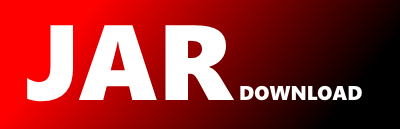
com.hn.utils.RongYingCall Maven / Gradle / Ivy
package com.hn.utils;
import cn.hutool.core.codec.Base64;
import cn.hutool.core.date.DatePattern;
import cn.hutool.core.date.DateTime;
import cn.hutool.core.date.DateUnit;
import cn.hutool.core.date.DateUtil;
import cn.hutool.crypto.SecureUtil;
import cn.hutool.crypto.digest.MD5;
import cn.hutool.http.HttpException;
import cn.hutool.http.HttpRequest;
import cn.hutool.http.HttpResponse;
import cn.hutool.json.JSONException;
import cn.hutool.json.JSONObject;
import cn.hutool.json.JSONUtil;
import java.io.UnsupportedEncodingException;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
/**
* 融营云通信
*
*
* 官网
* 文档地址
*
*/
public class RongYingCall {
/**
* 授权令牌
*/
private static final String accountSid = "465f5a35e6664e31ae9e956f1bd5e6db" ;
/**
* 应用Id
*/
static String appId = "73ca599bae17450daaea74544003b54c" ;
/**
* 应用Token
*/
static String appToken = "bb48216306444a96b12bc0b5ae1bb272" ;
/**
* 创建坐席
*/
private static final String createUrl = "https://wdapi.yuntongxin.vip/20190105/rest/CreateSeatAccount/v1?sig=" ;
/**
* 小号号码
*/
private static final String bindUrl = "https://wdapi.yuntongxin.vip/20190105/axb/bind/callEvent/v1?sig=" ;
/**
* 解绑小号号码
*/
private static final String unbindUrl = "https://wdapi.yuntongxin.vip/20190105/axb/unbind/number/Event/v1?sig=" ;
/**
* 点击外呼请求地址
*/
private static final String callUrl = "https://wdapi.yuntongxin.vip/20190105/rest/click/call/event/v1?sig=" ;
/**
* 修改绑定号码请求地址
*/
private static final String upUrl = "https://wdapi.yuntongxin.vip/20190105/rest/ChangeBindNumber/v1?sig=" ;
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
// TODO code application logic here
String bind_number = "18715083549" ; // 坐席绑定号码
String calleer = "15755137199" ; // 主叫号码
String callee = "15556508251" ; // 被叫号码
String virtual_num = "17138380010" ; // 小号号码
String new_number = "18911111111" ; // 新绑定电话号码
// createSeat(bind_number); // 创建坐席
// xiaohao(calleer,callee);
// seatBindNumber(bind_number, virtual_num); //坐席绑定小号
// seat_call_phone(virtual_num, callee); // 坐席请求外呼
// up_bind_number(bind_number, new_number); // 修改坐席绑定号码
// seat_unbind_number("833daeec-31e2-4bdc-9d21-48ab3c2f816e", virtual_num); //坐席解绑小号
seatBindNumber(calleer,callee,virtual_num);
// seatUnbindNumber("e403fb11-ea76-4d07-b8b6-b8f6345a790c",virtual_num);
}
/**
* 小号号码绑定
* {
* "Flag": 1,
* "Msg": "+8617138380010",
* "SubscriptionId": "e403fb11-ea76-4d07-b8b6-b8f6345a790c"
* }
*
* @param caller 主叫号码
* @param callee 被叫号码
* @param virtualNum 小号号码
*/
public static void seatBindNumber(String caller, String callee, String virtualNum) {
Map infos = new HashMap<>();
infos.put("AccountSid", accountSid);
infos.put("Appid", appId);
// 主叫号码
infos.put("Caller", caller);
// 被叫号码
infos.put("Callee", callee);
// 虚拟号码
infos.put("VirtualNum", virtualNum);
// 允许主被叫呼叫方向 默认为 0
// 0:主被叫可以相互呼叫; 1:只能主叫呼叫被叫; 2:只能被叫呼叫主叫;
infos.put("Direct", "1");
// 号码绑定关系有效期
// 绑定关系到期后将自动解除此绑定关系,如果不携带或参数为 0 则默认一天;
// 1. 单位为分钟;例如:1(分钟)
// 2. 默认时间为一天;
// 3. 最大绑定关系 30 天;
// infos.put("Duration", "1");
// 单次通话最大时长
// 允许单次通话进行最大时长,通话时间从接通被叫开始计算;
// infos.put("MaxDuration", "60");
post(bindUrl, infos);
}
/**
* 小号号码解绑
*
* @param subscriptionId 需解绑对应小号关系标识
* @param virtualNum 坐席绑定的小号号码
*/
public static void seatUnbindNumber(String subscriptionId, String virtualNum) {
Map infos = new HashMap<>();
infos.put("VirtualNum", virtualNum);
infos.put("SubscriptionId", subscriptionId);
post(unbindUrl, infos);
}
/**
* 创建坐席
*
* @param bindNumber 坐席绑定的号码
*/
public static void createSeat(String bindNumber) {
Map infos = new HashMap<>();
infos.put("appId", appId);
infos.put("bindNumber", bindNumber);
post(createUrl, infos);
}
/**
* 修改坐席绑定号码
*
* @param oldNumber oldNumber
* @param newNumber newNumber
*/
public static void upBindNumber(String oldNumber, String newNumber) {
Map infos = new HashMap<>();
infos.put("Appid", appId);
infos.put("oldNumber", oldNumber);
infos.put("newNumber", newNumber);
post(upUrl, infos);
}
/**
* 坐席请求外呼
*
* @param virtualNum 坐席绑定的小号号码
* @param toPhone 被叫号码
*/
public static void seatCallPhone(String virtualNum, String toPhone) {
Map infos = new HashMap<>();
infos.put("Appid", appId);
infos.put("AccountSid", accountSid);
infos.put("Caller", virtualNum);
infos.put("Callee", toPhone);
infos.put("IsDisplayCalleeNbr", "false");
post(callUrl, infos);
}
private static String post(String url, Map param) {
String timeStr = DateUtil.format(DateUtil.date(), DatePattern.PURE_DATETIME_FORMAT);
String sign = SecureUtil.md5(accountSid + ":" + appId + ":" + timeStr).toUpperCase();
String auth = Base64.encode(appId + ":" + appToken + ":" + timeStr);
System.out.println("请求参数"+JSONUtil.toJsonStr(param));
HttpResponse response = HttpRequest.post(url + sign)
.header("Authorization", auth)
.body(JSONUtil.toJsonStr(param)).execute();
String result = response.body();
JSONObject resultObj = null;
try {
resultObj = JSONUtil.parseObj(result);
} catch (JSONException jsonException) {
throw new HttpException("融营通信失败:" + result);
}
Integer flag = resultObj.getInt("Flag");
System.out.println(result);
if (flag != 1) {
throw new HttpException("融营通信失败:" + resultObj.getStr("Msg"));
}
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy