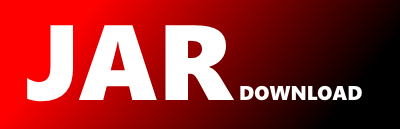
com.github.hetianyi.common.util.CollectionUtil Maven / Gradle / Ivy
package com.github.hetianyi.common.util;
import java.util.*;
/**
* 集合工具类
*
* @author Jason He
* @version 1.0.6
* @since 1.0.0
* @date 2019-12-27
*/
public final class CollectionUtil {
/**
* 不可变的空list
*/
public static final List EMPTY_LIST = Collections.EMPTY_LIST;
/**
* 不可变的空map
*/
public static final Map EMPTY_MAP = Collections.EMPTY_MAP;
/**
* 不可变的空set
*/
public static final Set EMPTY_SET = Collections.EMPTY_SET;
/**
* 判断集合是否为null或者空集合
* @param collection
* @return
*/
public static final boolean isNullOrEmpty(Collection collection) {
return null == collection || collection.isEmpty();
}
/**
* 确保List集合不为null,如果为null,则替换为一个empty的List返回。
* @param data 数据
* @return
*/
public static final List ensureNotNull(List data) {
if (null == data) {
return EMPTY_LIST;
}
return data;
}
/**
* 确保Set集合不为null,如果为null,则替换为一个empty的Set返回。
* @param data 数据
* @return
*/
public static final Set ensureNotNull(Set data) {
if (null == data) {
return EMPTY_SET;
}
return data;
}
/**
* 确保Map不为null,如果为null,则替换为一个empty的Map返回。
* @param data 数据
* @return
*/
public static final Map ensureNotNull(Map data) {
if (null == data) {
return EMPTY_MAP;
}
return data;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy