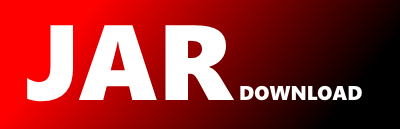
com.hn.utils.generate.GenDomain Maven / Gradle / Ivy
package com.hn.utils.generate;
import com.hn.utils.AssertUtils;
import com.hn.utils.table.TableUtils;
import com.hn.utils.table.TableInfo;
import freemarker.template.TemplateExceptionHandler;
import javax.sql.DataSource;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
/**
* 描述:
* 代码生成器,根据数据表名称生成对应的Model简化开发。
*
* @author fei
* 2020-01-17 10:29
*/
public class GenDomain {
/**
* 项目在硬盘上的基础路径
*/
private static final String PROJECT_PATH = System.getProperty("user.dir");
/**
* 模板位置
*/
private static final String TEMPLATE_FILE_PATH = PROJECT_PATH + "/src/main/resources/template";
/**
* 生成路径
*/
private String targetPath;
/**
* 作者
*/
private String author;
/**
* {@link TableUtils}
*/
private TableUtils tableUtils;
/**
* 连接数据库
*
* @param jdbcUrl jdbcUrl
* @param userName 用户名
* @param password 密码
* @return GenDomain
*/
public static GenDomain db(String jdbcUrl, String userName, String password) {
TableUtils tableUtil = new TableUtils(jdbcUrl, userName, password);
return new GenDomain(tableUtil);
}
/**
* 连接数据库
*
* @param dataSource 数据源
* @return GenDomain
*/
public static GenDomain db(DataSource dataSource) {
TableUtils tableUtil = new TableUtils(dataSource);
return new GenDomain(tableUtil);
}
/**
* 作者
*
* @param author 作者
* @return GenDomain
*/
public GenDomain author(String author) {
this.author = author;
return this;
}
/**
* 生成路径
*
* @param targetPath 生成路径
* @return GenDomain
*/
public GenDomain targetPath(String targetPath) {
this.targetPath = targetPath;
return this;
}
private GenDomain(TableUtils tableUtils) {
this.tableUtils = tableUtils;
}
/**
* 通过数据表名称,和自定义的 Model 名称生成代码
* 如输入表名称 "t_user_detail" 和自定义的 Model 名称 "User" 将生成 User、UserMapper、UserService ...
*
* @param tableName 数据表名称
*/
public void genCode(String tableName) {
AssertUtils.notNull(tableUtils, "请先连接数据库");
AssertUtils.notNull(tableName, "数据表tableName不能为空");
AssertUtils.notNull(targetPath, "生成目录targetPath不能为空");
genPojo(tableName);
}
private void genPojo(String tableName) {
try {
freemarker.template.Configuration cfg = getConfiguration();
Map data = new HashMap<>(3);
data.put("author", author);
String modelNameUpperCamel = TableUtils.nameConvertUpperCamel(tableName);
data.put("modelNameUpperCamel", modelNameUpperCamel);
data.put("modelNameLowerCamel", TableUtils.nameConvertLowerCamel(tableName));
TableInfo table = tableUtils.getTable(tableName);
data.put("tableComment", table.getTableComment());
data.put("columns", table.getColumns());
data.put("primaryKey", table.getPrimaryKey());
File file = new File(targetPath + modelNameUpperCamel + ".java");
if (!file.getParentFile().exists()) {
file.getParentFile().mkdirs();
}
cfg.getTemplate("domain.ftl").process(data, new FileWriter(file));
System.out.println(modelNameUpperCamel + ".java 生成成功");
} catch (Exception e) {
throw new RuntimeException("生成domain失败", e);
}
}
/**
* Freemarker提供了3种加载模板目录的方法,使用Configuration类加载模板
* 第一种示例(适合加载Jar包内的资源):
*
* Configuration cfg = new Configuration();
* cfg.setClassForTemplateLoading(FreemarkerUtil.class, "/template");
* cfg.getTemplate("Base.ftl");
*
* 第二种示例(适合加载文件路径):
* Configuration cfg = new Configuration();
* cfg.setDirectoryForTemplateLoading(new File("/home/user/template"));
* cfg.getTemplate("Base.ftl");
*
* 第三种示例(基于WebRoot下的):
* Configuration cfg = new Configuration();
* 指定FreeMarker模板文件的位置
*
* try {
* cfg.setServletContextForTemplateLoading(getServletContext(), "/ftl");就是 /WebRoot/ftl目录
* cfg.getTemplate("Base.ftl");
* } catch (Exception e) {
* e.printStackTrace();
* }
*
* @return Configuration {@link freemarker.template.Configuration}
* @throws IOException IOException
*/
private freemarker.template.Configuration getConfiguration() throws IOException {
freemarker.template.Configuration cfg = new freemarker.template.Configuration(freemarker.template.Configuration.VERSION_2_3_23);
// ClassLoader cd = this.getClass().getClassLoader();
// File file = FileUtil.file(cd.getResource("template"));
// cfg.setDirectoryForTemplateLoading(file);
cfg.setClassForTemplateLoading(GenDomain.class, "/template");
cfg.setDefaultEncoding("UTF-8");
cfg.setTemplateExceptionHandler(TemplateExceptionHandler.IGNORE_HANDLER);
return cfg;
}
}