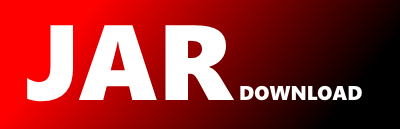
com.hn.doc.xyj.parse.ParseUtils Maven / Gradle / Ivy
package com.hn.doc.xyj.parse;
import cn.hutool.core.io.FileUtil;
import cn.hutool.log.Log;
import cn.hutool.log.LogFactory;
import com.github.javaparser.JavaParser;
import com.github.javaparser.ast.CompilationUnit;
import com.github.javaparser.ast.Modifier;
import com.github.javaparser.ast.NodeList;
import com.github.javaparser.ast.body.FieldDeclaration;
import com.github.javaparser.ast.body.MethodDeclaration;
import com.github.javaparser.ast.body.VariableDeclarator;
import com.github.javaparser.javadoc.Javadoc;
import com.github.javaparser.javadoc.JavadocBlockTag;
import com.hn.doc.xyj.Xyj;
import com.hn.doc.xyj.domain.DocData;
import com.hn.utils.AssertUtils;
import lombok.Data;
import java.io.FileNotFoundException;
import java.net.URL;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.function.Consumer;
/**
* 描述: 实体类解析
*
* @author fei
* 2020-01-10 11:37
*/
@Data
public class ParseUtils {
private static final Log log = LogFactory.get();
public static DomainParse domain(Class clazz) {
String className = clazz.getSimpleName();
log.info("className:{}", className);
URL classUrl = clazz.getResource("");
AssertUtils.notNull(classUrl, "class URL not found");
String javaSrcPath = String.valueOf(classUrl).replace("target/classes/",
"src/main/java/") + clazz.getSimpleName() + ".java";
log.info("class文件路径:{}", javaSrcPath);
CompilationUnit compilationUnit = null;
try {
compilationUnit = JavaParser.parse(FileUtil.file(javaSrcPath));
} catch (FileNotFoundException e) {
log.error("JavaParser解析失败");
e.printStackTrace();
}
log.info("JavaParser解析成功");
DomainParse domainParse = new DomainParse();
domainParse.setClassName(className);
domainParse.setJavaSrcPath(javaSrcPath);
List fieldList = new ArrayList<>();
compilationUnit.getClassByName(className)
.ifPresent(c -> {
c.findAll(FieldDeclaration.class).stream().filter(
fd -> fd.getModifiers().contains(Modifier.PRIVATE)
&& !fd.getModifiers().contains(Modifier.STATIC)
&& !fd.getModifiers().contains(Modifier.FINAL))
.forEach(fd -> {
DomainParse.Field field = domainParse.createField();
NodeList variables = fd.getVariables();
variables.forEach(v -> {
String name = v.getNameAsString();
String type = v.getTypeAsString();
field.setName(name);
field.setType(type);
});
fd.getComment().ifPresent(comment -> {
String content = comment.getContent();
content = cleanCommentContent(content);
field.setComment(content);
});
fieldList.add(field);
});
});
domainParse.setFieldList(fieldList);
return domainParse;
}
public static List method(Class clazz) {
String className = clazz.getSimpleName();
log.info("className:{}", className);
URL classUrl = clazz.getResource("");
AssertUtils.notNull(classUrl, "class URL not found");
String javaSrcPath = String.valueOf(classUrl).replace("target/classes/",
"src/main/java/") + clazz.getSimpleName() + ".java";
log.info("class文件路径:{}", javaSrcPath);
CompilationUnit compilationUnit = null;
try {
compilationUnit = JavaParser.parse(FileUtil.file(javaSrcPath));
} catch (FileNotFoundException e) {
log.error("JavaParser解析失败");
e.printStackTrace();
}
log.info("JavaParser解析成功");
List methodParses = new ArrayList<>();
compilationUnit.getClassByName(className)
.ifPresent(c -> {
c.findAll(MethodDeclaration.class).stream().filter(
md -> md.getModifiers().contains(Modifier.PUBLIC)
&& !md.getModifiers().contains(Modifier.STATIC)
&& !md.getModifiers().contains(Modifier.FINAL))
.forEach(md -> {
MethodParse methodParse = new MethodParse();
methodParse.setName(md.getName().asString());
md.getJavadoc().ifPresent(javaDoc -> {
methodParse.setDescription(javaDoc.getDescription().toText());
javaDoc.getBlockTags().stream().filter(
javadocBlockTag -> "param".equals(javadocBlockTag.getTagName()))
.forEach(jbt -> {
String name = jbt.getName().get();
String content = jbt.getContent().toText();
methodParse.addParam(name, content);
});
methodParses.add(methodParse);
});
});
});
return methodParses;
}
public static MethodParse method(Class clazz, String methodName) {
List methodParses = method(clazz);
for (MethodParse methodParse : methodParses) {
if (methodName.equals(methodParse.getName())) {
return methodParse;
}
}
return null;
}
public static void main(String[] args) throws Exception {
MethodParse method = method(Xyj.class,"req");
List method1 = method(Xyj.class);
System.out.println();
}
/**
* 移除一些字符比如 [* \n]
*
* @param content 内容
* @return 移除后内容
*/
public static String cleanCommentContent(String content) {
return content.replace("*", "").replace("\n", "").trim();
}
/**
* means a model class type
*/
private static final String TYPE_MODEL = "unkown";
/**
* unify the type show in docs
*
* @param className className
* @return String
*/
public static String unifyType(String className) {
String[] cPaths = className.split("\\.");
String rawType = cPaths[cPaths.length - 1];
if ("byte".equalsIgnoreCase(rawType)) {
return "byte";
} else if ("short".equalsIgnoreCase(rawType)) {
return "short";
} else if ("int".equalsIgnoreCase(rawType)
|| "Integer".equalsIgnoreCase(rawType)
|| "BigInteger".equalsIgnoreCase(rawType)) {
return "int";
} else if ("long".equalsIgnoreCase(rawType)) {
return "long";
} else if ("float".equalsIgnoreCase(rawType)) {
return "float";
} else if ("double".equalsIgnoreCase(rawType)
|| "BigDecimal".equalsIgnoreCase(rawType)) {
return "double";
} else if ("boolean".equalsIgnoreCase(rawType)) {
return "boolean";
} else if ("char".equalsIgnoreCase(rawType)
|| "Character".equalsIgnoreCase(rawType)) {
return "char";
} else if ("String".equalsIgnoreCase(rawType)) {
return "string";
} else if ("date".equalsIgnoreCase(rawType)
|| "ZonedDateTime".equalsIgnoreCase(rawType)) {
return "date";
} else if ("file".equalsIgnoreCase(rawType)) {
return "file";
} else {
return TYPE_MODEL;
}
}
/**
* is implements from Collection or not
*
* @param className className
* @return boolean
*/
public static boolean isCollectionType(String className) {
String[] cPaths = className.split("\\.");
String genericType = cPaths[cPaths.length - 1];
//fix List
for (String cPath : cPaths) {
if (cPath.contains("<")) {
genericType = cPath;
}
}
int genericLeftIndex = genericType.indexOf("<");
String rawType = genericLeftIndex != -1 ? genericType.substring(0, genericLeftIndex) : genericType;
String collectionClassName = "java.util." + rawType;
try {
Class collectionClass = Class.forName(collectionClassName);
return Collection.class.isAssignableFrom(collectionClass);
} catch (ClassNotFoundException e) {
return false;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy