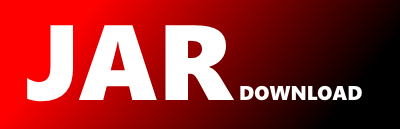
com.github.hengboy.job.schedule.runnable.MicroJobConsumerStateRunnable Maven / Gradle / Ivy
/*
* Copyright [2019] [恒宇少年 - 于起宇]
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.github.hengboy.job.schedule.runnable;
import com.github.hengboy.job.core.http.MicroJobRestTemplate;
import com.github.hengboy.job.core.http.RestUrlConstants;
import com.github.hengboy.job.core.http.RestUrlTools;
import com.github.hengboy.job.core.http.model.request.InstanceInfo;
import com.github.hengboy.job.core.http.model.request.InstanceInfos;
import com.github.hengboy.job.core.strategy.model.LoadBalanceNode;
import com.github.hengboy.job.schedule.resource.MicroJobScheduleResource;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.http.HttpHeaders;
import org.springframework.util.ObjectUtils;
/**
* 开启线程执行实时同步消费者列表
*
* @author:恒宇少年 - 于起宇
*
* DateTime:2019-01-31 13:48
* Blog:http://blog.yuqiyu.com
* WebSite:http://www.jianshu.com/u/092df3f77bca
* Gitee:https://gitee.com/hengboy
* GitHub:https://github.com/hengyuboy
*/
public class MicroJobConsumerStateRunnable implements Runnable {
/**
* logger instance
*/
static Logger logger = LoggerFactory.getLogger(MicroJobConsumerStateRunnable.class);
/**
* 线程处理同步消费者列表
*/
@Override
public void run() {
try {
// 获取任务注册中心内的消费者列表
MicroJobRestTemplate restTemplate = MicroJobScheduleResource.getRestTemplate();
String getAllInstancesUrl = RestUrlTools.formatter(MicroJobScheduleResource.getRegistryIpAddress(), MicroJobScheduleResource.getRegistryPort(), RestUrlConstants.REGISTRY_GET_ALL_INSTANCE_FULL_URL);
InstanceInfos instanceInfos = restTemplate.getForObject(getAllInstancesUrl, HttpHeaders.EMPTY, InstanceInfos.class, InstanceInfo.InstanceType.CONSUMER);
if (!ObjectUtils.isEmpty(instanceInfos)) {
instanceInfos.getInstanceInfos().stream().forEach(registryConsumer -> {
// 查询是否之前存在该节点
LoadBalanceNode loadBalanceNode = MicroJobScheduleResource.getConsumerLbNode(registryConsumer.getInstanceAddress());
if (loadBalanceNode == null) {
// 如果调度器状态为"UP"时
if (InstanceInfo.InstanceStatus.UP.toString().equals(registryConsumer.getInstanceStatus().toString())) {
// 执行添加调度器到缓存内
MicroJobScheduleResource.addConsumerLbNode(new LoadBalanceNode(registryConsumer.getLoadBalanceWeight(), registryConsumer.getInstanceAddress(), registryConsumer.getLoadBalanceWeight()));
}
}
// 之前存在该节点
else {
// 如果调度器状态为"DOWN"时
if (InstanceInfo.InstanceStatus.DOWN.toString().equals(registryConsumer.getInstanceStatus().toString())) {
// 删除负载节点缓存信息
MicroJobScheduleResource.removeConsumerLbNode(registryConsumer.getInstanceAddress());
}
}
logger.info("Job execution consumer: [{}] -> [{}] Real-time status update completed.", registryConsumer.getInstanceAddress(), registryConsumer.getInstanceStatus().toString());
});
}
} catch (Exception e) {
logger.error("Update scheduler status encounter exception information", e);
}
}
}