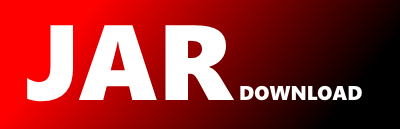
com.hn.robot.dingtalk.domain.Markdown Maven / Gradle / Ivy
package com.hn.robot.dingtalk.domain;
/**
* 描述: markdown类型
* 标题
* # 一级标题
* ## 二级标题
* ### 三级标题
* #### 四级标题
* ##### 五级标题
* ###### 六级标题
*
* 引用
*
* 文字加粗、斜体
* **bold**
* *italic*
*
* 链接
* [this is a link](http://name.com)
*
* 图片
* 
*
* 无序列表
* - item1
* - item2
*
* 有序列表
* 1. item1
* 2. item2
*
* @author fei
*/
public class Markdown {
/**
* 标题
*/
private String title;
/**
* 文本内容
*/
private StringBuilder text=new StringBuilder();
public Markdown(String title) {
this.title = title;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public StringBuilder getText() {
return text;
}
public void setText(StringBuilder text) {
this.text = text;
}
/**
* 标题
* # 一级标题
* ## 二级标题
* ### 三级标题
* #### 四级标题
* ##### 五级标题
* ###### 六级标题
* @param level 标题级别
* @param title 标题名称
* @return Markdown
*/
public Markdown title(int level,String title){
if(level==0){
level=1;
}
for (int i = 0; i < level; i++) {
text.append("#");
}
text.append(" ");
text.append(title);
return this;
}
/**
* 引用
* @param content 内容
* @return Markdown
*/
public Markdown quote(String content){
text.append("> ").append(content);
return this;
}
/**
* 文字加粗
* @param content 内容
* @return Markdown
*/
public Markdown bold(String content){
text.append("**").append(content).append("**");
return this;
}
/**
* 斜体
* @param content 内容
* @return Markdown
*/
public Markdown italic(String content){
text.append("*").append(content).append("*");
return this;
}
/**
* 链接
* [this is a link](http://name.com)
* @param content 链接名称
* @param link 链接地址
* @return Markdown
*/
public Markdown link(String content,String link){
text.append("[").append(content).append("](").append(link).append(")");
return this;
}
/**
* 图片
* 
* @param imgUrl 图片链接
* @return Markdown
*/
public Markdown image(String imgUrl){
text.append(".append(imgUrl).append(")");
return this;
}
/**
* 无序列表
* - item1
* - item2
* @param content 内容
* @return Markdown
*/
public Markdown item(String content){
text.append("-").append(" ").append(content);
return this;
}
/**
* 换行
* @return Markdown
*/
public Markdown ln(){
text.append("\n");
return this;
}
private int i = 0;
/**
* 有序列表
* 1. item1
* 2. item2
* @param content 内容
* @return Markdown
*/
public Markdown itemNum(String content){
i++;
text.append(i).append(".").append(" ").append(content);
return this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy