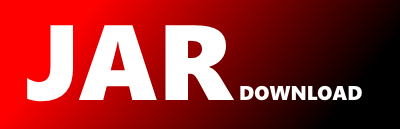
com.github.hengboy.job.schedule.store.delegate.DriverDelegate Maven / Gradle / Ivy
/*
* Copyright [2019] [恒宇少年 - 于起宇]
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.github.hengboy.job.schedule.store.delegate;
import com.github.hengboy.job.core.exception.JobException;
import com.github.hengboy.job.core.wrapper.JobWrapper;
import com.github.hengboy.job.schedule.store.model.JobDetail;
import com.github.hengboy.job.schedule.store.model.JobExecuteLog;
import com.github.hengboy.job.schedule.store.model.JobExecuteQueue;
import com.github.hengboy.job.schedule.store.model.JobExecuteSchedule;
import java.sql.Connection;
import java.sql.Timestamp;
/**
* 这是所有数据库驱动程序委托类的基本接口。
*
* @author:恒宇少年 - 于起宇
*
* DateTime:2019-01-28 14:34
* Blog:http://blog.yuqiyu.com
* WebSite:http://www.jianshu.com/u/092df3f77bca
* Gitee:https://gitee.com/hengboy
* GitHub:https://github.com/hengyuboy
*/
public interface DriverDelegate {
/**
* 初始化delegate所需要的相关配置信息
*
* @param tablePrefix 表前缀
*/
void initialize(String tablePrefix);
/**
* 添加任务定义的基本信息
*
* @param connection 数据库链接
* @param jobWrapper 任务封装对象
* @return 任务定义的主键值
* @throws JobException 任务异常
*/
String addJob(Connection connection, JobWrapper jobWrapper) throws JobException;
/**
* 根据任务定义的key查询任务定义信息
*
* @param connection 数据库连接
* @param jobKey 任务定义的key
* @return 任务详情
* @throws JobException 任务异常
*/
JobDetail selectJobByKey(Connection connection, String jobKey) throws JobException;
/**
* 根据任务定义的主键查询任务定义信息
*
* @param connection 数据库连接
* @param jobId 任务定义主键
* @return 任务详情
* @throws JobException 任务异常
*/
JobDetail selectJobById(Connection connection, String jobId) throws JobException;
/**
* 添加任务队列基本信息
*
* @param connection 数据库链接
* @param newJobId 新任务编号
* @param jobWrapper 任务封装对象
* @return 任务队列的主键值
* @throws JobException 任务异常
*/
String addJobQueue(Connection connection, String newJobId, JobWrapper jobWrapper) throws JobException;
/**
* 更新任务队列cron表达式
*
* @param connection 数据库链接
* @param jobQueueId 任务队列主键
* @param cron cron表达式
* @throws JobException 任务异常
*/
void updateJobQueueCron(Connection connection, String jobQueueId, String cron) throws JobException;
/**
* 更新loop类型任务所需要的配置
*
* @param connection 数据库链接
* @param jobQueueId 任务队列主键
* @param loopTimes 循环次数
* @param loopIntervalMillis 每次的间隔毫秒值
* @throws JobException 任务异常
*/
void updateJobQueueLoop(Connection connection, String jobQueueId, int loopTimes, long loopIntervalMillis) throws JobException;
/**
* 根据自定义的任务队列key进行删除任务
*
* @param connection 数据库链接
* @param jobQueueId 任务队列主键
* @throws JobException 任务异常
*/
void removeJobQueue(Connection connection, String jobQueueId) throws JobException;
/**
* 根据任务队列主键查询任务队列信息
*
* @param connection 数据库连接
* @param jobQueueId 任务队列主键值
* @return 任务执行队列
* @throws JobException 任务异常
*/
JobExecuteQueue selectQueueById(Connection connection, String jobQueueId) throws JobException;
/**
* 根据任务队列自定义key查询队列信息
*
* @param connection 数据库连接
* @param jobQueueKey 任务队列自定义key
* @return 任务执行队列
* @throws JobException 任务异常
*/
JobExecuteQueue selectQueueByKey(Connection connection, String jobQueueKey) throws JobException;
/**
* 添加任务执行日志
*
* @param connection 数据库连接
* @param jobExecuteLog 任务执行日志数据实体
* @throws JobException 任务异常
*/
void addLog(Connection connection, JobExecuteLog jobExecuteLog) throws JobException;
/**
* 根据任务队列主键值查询日志信息
*
* @param connection 数据库连接
* @param jobLogId 任务日志编号
* @return 任务执行日志
* @throws JobException 任务异常
*/
JobExecuteLog selectLog(Connection connection, String jobLogId) throws JobException;
/**
* 更新日志状态
*
* @param connection 数据库连接
* @param jobLogId 任务日志编号
* @param logState 任务日志状态
* @throws JobException 任务异常
*/
void updateLogState(Connection connection, String jobLogId, String logState) throws JobException;
/**
* 更新日志消费者地址
*
* @param connection 数据库连接
* @param jobLogId 任务日志编号
* @param consumerAddress 任务消费者地址
* @throws JobException 任务异常
*/
void updateLogConsumerAddress(Connection connection, String jobLogId, String consumerAddress) throws JobException;
/**
* 更新日志重试次数
*
* @param connection 数据库连接
* @param jobLogId 任务日志编号
* @param retryCount 重试次数
* @throws JobException 任务异常
*/
void updateLogRetryCount(Connection connection, String jobLogId, int retryCount) throws JobException;
/**
* 更新任务日志执行成功时间
*
* @param connection 数据库连接
* @param jobLogId 任务日志编号
* @param successTime 执行成功时间
* @throws JobException 任务异常
*/
void updateLogSuccessTime(Connection connection, String jobLogId, Timestamp successTime) throws JobException;
/**
* 添加任务执行调度器信息
*
* @param connection 数据库连接
* @param jobExecuteSchedule 任务调度器数据实体
* @return 调度器主键
*/
String addSchedule(Connection connection, JobExecuteSchedule jobExecuteSchedule) throws JobException;
/**
* 更新任务调度器状态
*
* @param connection 数据库连接
* @param scheduleAddress 调度器地址
* @param scheduleState 调度器运行状态
* @throws JobException 任务异常
*/
void updateScheduleState(Connection connection, String scheduleAddress, String scheduleState) throws JobException;
/**
* 删除任务调度器
*
* @param connection 数据库连接
* @param scheduleAddress 任务调度器地址
* @throws JobException 任务异常
*/
void removeSchedule(Connection connection, String scheduleAddress) throws JobException;
}