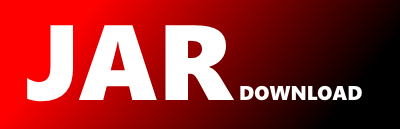
com.github.hetianyi.common.util.InetUtil Maven / Gradle / Ivy
package com.github.hetianyi.common.util;
import com.google.common.collect.Maps;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.core.env.ConfigurableEnvironment;
import org.springframework.core.env.PropertySource;
import org.springframework.core.env.SystemEnvironmentPropertySource;
import java.io.IOException;
import java.net.Inet4Address;
import java.net.InetAddress;
import java.net.NetworkInterface;
import java.util.*;
import java.util.stream.Collectors;
import static org.springframework.core.env.StandardEnvironment.SYSTEM_ENVIRONMENT_PROPERTY_SOURCE_NAME;
/**
* network address util
*
* @author Jason He
* @version 1.0.0
* @since 1.0.2
* @date 2020-01-20
*/
public class InetUtil {
private static final Logger log = LoggerFactory.getLogger(InetUtil.class);
/**
* 查找本机的网络地址
* @return
*/
public static List findNonLoopbackAddress() {
List addresses = new LinkedList<>();
List interfaces = new LinkedList<>();
try {
for (Enumeration nics = NetworkInterface
.getNetworkInterfaces(); nics.hasMoreElements();) {
NetworkInterface ifc = nics.nextElement();
if (ifc.isUp() ) {
interfaces.add(ifc);
}
}
interfaces = interfaces.stream().sorted(Comparator.comparing(NetworkInterface::getIndex))
.collect(Collectors.toList());
interfaces.forEach(ifc -> {
for (Enumeration addrs = ifc
.getInetAddresses(); addrs.hasMoreElements();) {
InetAddress address = addrs.nextElement();
if (address instanceof Inet4Address
&& !address.isLoopbackAddress()) {
addresses.add(address);
}
}
});
}
catch (IOException ex) {
log.error("Cannot get first non-loopback address", ex);
}
if (!CollectionUtil.isNullOrEmpty(addresses)) {
return addresses;
}
return null;
}
/**
* 获取所有网卡和对应的网络地址
* @return
*/
public static Map> findNonLoopbackInterfacesWithAddress() {
Map> ret = new HashMap<>();
try {
for (Enumeration nics = NetworkInterface
.getNetworkInterfaces(); nics.hasMoreElements();) {
NetworkInterface ifc = nics.nextElement();
if (ifc.isUp()) {
List addresses = new LinkedList<>();
for (Enumeration addrs = ifc
.getInetAddresses(); addrs.hasMoreElements();) {
InetAddress address = addrs.nextElement();
if (address instanceof Inet4Address
&& !address.isLoopbackAddress()) {
addresses.add(address);
}
}
ret.put(ifc, addresses);
}
}
return ret;
}
catch (IOException ex) {
log.error("Cannot get first non-loopback address", ex);
}
return null;
}
/**
* 根据网卡过滤IP地址
* @param interfaceNames
* @return
*/
public static List filterByInterfaceNames(List interfaceNames) {
List result = new ArrayList<>();
if (CollectionUtil.isNullOrEmpty(interfaceNames)) {
return null;
}
Map> ni = findNonLoopbackInterfacesWithAddress();
if (ni.isEmpty()) {
return null;
}
interfaceNames.forEach(o -> {
String m = o.toLowerCase();
ni.forEach((k, v) -> {
if (Objects.equals(k.getName().toLowerCase(), m) || Objects.equals(k.getDisplayName().toLowerCase(), m)
|| k.getName().toLowerCase().contains(m) || k.getDisplayName().toLowerCase().contains(m)) {
v.forEach(n -> result.add(n.getHostAddress()));
}
});
});
return result;
}
/**
* 根据IP地址过滤IP地址
* @param addressPatterns
* @return
*/
public static List filterByAddress(List addressPatterns) {
List result = new ArrayList<>();
if (CollectionUtil.isNullOrEmpty(addressPatterns)) {
return null;
}
List nonLoopbackAddress = findNonLoopbackAddress();
if (nonLoopbackAddress.isEmpty()) {
return null;
}
addressPatterns.forEach(o -> {
nonLoopbackAddress.forEach(v -> {
if (Objects.equals(v.getHostAddress(), o) || v.getHostAddress().contains(o)) {
result.add(v.getHostAddress());
}
});
});
return result;
}
/**
* 设置启动时spring cloud注册到注册中心的IP地址。
* Example:
*
* @Order(Ordered.HIGHEST_PRECEDENCE)
* public class SpringCloudInetResolver implements BeanPostProcessor, EnvironmentPostProcessor, Ordered {
*
* @Override
* public void postProcessEnvironment(ConfigurableEnvironment environment, SpringApplication application) {
* InetUtil.processNetworkEnvironment(environment, new InetUtil.NetworkProviderFilter() {
* @Override
* public List<String> provide(String os) {
* return Arrays.asList("wlan", "eth0");
* // return Arrays.asList("192.168.1.");
* }
*
* @Override
* public int filterBy(String os) {
* return InetUtil.INTERFACE_NAME;
* // return InetUtil.IP_ADDRESS;
* }
* });
* }
*
* @Override
* public int getOrder() {
* return ConfigFileApplicationListener.DEFAULT_ORDER - 2;
* }
* }
*
*
*
* 然后创建文件:resources/META-INF/spring.factories
*
* 文件内容为:
*
*
* org.springframework.boot.env.EnvironmentPostProcessor=\
* com.xxx.SpringCloudInetResolver
*
* @param environment
* @param networkProviderFilter
*/
public static void processNetworkEnvironment(ConfigurableEnvironment environment, NetworkProviderFilter networkProviderFilter) {
AssertUtil.notNull(networkProviderFilter, "networkProviderFilter must not be null");
String proKey = "spring.cloud.inetutils.preferredNetworks";
Map preferredNetworks = Maps.newHashMap();
String os = System.getProperty("os.name").toLowerCase();
preferredNetworks.put(proKey,
networkProviderFilter.filterBy(os) == INTERFACE_NAME ?
InetUtil.filterByInterfaceNames(networkProviderFilter.provide(os)) :
InetUtil.filterByAddress(networkProviderFilter.provide(os))
);
PropertySource
© 2015 - 2024 Weber Informatics LLC | Privacy Policy