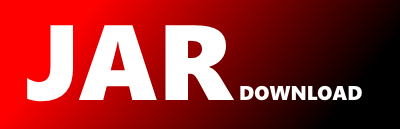
com.hn.upload.QiNiuUpload Maven / Gradle / Ivy
package com.hn.upload;
import cn.hutool.core.exceptions.UtilException;
import cn.hutool.core.io.FileUtil;
import cn.hutool.core.util.ReflectUtil;
import cn.hutool.core.util.StrUtil;
import cn.hutool.log.Log;
import cn.hutool.log.LogFactory;
import com.google.gson.Gson;
import com.hn.config.HnConfigUtils;
import com.hn.config.exception.ConfigException;
import com.hn.utils.AssertUtils;
import com.qiniu.common.QiniuException;
import com.qiniu.http.Response;
import com.qiniu.storage.Configuration;
import com.qiniu.storage.Region;
import com.qiniu.storage.UploadManager;
import com.qiniu.storage.model.DefaultPutRet;
import com.qiniu.util.Auth;
import com.qiniu.util.IOUtils;
import lombok.Data;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
/**
* 描述: 七牛云存储
* api: https://developer.qiniu.com/kodo/api/1731/api-overview
* java sdk: https://developer.qiniu.com/kodo/sdk/1239/java
*
* @author fei
* 2019-12-20 09:06
*/
public class QiNiuUpload extends AbstractFileUpload {
private static final Log log = LogFactory.get();
private Param param;
public static Param createParam() {
return new Param();
}
public QiNiuUpload(Param param) {
checkParam(param);
this.param = param;
}
public QiNiuUpload(String scene) {
Param param = getRequestParam(scene);
checkParam(param);
this.param = param;
}
/**
* 获取七牛云地址
*
* @return fileUrl
*/
@Override
public String getFileBaseUrl() {
return param.fileUrl;
}
/**
* 获取文件临时下载地址
* @param key 文件key(路径)
* @param expireTime 过期时间 以分为单位
* @return 下载地址
*/
@Override
public String getTempDownUrl(String key, Integer expireTime) {
String domainOfBucket = param.fileUrl;
String encodedFileName = null;
try {
encodedFileName = URLEncoder.encode(key, "utf-8").replace("+", "%20");
} catch (UnsupportedEncodingException e) {
throw new UploadException("七牛云存储URLEncoder编码异常");
}
String publicUrl = String.format("%s/%s", domainOfBucket, encodedFileName);
Auth auth = Auth.create(param.accessKey, param.secretKey);
long expireInSeconds = expireTime * 60L;
return auth.privateDownloadUrl(publicUrl, expireInSeconds);
}
/**
* 生成上传授权uptoken
*
* @return 上传授权uptoken
*/
@Override
public String getToken() {
Auth auth = Auth.create(param.accessKey, param.secretKey);
return auth.uploadToken(param.bucket);
}
/**
* 文件上传
*
* @param bytes 字节数组
* @param key 默认不指定key的情况下,以文件内容的hash值作为文件名
* @return key
*/
private String uploadFile(byte[] bytes, String key) {
if (bytes.length <= 0) {
AssertUtils.error(UploadException.exception("七牛云获取getBytes失败"));
}
//构造一个带指定 Region 对象的配置类
Configuration cfg = param.configuration();
UploadManager uploadManager = new UploadManager(cfg);
// 生成上传凭证,然后准备上传
String upToken = getToken();
try {
Response response = uploadManager.put(bytes, key, upToken);
//解析上传成功的结果
DefaultPutRet ret = new Gson().fromJson(response.bodyString(), DefaultPutRet.class);
log.info("七牛云上传成功 文件路径:{} hash:{}", ret.key, ret.hash);
} catch (QiniuException ex) {
Response r = ex.response;
log.info("七牛云上传失败 文件路径:{} hash:{}", r.toString());
}
// 返回的图像路径
return key;
}
@Override
public String uploadFile(File file, String key) {
AssertUtils.notNull(file, UploadException.exception("七牛云上传文件不能为空"));
byte[] bytes = new byte[0];
try {
bytes = IOUtils.toByteArray(FileUtil.getInputStream(file));
} catch (IOException e) {
e.printStackTrace();
}
return uploadFile(bytes, key);
}
@Override
public String uploadFile(InputStream is, String key) {
AssertUtils.notNull(is, UploadException.exception("七牛云上传文件不能为空"));
byte[] bytes = new byte[0];
try {
bytes = IOUtils.toByteArray(is);
} catch (IOException e) {
e.printStackTrace();
}
return uploadFile(bytes, key);
}
private void checkParam(Param param) {
AssertUtils.notNull(param, "param is null");
AssertUtils.notNull(param.accessKey,
ConfigException.exception("七牛云存储accessKey未配置"));
AssertUtils.notNull(param.secretKey,
ConfigException.exception("七牛云存储secretKey未配置"));
AssertUtils.notNull(param.bucket,
ConfigException.exception("七牛云存储bucketName未配置"));
AssertUtils.notNull(param.fileUrl,
ConfigException.exception("七牛云存储fileUrl未配置"));
AssertUtils.notNull(param.region,
ConfigException.exception("七牛云存储区域名称region未配置"));
}
/**
* 配置前缀名
*/
private static final String CONFIG_KEY = "upload.qiNiu";
/**
* 获取数据配置
*
* @return Param
*/
private Param getRequestParam(String scene) {
String configKey = CONFIG_KEY;
if (StrUtil.isNotBlank(scene)) {
configKey = configKey.concat("-").concat(scene);
}
String accessKey = HnConfigUtils.getConfig(configKey.concat(".accessKey"));
String secretKey = HnConfigUtils.getConfig(configKey.concat(".secretKey"));
String bucket = HnConfigUtils.getConfig(configKey.concat(".bucket"));
String fileUrl = HnConfigUtils.getConfig(configKey.concat(".fileUrl"));
String region = HnConfigUtils.getConfig(configKey.concat(".region"));
Param param = new Param();
param.setAccessKey(accessKey);
param.setSecretKey(secretKey);
param.setBucket(bucket);
param.setFileUrl(fileUrl);
param.setRegion(region);
return param;
}
@Data
public static class Param {
/**
* 【七牛云存储】accessKey
*/
private String accessKey;
/**
* 【七牛云存储】secretKey
*/
private String secretKey;
/**
* 【七牛云存储】bucket
*/
private String bucket;
/**
* 【七牛云存储】fileUrl
*/
private String fileUrl;
/**
* 【七牛云存储】区域名称region
*/
private String region;
public Configuration configuration() {
Region r = new Region();
try {
r = ReflectUtil.invoke(r, this.region);
} catch (UtilException e) {
throw new UploadException("七牛云查询不到此区域 区域参考:华东=huadong 华北=huabei" +
" 华南=huanan 北美=beimei 新加坡=xinjiapo ");
}
return new Configuration(r);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy