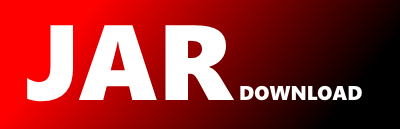
com.hn.utils.dingtalk.DingUserUtil Maven / Gradle / Ivy
package com.hn.utils.dingtalk;
import cn.hutool.json.JSONUtil;
import com.dingtalk.api.DefaultDingTalkClient;
import com.dingtalk.api.DingTalkClient;
import com.dingtalk.api.request.*;
import com.dingtalk.api.response.*;
import com.hn.utils.dingtalk.constant.DingConstant;
import com.hn.utils.dingtalk.exception.DingTalkException;
import com.taobao.api.ApiException;
import lombok.extern.slf4j.Slf4j;
import java.util.ArrayList;
import java.util.List;
/**
* 描述:
* 钉钉用户
*
* @author fei
* 2019-06-28 14:23
*/
@Slf4j
public class DingUserUtil {
/**
* 获取用户信息通过 requestAuthCode
* @param requestAuthCode 授权code
* @return {
* "userid": "****", 员工在当前企业内的唯一标识,也称staffId
* "sys_level": 1, 级别,1:主管理员,2:子管理员,100:老板,0:其他(如普通员工)
* "errmsg": "ok", 对返回码的文本描述内容
* "is_sys": true, 是否是管理员,true:是,false:不是
* "errcode": 0 返回码
* }
*/
public static OapiUserGetuserinfoResponse getUserInfoByCode(String requestAuthCode){
DingTalkClient client = new DefaultDingTalkClient("https://oapi.dingtalk.com/user/getuserinfo");
OapiUserGetuserinfoRequest request = new OapiUserGetuserinfoRequest();
request.setCode(requestAuthCode);
request.setHttpMethod("GET");
OapiUserGetuserinfoResponse response = null;
try {
response = client.execute(request, AccessTokenUtil.getToken());
if (DingConstant.SUCCESS_CODE.equals(response.getErrcode())) {
log.debug("获取用户详情 success,{}", JSONUtil.toJsonStr(response));
return response;
}
throw new DingTalkException("获取用户详情异常," + response.getErrmsg());
} catch (ApiException e) {
throw new DingTalkException("获取用户详情异常," + e.getErrMsg());
}
}
/**
* 获取用户详情
* @param userId 用户id
* @return {@link OapiUserGetResponse}
*/
public static OapiUserGetResponse get(String userId) {
DingTalkClient client = new DefaultDingTalkClient("https://oapi.dingtalk.com/user/get");
OapiUserGetRequest request = new OapiUserGetRequest();
request.setUserid(userId);
request.setHttpMethod("GET");
try {
OapiUserGetResponse response = client.execute(request, AccessTokenUtil.getToken());
if (DingConstant.SUCCESS_CODE.equals(response.getErrcode())) {
log.debug("获取用户详情 success,{}", JSONUtil.toJsonStr(response));
return response;
}
throw new DingTalkException("获取用户详情异常," + response.getErrmsg());
} catch (ApiException e) {
throw new DingTalkException("获取用户详情异常," + e.getErrMsg());
}
}
/**
* 获取部门用户userId列表
*
* @param deptId 部门id
* @return list userid列表
*/
public static List listUserIdsByDeptId(String deptId) {
DingTalkClient client = new DefaultDingTalkClient("https://oapi.dingtalk.com/user/getDeptMember");
OapiUserGetDeptMemberRequest req = new OapiUserGetDeptMemberRequest();
req.setDeptId(deptId);
req.setHttpMethod("GET");
OapiUserGetDeptMemberResponse response = null;
try {
response = client.execute(req, AccessTokenUtil.getToken());
} catch (ApiException e) {
throw new DingTalkException("获取部门用户userId列表异常," + e.getErrMsg());
}
if (DingConstant.SUCCESS_CODE.equals(response.getErrcode())) {
log.debug("获取部门用户userId列表 success,{}", response.getUserIds());
return response.getUserIds();
}
throw new DingTalkException("获取部门用户userId列表异常," + response.getErrmsg());
}
/**
* 获取部门用户
* @param deptId 部门id
* @return {@link OapiUserSimplelistResponse.Userlist}
*/
public static List listUserNameByDeptId(Long deptId) {
DingTalkClient client = new DefaultDingTalkClient("https://oapi.dingtalk.com/user/simplelist");
OapiUserSimplelistRequest request = new OapiUserSimplelistRequest();
request.setDepartmentId(deptId);
request.setOffset(0L);
request.setSize(10L);
request.setHttpMethod("GET");
try {
OapiUserSimplelistResponse response = client.execute(request, AccessTokenUtil.getToken());
if (DingConstant.SUCCESS_CODE.equals(response.getErrcode())) {
log.debug("获取部门用户 success,{}", response.getUserlist());
return response.getUserlist();
}
throw new DingTalkException("获取部门用户异常," + response.getErrmsg());
} catch (ApiException e) {
throw new DingTalkException("获取部门用户异常," + e.getErrMsg());
}
}
/**
* 获取部门用户详情
* @param deptId 部门id
* @return {@link OapiUserListbypageResponse.Userlist}
*/
public static List listUserByDeptId(Long deptId) {
DingTalkClient client = new DefaultDingTalkClient("https://oapi.dingtalk.com/user/listbypage");
OapiUserListbypageRequest request = new OapiUserListbypageRequest();
request.setDepartmentId(deptId);
request.setOffset(0L);
request.setSize(100L);
request.setOrder("entry_desc");
request.setHttpMethod("GET");
try {
OapiUserListbypageResponse response = client.execute(request, AccessTokenUtil.getToken());
if (DingConstant.SUCCESS_CODE.equals(response.getErrcode())) {
log.debug("获取部门用户详情 success,{}", response.getUserlist());
return response.getUserlist();
}
throw new DingTalkException("获取部门用户详情异常," + response.getErrmsg());
} catch (ApiException e) {
throw new DingTalkException("获取部门用户详情异常," + e.getErrMsg());
}
}
/**
* 根据手机号获取部门用户详情
* @param deptId 部门id
* @param phone 手机号
* @return {@link OapiUserListbypageResponse.Userlist}
*/
public static OapiUserListbypageResponse.Userlist getUserByDeptIdAndPhone(Long deptId,String phone) {
List userlists = listUserByDeptId(deptId);
for (OapiUserListbypageResponse.Userlist user : userlists) {
if(phone.equals(user.getMobile())){
return user;
}
}
return null;
}
/**
* 获取角色列表
*
* @param offset 当前页 默认0
* @param size 每页显示记录数
* @return {@link OapiRoleListResponse.PageVo}
*/
public static OapiRoleListResponse.PageVo listRole(Long offset, Long size) {
DingTalkClient client = new DefaultDingTalkClient("https://oapi.dingtalk.com/topapi/role/list");
OapiRoleListRequest request = new OapiRoleListRequest();
request.setOffset(offset);
request.setSize(size);
try {
OapiRoleListResponse response = client.execute(request, AccessTokenUtil.getToken());
if (DingConstant.SUCCESS_CODE.equals(response.getErrcode())) {
log.debug("获取角色列表 success,{}", JSONUtil.toJsonStr(response.getResult()));
return response.getResult();
}
throw new DingTalkException("获取角色列表异常," + response.getErrmsg());
} catch (ApiException e) {
throw new DingTalkException("获取角色列表异常," + e.getErrMsg());
}
}
/**
* 获取角色下的员工列表
* 请求方式:POST(HTTPS)
* 请求地址:https://oapi.dingtalk.com/topapi/role/simplelist?access_token=ACCESS_TOKEN
*
* @param roleId 权限id
* @param offset 当前页 默认0
* @param size 每页显示记录数
* @return {@link OapiRoleSimplelistResponse.PageVo}
*/
public static OapiRoleSimplelistResponse.PageVo listUserByRoleId(Long roleId, Long offset, Long size) {
DingTalkClient client = new DefaultDingTalkClient("https://oapi.dingtalk.com/topapi/role/simplelist");
OapiRoleSimplelistRequest request = new OapiRoleSimplelistRequest();
request.setRoleId(roleId);
request.setOffset(offset);
request.setSize(size);
try {
OapiRoleSimplelistResponse response = client.execute(request, AccessTokenUtil.getToken());
if (DingConstant.SUCCESS_CODE.equals(response.getErrcode())) {
log.debug("获取角色下的员工列表 success,{}", response.getResult());
return response.getResult();
}
throw new DingTalkException("获取角色下的员工列表异常," + response.getErrmsg());
} catch (ApiException e) {
throw new DingTalkException("获取角色下的员工列表异常," + e.getErrMsg());
}
}
/**
* 创建用户
* @param name 姓名
* @param mobile 手机号
* @param dingDeptId 部门id
* @return 用户id
*/
public static String createUser(String name,String mobile,Long dingDeptId){
DingTalkClient client = new DefaultDingTalkClient("https://oapi.dingtalk.com/user/create");
OapiUserCreateRequest request = new OapiUserCreateRequest();
request.setMobile(mobile);
request.setName(name);
//所属部门
List departments = new ArrayList();
departments.add(dingDeptId);
request.setDepartment(JSONUtil.toJsonStr(departments));
try {
OapiUserCreateResponse response = client.execute(request, AccessTokenUtil.getToken());
if (DingConstant.SUCCESS_CODE.equals(response.getErrcode())) {
log.debug("创建用户 success,{}", response.getUserid());
return response.getUserid();
}
throw new DingTalkException("创建用户失败," + response.getErrmsg());
} catch (ApiException e){
throw new DingTalkException("创建用户失败," + e.getErrMsg());
}
}
/**
* 更新用户
* @param userId 用户id
* @param name 姓名
* @param mobile 手机号
* @param deptId 部门id
*/
public static void updateUser(String userId,String name,String mobile,Long deptId){
DingTalkClient client = new DefaultDingTalkClient("https://oapi.dingtalk.com/user/update");
OapiUserUpdateRequest request = new OapiUserUpdateRequest();
request.setUserid(userId);
request.setName(name);
request.setMobile(mobile);
//所属部门
List departments = new ArrayList();
departments.add(deptId);
request.setDepartment(departments);
try {
OapiUserUpdateResponse response = client.execute(request, AccessTokenUtil.getToken());
if (DingConstant.SUCCESS_CODE.equals(response.getErrcode())) {
log.debug("更新用户 success,{}", response.getErrcode());
}
throw new DingTalkException("更新用户失败," + response.getErrmsg());
} catch (ApiException e){
throw new DingTalkException("更新用户失败," + e.getErrMsg());
}
}
/**
* 删除用户
* @param userId 用户ID
*/
public static void delUser(String userId){
DingTalkClient client = new DefaultDingTalkClient("https://oapi.dingtalk.com/user/delete");
OapiUserDeleteRequest request = new OapiUserDeleteRequest();
request.setUserid(userId);
request.setHttpMethod("GET");
try {
OapiUserDeleteResponse response = client.execute(request, AccessTokenUtil.getToken());
if (DingConstant.SUCCESS_CODE.equals(response.getErrcode())) {
log.debug("删除用户 success,{}", response.getErrcode());
}
throw new DingTalkException("删除用户失败," + response.getErrmsg());
} catch (ApiException e) {
throw new DingTalkException("删除用户失败," + e.getErrMsg());
}
}
public static void main(String[] args) {
/* List list = listUserIdsByDeptId("117628808");
for (String userId : list){
OapiUserGetResponse userGetResponse = get(userId);
System.out.println(userGetResponse.getName());
}*/
// 获取权限列表
/* OapiRoleListResponse.PageVo pageVo = listRole(0L, 10L);
List list1 = pageVo.getList();
for (OapiRoleListResponse.OpenRoleGroup openRoleGroup : list1) {
String name = openRoleGroup.getName();
}
OapiUserGetResponse userGetResponse = get("0502101558117240531");*/
/* List list = listUserIdsByDeptId("1");
System.out.println(list);*/
OapiUserGetResponse userGetResponse = get("0502101558117240531");
}
/**
* 根据手机号获取用户信息
* @param phone 手机号
* @return 用户信息 {@link OapiUserGetResponse}
*/
public static OapiUserGetResponse getDingUserByPhone(String phone) {
DingTalkClient client = new DefaultDingTalkClient("https://oapi.dingtalk.com/user/get_by_mobile");
OapiUserGetByMobileRequest request = new OapiUserGetByMobileRequest();
request.setMobile(phone);
try {
OapiUserGetByMobileResponse response = client.execute(request, AccessTokenUtil.getToken());
if (DingConstant.SUCCESS_CODE.equals(response.getErrcode())) {
log.debug("根据手机号获取userid success,{}", response.getErrcode());
String userid = response.getUserid();
OapiUserGetResponse userGetResponse = get(userid);
if (DingConstant.SUCCESS_CODE.equals(userGetResponse.getErrcode())) {
log.debug("根据手机号获取用户信息 success,{}", response.getErrcode());
return userGetResponse;
}
return null;
}
log.debug("根据手机号获取userid 失败,{}", response.getErrmsg());
return null;
} catch (ApiException e) {
log.debug("根据手机号获取userid 失败,{}", e.getErrMsg());
return null;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy