getJobKey());
// 循环触发器
Trigger trigger = TriggerBuilder.newTrigger().withIdentity(triggerKey).withSchedule(SimpleScheduleBuilder.simpleSchedule().withRepeatCount(wrapper.getLoopTimes()).withIntervalInMilliseconds(wrapper.getLoopIntervalTime())).startAt(wrapper.getStartAtTime()).build();
// 任务添加到调度中心
scheduler.scheduleJob(jobDetail, trigger);
}
/**
* 创建 once 类型的quartz任务
*
* @param scheduler 任务调度器
* @param wrapper once任务封装对象
* @param jobQueueId 任务队列主键
* @throws SchedulerException 调度异常
*/
public static void newOnceJob(Scheduler scheduler, OnceJobWrapper wrapper, String jobQueueId) throws SchedulerException {
JobDetail jobDetail = getJobDetail(wrapper, jobQueueId);
// 触发器key
TriggerKey triggerKey = TriggerKey.triggerKey(jobQueueId, wrapper.getJobKey());
// 循环触发器
Trigger trigger = TriggerBuilder.newTrigger().withIdentity(triggerKey).withSchedule(SimpleScheduleBuilder.simpleSchedule()).startAt(wrapper.getStartAtTime()).build();
// 任务添加到调度中心
scheduler.scheduleJob(jobDetail, trigger);
}
/**
* 检查任务是否存在
*
* @param scheduler quartz 调度对象
* @param jobQueueId micro job consumer上报任务定义的队列id,用于quartz的job Name
* @param jobKey micro job consumer上报任务的key,用于quartz的job Group
* @return true:存在,false:不存在
* @throws SchedulerException 调度异常
*/
public static boolean checkExists(Scheduler scheduler, String jobQueueId, String jobKey) throws SchedulerException {
return scheduler.checkExists(new JobKey(jobQueueId, jobKey));
}
/**
* 删除任务
*
* @param scheduler quartz 调度对象
* @param jobQueueId 任务队列主键
* @param jobKey 任务队列所属任务定义的key
* @throws SchedulerException 调度异常
*/
public static void removeJob(Scheduler scheduler, String jobQueueId, String jobKey) throws SchedulerException {
scheduler.deleteJob(new JobKey(jobQueueId, jobKey));
}
/**
* 任务执行详情构建
*
* @param wrapper 任务封装对象
* @return quartz任务详情
*/
private static JobDetail getJobDetail(JobWrapper wrapper, String jobQueueId) {
// 封装job key
JobKey jobKey = JobKey.jobKey(jobQueueId, wrapper.getJobKey());
// 创建job detail
JobDetail jobDetail = JobBuilder.newJob(DEFAULT_JOB_CLASS).withIdentity(jobKey).build();
// 参数设置
if (wrapper.getParam() != null) {
jobDetail.getJobDataMap().put(QuartzJobConstants.NODE_JOB_PARAM, JSON.toJSONString(wrapper.getParam()));
}
return jobDetail;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy