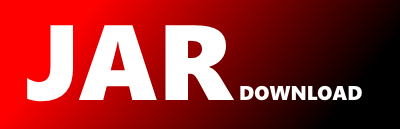
com.hn.map.qq.LocationUtil Maven / Gradle / Ivy
package com.hn.map.qq;
import cn.hutool.core.util.StrUtil;
import cn.hutool.http.HttpUtil;
import cn.hutool.json.JSONObject;
import cn.hutool.json.JSONUtil;
import cn.hutool.log.Log;
import cn.hutool.log.LogFactory;
import com.hn.config.HnConfigUtils;
import com.hn.config.exception.ConfigException;
import com.hn.map.MapException;
import com.hn.map.qq.domain.AddressComponent;
import com.hn.map.qq.domain.Location;
import com.hn.utils.AssertUtils;
import java.util.HashMap;
import java.util.Map;
/**
* 描述:
* 位置工具类
*
* @author fei
* 2019-12-30 18:31
*/
public class LocationUtil {
private static final Log log = LogFactory.get();
/**
* 开发密钥
*/
private static String key;
/**
* 请求地址
*/
private static final String REQ_URL = "https://apis.map.qq.com/ws/geocoder/v1/";
static {
// 初始化key
key = getKey("");
}
/**
* 读取对应场景的key
* @param scene 场景
*/
public static void scene(String scene){
// 这样会存在线程安全问题
key = getKey(scene);
}
/**
* 逆地址解析(坐标位置描述)
*
* @param lat 纬度
* @param lng 经度
* @return addressComponent {@link AddressComponent}
*/
public static AddressComponent locationToAddress(double lat, double lng) {
Map param = new HashMap<>();
param.put("key", key);
// 是否返回周边POI列表: 1.返回;0不返回(默认)
param.put("get_poi", 1);
// param.put("poi_options","address_format=short");
// 返回格式:支持JSON/JSONP,默认JSON
param.put("output", "JSON");
param.put("location", lat + "," + lng);
String resultStr = HttpUtil.get(REQ_URL, param);
log.debug("[腾讯地图]逆地址解析(坐标位置描述) 返回结果:{}", resultStr);
JSONObject resultObject = JSONUtil.parseObj(resultStr);
if (resultObject.getInt("status") == 0) {
JSONObject result = resultObject.getJSONObject("result");
return JSONUtil.toBean(result.getStr("address_component"),AddressComponent.class);
}
throw new MapException("[腾讯地图]逆地址解析失败:" + resultObject.getStr("message"));
}
/**
* 地址解析(地址转坐标)
*
* @param address 地址 (注:地址中请包含城市名称,否则会影响解析效果)
* @return 坐标信息 {@link Location}
*/
public static Location addressToLocation(String address) {
Map param = new HashMap<>();
param.put("key", key);
// 返回格式:支持JSON/JSONP,默认JSON
param.put("output", "JSON");
param.put("address", address);
String resultStr = HttpUtil.get(REQ_URL, param);
log.debug("[腾讯地图]地址解析(地址转坐标) 返回结果:{}", resultStr);
JSONObject resultObject = JSONUtil.parseObj(resultStr);
if (resultObject.getInt("status") == 0) {
JSONObject result = resultObject.getJSONObject("result");
JSONObject location = result.getJSONObject("location");
return new Location(location.getDouble("lat"), location.getDouble("lng"));
}
throw new MapException("【腾讯地图】地址解析(地址转坐标)失败:" + resultObject.getStr("message"));
}
/**
* 配置前缀名
*/
private static final String CONFIG_KEY = "map.qq";
private static String getKey(String scene){
if(StrUtil.isNotBlank(scene)){
scene = "-".concat(scene);
}
return AssertUtils.notNull(HnConfigUtils.getConfig(CONFIG_KEY.concat(scene).concat(".key")),
ConfigException.exception("腾讯地图key未配置"));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy