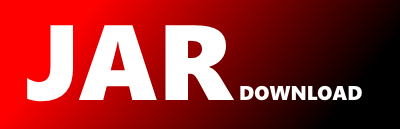
com.hn.robot.dingtalk.DingChatRobot Maven / Gradle / Ivy
package com.hn.robot.dingtalk;
import cn.hutool.core.util.StrUtil;
import cn.hutool.http.HttpUtil;
import cn.hutool.log.Log;
import cn.hutool.log.LogFactory;
import com.hn.config.HnConfigUtils;
import com.hn.config.exception.ConfigException;
import com.hn.robot.dingtalk.domain.Text;
import com.hn.robot.dingtalk.domain.TextMsg;
import com.hn.robot.exception.ChatRobotException;
import com.hn.utils.AssertUtils;
import org.apache.commons.codec.binary.Base64;
import javax.crypto.Mac;
import javax.crypto.spec.SecretKeySpec;
import java.net.URLEncoder;
/**
* 钉钉推送机器人,来源【群设置 =》 智能群助手 =》 添加机器人】
* 安全设置分三种
* 1、自定义关键词(只要内容里面包含关键词就可以发送)
* 2、加签 调用{setSecretKey(String)}方法
* 3、IP地址 (段)
*
* @author fei
*/
public class DingChatRobot {
private static final Log log = LogFactory.get();
private String URL = "https://oapi.dingtalk.com/robot/send?access_token={accessToken}";
/**
* 访问令牌
*/
private String accessToken;
/**
* 密钥
*/
private String secretKey;
public static DingChatRobot create() {
return new DingChatRobot();
}
public static DingChatRobot create(String accessToken, String secretKey) {
return new DingChatRobot(accessToken, secretKey);
}
public static DingChatRobot create(String accessToken) {
return new DingChatRobot(accessToken, null);
}
private DingChatRobot(String accessToken, String secretKey) {
this.accessToken = accessToken;
this.secretKey = secretKey;
setUrl(this.accessToken, this.secretKey);
}
private DingChatRobot() {
getParam();
setUrl(this.accessToken, this.secretKey);
}
/**
* accessToken 来源 群设置 =》 智能群助手 =》 添加机器人 =》 选择自定义 =》 安全设置(推荐加签或ip)
* =》 复制 webhook(https://oapi.dingtalk.com/robot/send?access_token=a8929628776118498168edf12293ab144e09ad92028950a63fa404c74731143f)
* 将access_token的值 赋值到这里
* 加签
*
* @param accessToken 机器人访问令牌
* @param ecretKey 密钥
* @return String
*/
private void setUrl(String accessToken, String secretKey) {
URL = URL.replace("{accessToken}", accessToken);
if (StrUtil.isNotBlank(secretKey)) {
Long timestamp = System.currentTimeMillis();
String stringToSign = timestamp + "\n" + secretKey;
try {
Mac mac = Mac.getInstance("HmacSHA256");
mac.init(new SecretKeySpec(secretKey.getBytes("UTF-8"), "HmacSHA256"));
byte[] signData = mac.doFinal(stringToSign.getBytes("UTF-8"));
String sign = URLEncoder.encode(new String(Base64.encodeBase64(signData)), "UTF-8");
URL = URL + "×tamp=" + timestamp + "&sign=" + sign;
} catch (Exception e) {
log.error("钉钉机器人加签失败:secretKey={}", secretKey);
throw new ChatRobotException("钉钉机器人加签失败:", e);
}
}
}
/**
* 发送普通文本
*
* @param msgContent 消息内容
*/
public void send(String msgContent) {
TextMsg textMsg = new TextMsg();
Text text = textMsg.text();
text.setContent(msgContent);
send(textMsg);
}
/**
* 发送指定类型消息内容
*
* @param textMsg {@link TextMsg}
*/
public void send(TextMsg textMsg) {
String result = HttpUtil.post(URL, textMsg.toJsonStr());
log.info("钉钉机器人推送返回结果:{}", result);
}
/**
* 配置前缀名
*/
private static final String CONFIG_KEY = "robot.ding";
/**
* 从数据库获取钉钉机器人配置
*/
private void getParam() {
String accessToken = AssertUtils.notNull(HnConfigUtils.getConfig(CONFIG_KEY + ".accessToken"),
ConfigException.exception("钉钉机器人accessToken未配置"));
String secretKey = HnConfigUtils.getConfig(CONFIG_KEY + ".secretKey");
if (StrUtil.isNotBlank(secretKey)) {
this.secretKey = secretKey;
}
this.accessToken = accessToken;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy