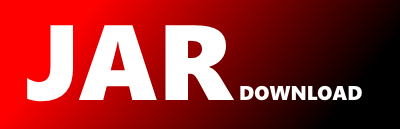
com.hn.robot.tuling.TuLingMsg Maven / Gradle / Ivy
package com.hn.robot.tuling;
import lombok.Data;
import java.util.HashMap;
import java.util.Map;
/**
* 图灵机器人 请求消息实体
*/
@Data
public class TuLingMsg {
/**
* 输入类型:0-文本(默认)、1-图片、2-音频
*/
private Integer reqType;
/**
* 输入信息
*/
private Map perception;
/**
* 用户参数
*/
private TuLingUserInfo userInfo;
public TuLingMsg() {
this.userInfo = new TuLingUserInfo();
this.perception = new HashMap<>(4);
}
public void setReqType(ReqType reqType) {
this.reqType = reqType.type;
}
/**
* 文本信息
* @param text 文本信息
*/
public void setText(String text) {
Map textMap = new HashMap<>();
textMap.put("text",text);
perception.put("inputText",textMap);
}
/**
* 图片信息
* @param url 图片信息
*/
public void setImage(String url) {
Map imageMap = new HashMap<>();
imageMap.put("url",url);
perception.put("inputImage",imageMap);
}
/**
* 音频信息
* @param url 音频信息
*/
public void setMedia(String url) {
Map mediaMap = new HashMap<>();
mediaMap.put("url",url);
perception.put("inputMedia",mediaMap);
}
/**
* 客户端属性
* @param location 地理位置信息
*/
public void setLocation(Location location) {
Map selfInfo = new HashMap<>();
selfInfo.put("location",location);
perception.put("selfInfo",selfInfo);
}
/**
* 机器人标识
* @param apiKey 机器人标识
*/
public void setApiKey(String apiKey) {
userInfo.apiKey = apiKey;
}
/**
* 长度小于等于32位 用户唯一标识
* @param userId 用户唯一标识
*/
public void setUserId(String userId) {
userInfo.userId = userId;
}
/**
* 长度小于等于64位 群聊唯一标识
* @param groupId 群聊唯一标识
*/
public void setGroupId(String groupId) {
userInfo.groupId = groupId;
}
/**
* 长度小于等于64位 群内用户昵称
* @param userIdName 群内用户昵称
*/
public void setUserIdName(String userIdName) {
userInfo.userIdName = userIdName;
}
@Data
class TuLingUserInfo{
/**
* 32位 机器人标识
*/
private String apiKey;
/**
* 长度小于等于32位 用户唯一标识
*/
private String userId;
/**
* 长度小于等于64位 群聊唯一标识
*/
private String groupId;
/**
* 长度小于等于64位 群内用户昵称
*/
private String userIdName;
}
@Data
public static class Location{
/**
* 所在城市
*/
private String city;
/**
* 省份
*/
private String province;
/**
* 街道
*/
private String street;
}
/**
* 输入类型
*/
public enum ReqType{
/**
* 文本(默认)
*/
TEXT(0),
/**
* 图片
*/
IMAGE(1),
/**
* 音频
*/
MEDIA(2);
private int type;
ReqType(int type) {
this.type = type;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy