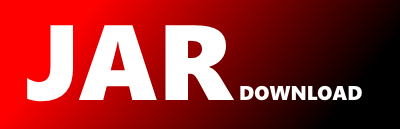
com.github.hetianyi.common.util.NumberUtil Maven / Gradle / Ivy
package com.github.hetianyi.common.util;
import java.math.BigDecimal;
import java.math.RoundingMode;
import java.text.DecimalFormat;
import java.util.HashMap;
import java.util.Map;
/**
* 数字操作工具类
*
* @author Jason He
* @version 1.0.9
* @date 2020-02-21
* @since 1.0.9
*/
public class NumberUtil {
private static final Map format = new HashMap(10);
private static final Map formatWithZero = new HashMap(10);
private static final int MODE_ZERO = 0;
private static final int MODE_ONLY = 1;
private static final String getPattern(int mode, int scale) {
Map cache = mode == MODE_ZERO ? formatWithZero : format;
String pattern = cache.get(scale);
if (null == pattern) {
synchronized (cache) {
pattern = (mode == MODE_ZERO ? "0." : "#.");
for (int i = 0; i < scale; i++) {
pattern += (mode == MODE_ZERO ? "0" : "#");
}
cache.put(scale, pattern);
}
}
return pattern;
}
/**
* 将数字四舍五入格式化为字符串,并保留指定小数位数,
* 不足的位数用0补齐。
*
* @param n 数字
* @param scale 小数位数
*/
public static String formatWithZero(Number n, int scale) {
return new BigDecimal(n.doubleValue()).setScale(scale, RoundingMode.HALF_UP).toString();
}
/**
* 将数字四舍五入格式化为字符串,并保留指定小数位数,
* 末位为0的舍去。
*
* @param n 数字
* @param scale 小数位数
*/
public static String format(Number n, int scale) {
if (scale == 0) {
return new DecimalFormat("0").format(n);
}
BigDecimal bg = new BigDecimal(n.doubleValue()).setScale(scale, RoundingMode.HALF_UP);
DecimalFormat df = new DecimalFormat(getPattern(MODE_ONLY, scale));
return df.format(bg.doubleValue());
}
/**
* 累加数字
* @param n 数字列表
* @return 数字累加和
*/
public static Number add(Number... n) {
if (null == n || n.length == 0) {
return 0;
}
double t = 0;
for (Number i : n) {
t += i.doubleValue();
}
return t;
}
/**
* 累加数字
* @param n 数字列表
* @return 数字累加和
*/
public static Number multiply(Number... n) {
if (null == n || n.length == 0) {
return 0;
}
double t = 0;
for (Number i : n) {
t *= i.doubleValue();
}
return t;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy