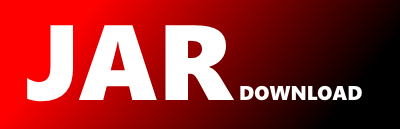
com.github.hengboy.job.schedule.store.delegate.JdbcSqlDelegate Maven / Gradle / Ivy
/*
* Copyright [2019] [恒宇少年 - 于起宇]
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.github.hengboy.job.schedule.store.delegate;
import com.alibaba.fastjson.JSON;
import com.github.hengboy.job.core.exception.JobException;
import com.github.hengboy.job.core.wrapper.JobWrapper;
import com.github.hengboy.job.schedule.store.JdbcSqlConstants;
import com.github.hengboy.job.schedule.store.model.JobDetail;
import com.github.hengboy.job.schedule.store.model.JobExecuteLog;
import com.github.hengboy.job.schedule.store.model.JobExecuteQueue;
import com.github.hengboy.job.schedule.store.model.JobExecuteSchedule;
import com.google.common.base.CaseFormat;
import java.lang.reflect.Field;
import java.sql.*;
import java.util.LinkedList;
import java.util.List;
import java.util.UUID;
/**
* jdbc方式驱动委托实现类
* 通过datasource的链接操作原生sql来处理数据
*
* @author:恒宇少年 - 于起宇
*
* DateTime:2019-01-28 14:41
* Blog:http://blog.yuqiyu.com
* WebSite:http://www.jianshu.com/u/092df3f77bca
* Gitee:https://gitee.com/hengboy
* GitHub:https://github.com/hengyuboy
*/
public class JdbcSqlDelegate implements DriverDelegate, JdbcSqlConstants {
/**
* 表前缀信息
*/
private String tablePrefix;
/**
* 初始化delegate所需要的相关配置信息
*
* @param tablePrefix 表前缀
*/
@Override
public void initialize(String tablePrefix) {
this.tablePrefix = tablePrefix;
}
/**
* 添加任务定义的基本信息
*
* @param connection 数据库链接
* @param jobWrapper 任务封装对象
* @return 任务定义的主键值
* @throws JobException 任务异常
*/
@Override
public String addJob(Connection connection, JobWrapper jobWrapper) throws JobException {
// 新任务的主键值
String newJobId = UUID.randomUUID().toString();
PreparedStatement ps = null;
try {
ps = connection.prepareStatement(replaceTablePrefix(INSERT_DETAIL));
ps.setString(1, newJobId);
ps.setString(2, jobWrapper.getJobKey());
ps.setString(3, jobWrapper.getStrategy().toString());
ps.setInt(4, jobWrapper.getWeight());
ps.executeUpdate();
} catch (SQLException e) {
throw new JobException("Execute addJob exception:" + e.getMessage(), e);
} finally {
closeStatement(ps);
}
return newJobId;
}
/**
* 查询任务定义基本信息
*
* @param connection 数据库连接
* @param jobKey 任务定义key
* @return 任务详情
* @throws JobException 任务异常
*/
@Override
public JobDetail selectJobByKey(Connection connection, String jobKey) throws JobException {
PreparedStatement ps = null;
ResultSet rs = null;
JobDetail jobDetail;
try {
ps = connection.prepareStatement(replaceTablePrefix(SELECT_JOB_DETAIL_WITH_KEY));
ps.setString(1, jobKey);
// 执行查询
rs = ps.executeQuery();
// 执行结果封装
jobDetail = wrapperOneResult(JobDetail.class, rs);
} catch (SQLException e) {
throw new JobException("Execute selectJobByKey exception:" + e.getMessage(), e);
} finally {
closeStatement(ps);
closeResultSet(rs);
}
return jobDetail;
}
/**
* 根据任务定义的主键查询任务定义信息
*
* @param connection 数据库连接
* @param jobId 任务定义主键
* @return 任务详情
* @throws JobException 任务异常
*/
@Override
public JobDetail selectJobById(Connection connection, String jobId) throws JobException {
PreparedStatement ps = null;
ResultSet rs = null;
JobDetail jobDetail;
try {
ps = connection.prepareStatement(replaceTablePrefix(SELECT_JOB_DETAIL_WITH_ID));
ps.setString(1, jobId);
// 执行查询
rs = ps.executeQuery();
// 执行结果封装
jobDetail = wrapperOneResult(JobDetail.class, rs);
} catch (SQLException e) {
throw new JobException("Execute selectJobById exception:" + e.getMessage(), e);
} finally {
closeStatement(ps);
closeResultSet(rs);
}
return jobDetail;
}
/**
* 添加任务队列基本信息
*
* @param connection 数据库链接
* @param newJobId 所属定义任务的主键值
* @param jobWrapper 任务封装对象
* @return 任务队列的主键值
* @throws JobException 任务异常
*/
@Override
public String addJobQueue(Connection connection, String newJobId, JobWrapper jobWrapper) throws JobException {
// 新任务的主键值
String newJobQueueId = UUID.randomUUID().toString();
PreparedStatement ps = null;
try {
ps = connection.prepareStatement(replaceTablePrefix(INSERT_EXECUTE_QUEUE));
ps.setString(1, newJobQueueId);
ps.setString(2, jobWrapper.getJobQueueKey());
ps.setString(3, newJobId);
ps.setString(4, jobWrapper.getJobExecuteAway().toString());
ps.setString(5, JSON.toJSONString(jobWrapper.getParam()));
ps.setString(6, jobWrapper.getStrategy().toString());
ps.setInt(7, jobWrapper.getWeight());
ps.executeUpdate();
} catch (SQLException e) {
throw new JobException("Execute addJobQueue exception:" + e.getMessage(), e);
} finally {
closeStatement(ps);
}
return newJobQueueId;
}
/**
* 更新任务队列cron表达式
*
* @param connection 数据库链接
* @param jobQueueId 任务队列主键
* @param cron cron表达式
* @throws JobException 任务异常
*/
@Override
public void updateJobQueueCron(Connection connection, String jobQueueId, String cron) throws JobException {
PreparedStatement ps = null;
try {
ps = connection.prepareStatement(replaceTablePrefix(UPDATE_EXECUTE_QUEUE_CRON));
ps.setString(1, cron);
ps.setString(2, jobQueueId);
ps.executeUpdate();
} catch (SQLException e) {
throw new JobException("Execute updateJobQueueCron exception:" + e.getMessage(), e);
} finally {
closeStatement(ps);
}
}
/**
* 更新loop类型任务所需要的配置
*
* @param connection 数据库链接
* @param jobQueueId 任务队列主键
* @param loopTimes 循环次数
* @param loopIntervalMillis 每次的间隔毫秒值
* @throws JobException 任务异常
*/
@Override
public void updateJobQueueLoop(Connection connection, String jobQueueId, int loopTimes, long loopIntervalMillis) throws JobException {
PreparedStatement ps = null;
try {
ps = connection.prepareStatement(replaceTablePrefix(UPDATE_EXECUTE_QUEUE_LOOP));
ps.setInt(1, loopTimes);
ps.setLong(2, loopIntervalMillis);
ps.setString(3, jobQueueId);
ps.executeUpdate();
} catch (SQLException e) {
throw new JobException("Execute updateJobQueueCron exception:" + e.getMessage(), e);
} finally {
closeStatement(ps);
}
}
/**
* 根据自定义的任务队列key进行删除任务
*
* @param connection 数据库链接
* @param jobQueueId 任务队列主键
* @throws JobException 任务异常
*/
@Override
public void removeJobQueue(Connection connection, String jobQueueId) throws JobException {
PreparedStatement ps = null;
try {
ps = connection.prepareStatement(replaceTablePrefix(REMOVE_EXECUTE_QUEUE));
ps.setString(1, jobQueueId);
ps.executeUpdate();
} catch (SQLException e) {
throw new JobException("Execute removeJobQueue exception:" + e.getMessage(), e);
} finally {
closeStatement(ps);
}
}
/**
* 根据任务队列key查询任务队列信息
*
* @param connection 数据库连接
* @param jobQueueId 任务队列主键
* @return 任务执行队列
* @throws JobException 任务异常
*/
@Override
public JobExecuteQueue selectQueueById(Connection connection, String jobQueueId) throws JobException {
PreparedStatement ps = null;
ResultSet rs = null;
JobExecuteQueue jobExecuteQueue;
try {
ps = connection.prepareStatement(replaceTablePrefix(SELECT_EXECUTE_QUEUE_DETAIL));
ps.setString(1, jobQueueId);
// 执行查询
rs = ps.executeQuery();
// 执行结果封装
jobExecuteQueue = wrapperOneResult(JobExecuteQueue.class, rs);
} catch (SQLException e) {
throw new JobException("Execute selectQueueById exception:" + e.getMessage(), e);
} finally {
closeStatement(ps);
closeResultSet(rs);
}
return jobExecuteQueue;
}
/**
* 根据任务队列key查询队列信息
*
* @param connection 数据库连接
* @param jobQueueKey 任务队列自定义key
* @return 任务执行队列
* @throws JobException 任务异常
*/
@Override
public JobExecuteQueue selectQueueByKey(Connection connection, String jobQueueKey) throws JobException {
PreparedStatement ps = null;
ResultSet rs = null;
JobExecuteQueue jobExecuteQueue;
try {
ps = connection.prepareStatement(replaceTablePrefix(SELECT_EXECUTE_QUEUE_DETAIL_BY_KEY));
ps.setString(1, jobQueueKey);
// 执行查询
rs = ps.executeQuery();
// 执行结果封装
jobExecuteQueue = wrapperOneResult(JobExecuteQueue.class, rs);
} catch (SQLException e) {
throw new JobException("Execute selectQueueByKey exception:" + e.getMessage(), e);
} finally {
closeStatement(ps);
closeResultSet(rs);
}
return jobExecuteQueue;
}
/**
* 添加任务执行日志
*
* @param connection 数据库连接
* @param jobExecuteLog 任务执行日志数据实体
* @throws JobException 任务异常
*/
@Override
public void addLog(Connection connection, JobExecuteLog jobExecuteLog) throws JobException {
PreparedStatement ps = null;
try {
ps = connection.prepareStatement(replaceTablePrefix(INSERT_EXECUTE_LOG));
ps.setString(1, jobExecuteLog.getJelId());
ps.setString(2, jobExecuteLog.getJelScheduleId());
ps.setString(3, jobExecuteLog.getJelQueueId());
ps.setString(4, jobExecuteLog.getJelNamespace());
ps.executeUpdate();
} catch (SQLException e) {
throw new JobException("Execute addLog exception:" + e.getMessage(), e);
} finally {
closeStatement(ps);
}
}
/**
* 根据任务队列主键值查询日志信息
*
* @param connection 数据库连接
* @param jobLogId 任务日志编号
* @return 任务执行日志
* @throws JobException 任务异常
*/
@Override
public JobExecuteLog selectLog(Connection connection, String jobLogId) throws JobException {
PreparedStatement ps = null;
ResultSet rs = null;
JobExecuteLog jobExecuteLog;
try {
ps = connection.prepareStatement(replaceTablePrefix(SELECT_EXECUTE_LOG_DETAIL));
ps.setString(1, jobLogId);
// 执行查询
rs = ps.executeQuery();
// 执行结果封装
jobExecuteLog = wrapperOneResult(JobExecuteLog.class, rs);
} catch (SQLException e) {
throw new JobException("Execute selectLog exception:" + e.getMessage(), e);
} finally {
closeStatement(ps);
closeResultSet(rs);
}
return jobExecuteLog;
}
/**
* 更新日志状态
*
* @param connection 数据库连接
* @param jobLogId 任务日志编号
* @param logState 日志状态
* @throws JobException 任务异常
*/
@Override
public void updateLogState(Connection connection, String jobLogId, String logState) throws JobException {
PreparedStatement ps = null;
try {
ps = connection.prepareStatement(replaceTablePrefix(UPDATE_EXECUTE_LOG_STATE));
ps.setString(1, logState);
ps.setString(2, jobLogId);
ps.executeUpdate();
} catch (SQLException e) {
throw new JobException("Execute updateLogState exception:" + e.getMessage(), e);
} finally {
closeStatement(ps);
}
}
/**
* 更新日志消费者地址
*
* @param connection 数据库连接
* @param jobLogId 任务日志编号
* @param consumerAddress 任务消费者地址
* @throws JobException 任务异常
*/
@Override
public void updateLogConsumerAddress(Connection connection, String jobLogId, String consumerAddress) throws JobException {
PreparedStatement ps = null;
try {
ps = connection.prepareStatement(replaceTablePrefix(UPDATE_EXECUTE_LOG_CONSUMER_ADDRESS));
ps.setString(1, jobLogId);
ps.setString(2, consumerAddress);
ps.executeUpdate();
} catch (SQLException e) {
throw new JobException("Execute updateLogConsumerAddress exception:" + e.getMessage(), e);
} finally {
closeStatement(ps);
}
}
/**
* 更新日志重试次数
*
* @param connection 数据库连接
* @param jobLogId 任务日志编号
* @param retryCount 重试次数
* @throws JobException 任务异常
*/
@Override
public void updateLogRetryCount(Connection connection, String jobLogId, int retryCount) throws JobException {
PreparedStatement ps = null;
try {
ps = connection.prepareStatement(replaceTablePrefix(UPDATE_EXECUTE_LOG_RETRY_COUNT));
ps.setString(1, jobLogId);
ps.setInt(2, retryCount);
ps.executeUpdate();
} catch (SQLException e) {
throw new JobException("Execute updateLogRetryCount exception:" + e.getMessage(), e);
} finally {
closeStatement(ps);
}
}
/**
* 更新任务日志执行成功时间
*
* @param connection 数据库连接
* @param jobLogId 任务日志编号
* @param successTime 执行成功时间
* @throws JobException 任务异常
*/
@Override
public void updateLogSuccessTime(Connection connection, String jobLogId, Timestamp successTime) throws JobException {
PreparedStatement ps = null;
try {
ps = connection.prepareStatement(replaceTablePrefix(UPDATE_EXECUTE_LOG_SUCCESS_TIME));
ps.setString(1, jobLogId);
ps.setTimestamp(2, successTime);
ps.executeUpdate();
} catch (SQLException e) {
throw new JobException("Execute updateLogSuccessTime exception:" + e.getMessage(), e);
} finally {
closeStatement(ps);
}
}
/**
* 添加任务执行调度器信息
*
* @param connection 数据库连接
* @param jobExecuteSchedule 任务调度器数据实体
*/
@Override
public String addSchedule(Connection connection, JobExecuteSchedule jobExecuteSchedule) throws JobException {
PreparedStatement ps = null;
String scheduleId = UUID.randomUUID().toString();
try {
ps = connection.prepareStatement(replaceTablePrefix(INSERT_EXECUTE_SCHEDULE));
ps.setString(1, scheduleId);
ps.setString(2, jobExecuteSchedule.getJesAddress());
ps.executeUpdate();
} catch (SQLException e) {
throw new JobException("Execute addSchedule exception:" + e.getMessage(), e);
} finally {
closeStatement(ps);
}
return scheduleId;
}
/**
* 更新任务调度器状态
*
* @param connection 数据库连接
* @param scheduleAddress 调度器地址
* @param scheduleState 调度器运行状态
* @throws JobException 任务异常
*/
@Override
public void updateScheduleState(Connection connection, String scheduleAddress, String scheduleState) throws JobException {
PreparedStatement ps = null;
try {
ps = connection.prepareStatement(replaceTablePrefix(UPDATE_EXECUTE_SCHEDULE_STATE));
ps.setString(1, scheduleState);
ps.setString(2, scheduleAddress);
ps.executeUpdate();
} catch (SQLException e) {
throw new JobException("Execute updateScheduleState exception:" + e.getMessage(), e);
} finally {
closeStatement(ps);
}
}
/**
* 删除任务调度器
*
* @param connection 数据库连接
* @param scheduleAddress 任务调度器地址
* @throws JobException 任务异常
*/
@Override
public void removeSchedule(Connection connection, String scheduleAddress) throws JobException {
PreparedStatement ps = null;
try {
ps = connection.prepareStatement(replaceTablePrefix(REMOVE_EXECUTE_SCHEDULE));
ps.setString(1, scheduleAddress);
ps.executeUpdate();
} catch (SQLException e) {
throw new JobException("Execute removeSchedule exception:" + e.getMessage(), e);
} finally {
closeStatement(ps);
}
}
/**
* 替换表前缀
*
* @param executeSql 执行的sql
* @return 替换表名后的sql
*/
protected String replaceTablePrefix(String executeSql) {
return String.format(executeSql, tablePrefix);
}
/**
* Cleanup helper method that closes the given ResultSet
* while ignoring any errors.
*
* @param rs restSet对象
*/
protected static void closeResultSet(ResultSet rs) {
if (null != rs) {
try {
rs.close();
} catch (SQLException ignore) {
}
}
}
/**
* Cleanup helper method that closes the given Statement
* while ignoring any errors.
*
* @param statement Statement执行对象
*/
protected static void closeStatement(Statement statement) {
if (null != statement) {
try {
statement.close();
} catch (SQLException ignore) {
}
}
}
/**
* 封装处理单个结果
*
* 约定:返回对象的字段遵循首字母小写,"_"后第一个字母大写规则命名(对应列名)
*
* @param resultClass 返回对象类型
* @param rs 查询结果集
* @param 返回对象泛型类型
* @return 新对象
*/
protected T wrapperOneResult(Class resultClass, ResultSet rs) throws JobException {
Object resultObj;
try {
// 实例化返回对象
resultObj = resultClass.newInstance();
// 查询结果的元数据信息
ResultSetMetaData metaData = rs.getMetaData();
int columnCount = metaData.getColumnCount();
while (rs.next()) {
// 遍历元数据内的列信息,根据列名进行设置到返回对象的对应字段
for (int index = 1; index < columnCount + 1; index++) {
// 列名
String columnName = metaData.getColumnName(index);
Object columnValue = rs.getObject(columnName);
if (columnValue != null) {
// 对应字段
Field field = resultClass.getDeclaredField(columnNameToFieldName(columnName));
field.setAccessible(true);
// 设置字段的值
field.set(resultObj, columnValue);
}
}
}
} catch (Exception e) {
throw new JobException("Encapsulation result set object encounters exception information:" + e.getMessage(), e);
}
return (T) resultObj;
}
/**
* 封装处理列表结果
*
* @param resultClass 返回对象类型
* @param rs 查询的结果集
* @param 返回的泛型类型
* @return 封装集合结果
* @throws JobException 任务异常
*/
protected List wrapperListResult(Class resultClass, ResultSet rs) throws JobException {
List resultList = new LinkedList();
resultList.add(wrapperOneResult(resultClass, rs));
return resultList;
}
/**
* 列名转换Field的名称
*
* @param columnName 列名
* @return Field名称
*/
private String columnNameToFieldName(String columnName) {
// 默认field为全小写的列名
String fieldName = columnName.toLowerCase();
// 返回转换驼峰后的字符串
return CaseFormat.LOWER_UNDERSCORE.to(CaseFormat.LOWER_CAMEL, fieldName);
}
}