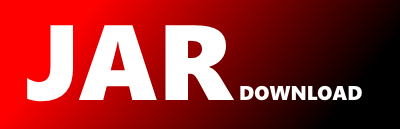
com.hn.utils.dingtalk.DingDeptUtils Maven / Gradle / Ivy
package com.hn.utils.dingtalk;
import cn.hutool.json.JSONUtil;
import com.dingtalk.api.DefaultDingTalkClient;
import com.dingtalk.api.DingTalkClient;
import com.dingtalk.api.request.OapiDepartmentCreateRequest;
import com.dingtalk.api.request.OapiDepartmentDeleteRequest;
import com.dingtalk.api.request.OapiDepartmentListRequest;
import com.dingtalk.api.request.OapiDepartmentUpdateRequest;
import com.dingtalk.api.response.*;
import com.hn.utils.dingtalk.constant.DingConstant;
import com.hn.utils.dingtalk.exception.DingTalkException;
import com.taobao.api.ApiException;
import lombok.extern.slf4j.Slf4j;
import java.util.List;
/**
* 描述:
* 部门管理
* @author fei
* 2019-06-28 14:32
*/
@Slf4j
public class DingDeptUtils {
/**
* 获取部门列表
*
* access_token String 是 调用接口凭证
* lang String 否 通讯录语言(默认zh_CN,未来会支持en_US)
* fetch_child Boolean 否 是否递归部门的全部子部门,ISV微应用固定传递false
* id String 是 父部门id(如果不传,默认部门为根部门,根部门ID为1)
* @param id 部门id
* @return {@link OapiDepartmentListResponse.Department}
*/
public static List list(String id){
DingTalkClient client = new DefaultDingTalkClient("https://oapi.dingtalk.com/department/list");
OapiDepartmentListRequest request = new OapiDepartmentListRequest();
request.setId(id);
request.setHttpMethod("GET");
try {
OapiDepartmentListResponse response = client.execute(request, AccessTokenUtil.getToken());
if (DingConstant.SUCCESS_CODE.equals(response.getErrcode()) ) {
log.debug("获取部门列表 success,{}",JSONUtil.toJsonStr(response.getDepartment()));
return response.getDepartment();
}
throw new DingTalkException("获取部门列表异常 fail:,"+response.getErrmsg());
} catch (ApiException e) {
throw new DingTalkException("获取部门列表异常 fail:,"+e.getErrMsg());
}
}
/**
* 根据部门名称获取部门
* @param deptName 部门名称
* @return 部门信息 {@link OapiDepartmentListResponse.Department}
*/
public static OapiDepartmentListResponse.Department getDeptByName(String deptName){
List list = list(null);
for (OapiDepartmentListResponse.Department dept : list) {
if(deptName.equals(dept.getName())){
return dept;
}
}
return null;
}
/**
* 创建部门
* @param parentId 父部门id
* @param deptName 部门名称
* @return 部门ID
*/
public static long createDept(String parentId,String deptName) {
DingTalkClient client = new DefaultDingTalkClient("https://oapi.dingtalk.com/department/create");
OapiDepartmentCreateRequest request = new OapiDepartmentCreateRequest();
// parentId为1,就是根部门
request.setParentid(parentId);
// 是否创建一个关联此部门的企业群,默认为false
request.setCreateDeptGroup(false);
request.setOrder("100");
request.setName(deptName);
try {
OapiDepartmentCreateResponse response = client.execute(request, AccessTokenUtil.getToken());
if (DingConstant.SUCCESS_CODE.equals(response.getErrcode()) ) {
log.debug("创建部门 success,{}",response.getId());
return response.getId();
}
throw new DingTalkException("创建部门失败 fail:,"+response.getErrmsg());
} catch (ApiException e) {
throw new DingTalkException("创建部门失败 fail:,"+e.getErrMsg());
}
}
/**
* 创建根部门下 的部门
* @param deptName 部门名称
* @return 部门id
*/
public static long createDept(String deptName) {
return createDept("1",deptName);
}
/**
* 更新部门
* @param deptId 部门id
* @param deptName 部门名称
*/
public static void updateDept(String deptId,String deptName) {
DingTalkClient client = new DefaultDingTalkClient("https://oapi.dingtalk.com/department/update");
OapiDepartmentUpdateRequest request = new OapiDepartmentUpdateRequest();
request.setId(Long.valueOf(deptId));
request.setName(deptName);
try {
OapiDepartmentUpdateResponse response = client.execute(request, AccessTokenUtil.getToken());
if (DingConstant.SUCCESS_CODE.equals(response.getErrcode()) ) {
log.debug("更新部门 success,{}",response.getId());
}
throw new DingTalkException("更新部门失败 fail:,"+response.getErrmsg());
} catch (ApiException e) {
throw new DingTalkException("更新部门失败 fail:,"+e.getErrMsg());
}
}
/**
* 删除部门
* (注:不能删除根部门;当部门里有员工,或者部门的子部门里有员工,也不能删除)
* @param deptId 部门id
*/
public static void delDept(String deptId) {
DingTalkClient client = new DefaultDingTalkClient("https://oapi.dingtalk.com/department/delete");
OapiDepartmentDeleteRequest request = new OapiDepartmentDeleteRequest();
request.setId(deptId);
request.setHttpMethod("GET");
try {
OapiDepartmentDeleteResponse response = client.execute(request, AccessTokenUtil.getToken());
if (DingConstant.SUCCESS_CODE.equals(response.getErrcode()) ) {
log.debug("删除部门 success,{}",deptId);
}
throw new DingTalkException("删除部门失败 fail:,"+response.getErrmsg());
} catch (ApiException e) {
throw new DingTalkException("删除部门失败 fail:,"+e.getErrMsg());
}
}
public static void main(String[] args) {
/* Long deptId = getDeptByName("办公室");
System.out.println(deptId);
List userlists = DingUserUtil.listUserByDeptId(deptId);*/
List list = list("1");
for (OapiDepartmentListResponse.Department department : list) {
System.out.println(department.getName());
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy