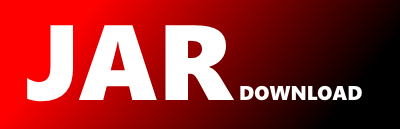
com.github.hetianyi.common.util.ArchiveUtil Maven / Gradle / Ivy
package com.github.hetianyi.common.util;
import org.apache.commons.compress.archivers.ArchiveEntry;
import org.apache.commons.compress.archivers.ArchiveInputStream;
import org.apache.commons.compress.archivers.ArchiveOutputStream;
import org.apache.commons.compress.archivers.tar.TarArchiveEntry;
import org.apache.commons.compress.archivers.tar.TarArchiveInputStream;
import org.apache.commons.compress.archivers.tar.TarArchiveOutputStream;
import org.apache.commons.compress.archivers.zip.Zip64Mode;
import org.apache.commons.compress.archivers.zip.ZipArchiveEntry;
import org.apache.commons.compress.archivers.zip.ZipArchiveInputStream;
import org.apache.commons.compress.archivers.zip.ZipArchiveOutputStream;
import org.apache.commons.compress.compressors.gzip.GzipCompressorInputStream;
import org.apache.commons.compress.compressors.gzip.GzipCompressorOutputStream;
import org.apache.commons.compress.utils.IOUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.*;
import java.nio.file.Files;
import java.util.List;
/**
* 文件解压缩工具
*
* @author Jason He
* @version 1.0.0
* @date 2019-12-28
*/
public class ArchiveUtil {
private static final Logger log = LoggerFactory.getLogger(ArchiveUtil.class);
public static final int ZIP = 1;
public static final int GZ = 2;
public static final int TAR = 3;
public static final int RAR = 4;
private static void compress(String parent, ArchiveOutputStream archiveOutput, File src, int compressType) throws IOException {
if (src.isDirectory()) {
File[] files = src.listFiles();
if (null == files || files.length == 0) {
ArchiveEntry entry = archiveOutput.createArchiveEntry(src, getEntryName(parent, src.getName()));
archiveOutput.putArchiveEntry(entry);
archiveOutput.closeArchiveEntry();
return;
}
for (File file : files) {
compress(getEntryName(parent, src.getName()), archiveOutput, file, compressType);
}
} else {
InputStream ips = null;
try {
ips = new FileInputStream(src);
if (compressType == ZIP) {
ZipArchiveEntry entry = new ZipArchiveEntry(src, getEntryName(parent, src.getName()));
entry.setSize(src.length());
archiveOutput.putArchiveEntry(entry);
} else if (compressType == TAR) {
TarArchiveEntry entry = new TarArchiveEntry(src, getEntryName(parent, src.getName()));
entry.setSize(src.length());
archiveOutput.putArchiveEntry(entry);
} else if (compressType == GZ) {
GzipCompressorOutputStream ops = new GzipCompressorOutputStream(archiveOutput);
TarArchiveEntry entry = new TarArchiveEntry(src, getEntryName(parent, src.getName()));
entry.setSize(src.length());
archiveOutput.putArchiveEntry(entry);
}
IOUtils.copy(ips, archiveOutput);
archiveOutput.closeArchiveEntry();
} catch (IOException e) {
throw e;
} finally {
if (null != ips) {
ips.close();
}
}
}
}
private static void compress(String parent, String fileName, ArchiveOutputStream archiveOutput,
InputStream ips, int compressType) throws IOException {
try {
if (compressType == ZIP) {
ZipArchiveEntry entry = new ZipArchiveEntry(getEntryName(parent, fileName));
archiveOutput.putArchiveEntry(entry);
} else if (compressType == TAR) {
TarArchiveEntry entry = new TarArchiveEntry(getEntryName(parent, fileName));
archiveOutput.putArchiveEntry(entry);
}
IOUtils.copy(ips, archiveOutput);
archiveOutput.closeArchiveEntry();
} catch (IOException e) {
throw e;
} finally {
if (null != ips) {
ips.close();
}
}
}
private static void decompress(ArchiveInputStream archiveInput, File targetDir) throws IOException {
try (ArchiveInputStream i = archiveInput) {
ArchiveEntry entry = null;
while ((entry = i.getNextEntry()) != null) {
if (!i.canReadEntryData(entry)) {
// log something?
continue;
}
File f = new File(targetDir, entry.getName());
if (entry.isDirectory()) {
if (!f.isDirectory() && !f.mkdirs()) {
throw new IOException("failed to create directory " + f);
}
} else {
File parent = f.getParentFile();
if (!parent.isDirectory() && !parent.mkdirs()) {
throw new IOException("failed to create directory " + parent);
}
log.info("decompressing -> {}", f.toPath());
try (OutputStream o = Files.newOutputStream(f.toPath())) {
IOUtils.copy(i, o);
}
}
}
}
}
/**
* 压缩文件
* @param src 源文件
* @param target 目标zip文件
* @throws IOException
*/
public static void zip(File src, File target) throws IOException {
ZipArchiveOutputStream zipOutput = null;
String parent = "";
try {
zipOutput = new ZipArchiveOutputStream(target);
zipOutput.setUseZip64(Zip64Mode.AsNeeded);
compress(parent, zipOutput, src, ZIP);
} catch (IOException e) {
throw e;
} finally {
if (null != zipOutput) {
zipOutput.finish();
zipOutput.close();
}
}
}
/**
* 压缩文件
* @param src 源文件
* @param target 目标zip文件
* @throws IOException
*/
public static void zip(String src, String target) throws IOException {
File srcFile = new File(src);
AssertUtil.exists(srcFile);
File targetFile = new File(target);
zip(srcFile, targetFile);
}
/**
* 将所有文件压缩在同一级目录。
* @param files 文件列表
* @param target 目标zip文件
* @throws IOException
*/
public static void zip(List files, File target) throws IOException {
ZipArchiveOutputStream zipOutput = null;
String parent = "";
try {
zipOutput = new ZipArchiveOutputStream(target);
zipOutput.setUseZip64(Zip64Mode.AsNeeded);
for (File file : files) {
compress(parent, zipOutput, file, ZIP);
}
} catch (IOException e) {
throw e;
} finally {
if (null != zipOutput) {
zipOutput.finish();
zipOutput.close();
}
}
}
/**
* 将流压缩到文件
* @param fileName 流的文件名
* @param ips 流
* @param target 目标zip文件
* @throws IOException
*/
public static void zip(String fileName, InputStream ips, String target) throws IOException {
File targetFile = new File(target);
ZipArchiveOutputStream zipOutput = null;
try {
zipOutput = new ZipArchiveOutputStream(targetFile);
zipOutput.setUseZip64(Zip64Mode.AsNeeded);
compress("", fileName, zipOutput, ips, ZIP);
} catch (IOException e) {
throw e;
} finally {
if (null != zipOutput) {
zipOutput.finish();
zipOutput.close();
}
}
}
public static void unzip(File src, File target) throws IOException {
ZipArchiveInputStream zipInput = null;
try {
zipInput = new ZipArchiveInputStream(new FileInputStream(src));
decompress(zipInput, target);
} catch (IOException e) {
throw e;
} finally {
if (null != zipInput) {
zipInput.close();
}
}
}
/**
* 压缩文件
* @param src 源文件
* @param target 目标tar.gz文件
* @throws IOException
*/
public static void tarGz(File src, File target) throws IOException {
TarArchiveOutputStream tarOutput = null;
String parent = "";
try {
GzipCompressorOutputStream gzipOut = new GzipCompressorOutputStream(new FileOutputStream(target));
tarOutput = new TarArchiveOutputStream(gzipOut);
compress(parent, tarOutput, src, TAR);
} catch (IOException e) {
throw e;
} finally {
if (null != tarOutput) {
tarOutput.finish();
tarOutput.close();
}
}
}
/**
* 压缩文件
* @param src 源文件
* @param target 目标tar.gz文件
* @throws IOException
*/
public static void tarGz(String src, String target) throws IOException {
File srcFile = new File(src);
AssertUtil.exists(srcFile);
File targetFile = new File(target);
tarGz(srcFile, targetFile);
}
/**
* 将所有文件压缩在同一级目录。
* @param files 文件列表
* @param target 目标tar.gz文件
* @throws IOException
*/
public static void tarGz(List files, File target) throws IOException {
TarArchiveOutputStream tarOutput = null;
String parent = "";
try {
GzipCompressorOutputStream gzipOut = new GzipCompressorOutputStream(new FileOutputStream(target));
tarOutput = new TarArchiveOutputStream(gzipOut);
for (File file : files) {
compress(parent, tarOutput, file, TAR);
}
} catch (IOException e) {
throw e;
} finally {
if (null != tarOutput) {
tarOutput.finish();
tarOutput.close();
}
}
}
/**
* 将流压缩到文件
* @param fileName 流的文件名
* @param ips 流
* @param target 目标tar.gz文件
* @throws IOException
*/
public static void tarGz(String fileName, InputStream ips, String target) throws IOException {
TarArchiveOutputStream tarOutput = null;
try {
GzipCompressorOutputStream gzipOut = new GzipCompressorOutputStream(new FileOutputStream(target));
tarOutput = new TarArchiveOutputStream(gzipOut);
compress("", fileName, tarOutput, ips, TAR);
} catch (IOException e) {
throw e;
} finally {
if (null != tarOutput) {
tarOutput.finish();
tarOutput.close();
}
}
}
public static void unTarGz(File src, File target) throws IOException {
TarArchiveInputStream tarInput = null;
try {
GzipCompressorInputStream gzipIn = new GzipCompressorInputStream(new FileInputStream(src));
tarInput = new TarArchiveInputStream(gzipIn);
decompress(tarInput, target);
} catch (IOException e) {
throw e;
} finally {
if (null != tarInput) {
tarInput.close();
}
}
}
public static void gz(File src, File target) throws IOException {
OutputStream ops = new FileOutputStream(target);
try {
gz(src, ops);
} catch (IOException e) {
throw e;
} finally {
ops.close();
}
}
public static void gz(File src, OutputStream ops) throws IOException {
if (!src.isFile()) {
throw new IllegalArgumentException("source file must not be a directory.");
}
InputStream ips = new BufferedInputStream(new FileInputStream(src));
try {
gz(ips, ops);
} catch (IOException e) {
throw e;
} finally {
ips.close();
}
}
public static void gz(InputStream src, File target) throws IOException {
OutputStream ops = new BufferedOutputStream(new FileOutputStream(target));
try {
gz(src, ops);
} catch (IOException e) {
throw e;
} finally {
ops.close();
}
}
public static byte[] gz(byte[] src) throws IOException {
InputStream ips = new ByteArrayInputStream(src);
ByteArrayOutputStream ops = new ByteArrayOutputStream();
try {
gz(ips, ops);
} catch (IOException e) {
throw e;
} finally {
ips.close();
ops.close();
return ops.toByteArray();
}
}
public static void gz(InputStream src, OutputStream ops) throws IOException {
OutputStream cops = null;
try {
cops = new GzipCompressorOutputStream(ops);
IOUtils.copy(src, cops);
} catch (IOException e) {
throw e;
} finally {
if (null != cops) {
cops.close();
}
}
}
public static void unGz(File src, File target) throws IOException {
InputStream ips = new FileInputStream(src);
OutputStream ops = new FileOutputStream(target);
try {
unGz(ips, ops);
} catch (IOException e) {
throw e;
} finally {
if (null != ips) {
ips.close();
}
if (null != ops) {
ops.close();
}
}
}
public static byte[] unGz(byte[] src) throws IOException {
InputStream ips = new ByteArrayInputStream(src);
ByteArrayOutputStream ops = new ByteArrayOutputStream();
try {
unGz(ips, ops);
} catch (IOException e) {
throw e;
} finally {
ips.close();
ops.close();
return ops.toByteArray();
}
}
public static void unGz(InputStream src, OutputStream ops) throws IOException {
InputStream cips = null;
try {
cips = new GzipCompressorInputStream(src);
IOUtils.copy(cips, ops);
} catch (IOException e) {
throw e;
} finally {
if (null != cips) {
cips.close();
}
}
}
private static String getEntryName(String parent, String fileName) {
return (StringUtil.isNullOrEmpty(parent) ? "" : parent + File.separator) + fileName;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy