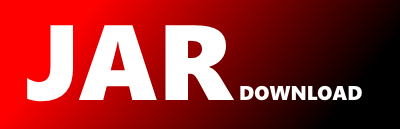
com.hn.asr.qcloud.voice.VoiceUtil Maven / Gradle / Ivy
package com.hn.asr.qcloud.voice;
import cn.hutool.core.codec.Base64;
import com.hn.config.HnConfigUtils;
import com.hn.config.exception.ConfigException;
import com.hn.utils.AssertUtils;
import org.apache.http.HttpResponse;
import org.apache.http.HttpStatus;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.ByteArrayEntity;
import org.apache.http.impl.client.DefaultHttpClient;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import javax.crypto.Mac;
import javax.crypto.spec.SecretKeySpec;
import java.io.*;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
import java.text.MessageFormat;
import java.util.HashMap;
import java.util.Map;
import java.util.Random;
/**
* 腾讯云语音识别/工具类
*
* @author: yang
* 2020/01/06 14:43
*/
public class VoiceUtil {
private static Logger logger = LoggerFactory.getLogger(VoiceUtil.class);
/**
* 配置前缀名
*/
private static final String CONFIG_KEY = "tencent.voiceDistinguish";
/**
* 静态代码块进行读取数据库配置
*/
static {
String appId = AssertUtils.notNull(HnConfigUtils.getConfig(CONFIG_KEY.concat(".appId")),
ConfigException.exception("appId未配置"));
String secretId = AssertUtils.notNull(HnConfigUtils.getConfig(CONFIG_KEY.concat(".secretId")),
ConfigException.exception("secretId未配置"));
String secretKey = AssertUtils.notNull(HnConfigUtils.getConfig(CONFIG_KEY.concat(".secretKey")),
ConfigException.exception("secretKey未配置"));
String callbackUrl = AssertUtils.notNull(HnConfigUtils.getConfig(CONFIG_KEY.concat(".callbackUrl")),
ConfigException.exception("callbackUrl未配置"));
APP_ID = appId;
SECTET_Id = secretId;
SECRET_KEY = secretKey;
CALLBACK_URL = callbackUrl;
}
//加密类型
private static final String HMAC_SHA1_ALGORITHM = "HmacSHA1";
//appId
private static final String APP_ID;
//sectetId
private static final String SECTET_Id;
//secretKey
private static final String SECRET_KEY;
//回调地址
private static final String CALLBACK_URL;
/**
* 语音识别 /录音文件
*
* @param voicePath 文件地址
* @return 发送结果
*/
public String sendVoiceFile(String voicePath) {
Map map = new HashMap<>();
map.put("projectid", "0");
map.put("secretid", SECTET_Id);
map.put("sub_service_type", "0");
map.put("engine_model_type", "8k_0");
map.put("res_text_format", "0");
map.put("res_type", "0");
map.put("callback_url", CALLBACK_URL);
map.put("channel_num", "1");
map.put("source_type", "1");
map.put("timestamp", String.valueOf(System.currentTimeMillis() / 1000));
map.put("expired", String.valueOf(System.currentTimeMillis() / 1000 + 1000));
Random random = new Random();
map.put("nonce", random.nextInt(100000) + "");
//网址
String host = "aai.qcloud.com";
//拼接请求链接
String requestUrl = "https://" + getRequestUrl(map);
//加密字段
String addPwdWord = "POST" + getRequestUrl(map);
//HmacSha1 算法进行加密处理
String authorization = getHMACSHA1(addPwdWord);
logger.info("请求链接:{}" + requestUrl);
String contentType = "application/octet-stream";
//返回结果
String result = null;
File file = new File(voicePath);
int contentLength = (int) file.length();
try {
FileInputStream fileInputStream = new FileInputStream(file);
BufferedInputStream bis = new BufferedInputStream(fileInputStream);
byte[] bytes = new byte[contentLength];
bis.read(bytes);
//发送post请求
result = sendPostVoice(requestUrl, host, authorization, contentType, bytes);
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return result;
}
/**
* 语音识别回调(方法模板/接收腾讯那边回调)
* 回调调用请求是 post
* @param code
* @param message
* @param requestId
* @param appId
* @param projectId
* @param audioUrl
* @param text 语音识别的内容
* @param audioTime
* @throws Exception
*/
/*@PostMapping("/callback")
public void callBack(@RequestParam(name = "code", required = false) String code,
@RequestParam(name = "message", required = false) String message,
@RequestParam(name = "requestId", required = false) String requestId,
@RequestParam(name = "appid", required = false) String appId,
@RequestParam(name = "projectid", required = false) String projectId,
@RequestParam(name = "audioUrl", required = false) String audioUrl,
@RequestParam(name = "text", required = false) String text,
@RequestParam(name = "audioTime", required = false) String audioTime) throws Exception {
if ("0".equals(code) && "成功".equals(message)) {
logger.info(code);
logger.info(message);
logger.info(requestId);
logger.info(appId);
logger.info(projectId);
logger.info(audioUrl);
logger.info("text:" + text);
//回调识别内容
logger.info("text utg-8:" + URLDecoder.decode(text, "UTF-8"));
logger.info(audioTime);
}
}*/
/**
* 发送post请求方法
*
* @param requestUrl
* @param host
* @param authorization
* @param contentType
* @param bytes
* @return 返回请求结果
*/
private String sendPostVoice(String requestUrl, String host, String authorization, String contentType, byte[] bytes) {
HttpClient httpClient = new DefaultHttpClient();
HttpPost httpPost = new HttpPost(requestUrl);
String result = "";
httpPost.setHeader("Host", host);
httpPost.setHeader("Authorization", authorization);
httpPost.setHeader("Content-Type", contentType);
httpPost.setEntity(new ByteArrayEntity(bytes));
try {
HttpResponse httpResponse = httpClient.execute(httpPost);
//请求服务器成功,做相应处理
if (httpResponse.getStatusLine().getStatusCode() == HttpStatus.SC_OK) {
InputStream is = httpResponse.getEntity().getContent();
BufferedReader br = new BufferedReader(new InputStreamReader(is, "UTF-8"));
StringBuffer sb = new StringBuffer();
String line = "";
while ((line = br.readLine()) != null) {
sb.append(line);
}
br.close();
is.close();
result = sb.toString();
logger.info("服务器请求成功结果:{}", result);
} else {
logger.info("服务器请求失败!");
}
} catch (IOException e) {
e.printStackTrace();
}
return result;
}
/**
* 加密字段方法
* HmacSha1 算法进行加密处理
*
* @param str
* @return 加密结果
*/
private String getHMACSHA1(String str) {
try {
SecretKeySpec signInKey = new SecretKeySpec(SECRET_KEY.getBytes(), HMAC_SHA1_ALGORITHM);
Mac mac = Mac.getInstance(HMAC_SHA1_ALGORITHM);
mac.init(signInKey);
byte[] rawHmac = mac.doFinal(str.getBytes());
return Base64.encode(rawHmac);
} catch (NoSuchAlgorithmException e) {
System.err.println(e.getMessage());
} catch (InvalidKeyException e) {
System.err.println(e.getMessage());
}
return null;
}
/**
* 封装请求url方法
*
* @param map
* @return 封装url
*/
private String getRequestUrl(Map map) {
StringBuilder sb = new StringBuilder("aai.qcloud.com/asr/v1/");
sb.append(APP_ID);
// key 要按照字典排序
sb.append(MessageFormat.format("?callback_url={0}", map.get("callback_url")));
sb.append(MessageFormat.format("&channel_num={0}", map.get("channel_num")));
sb.append(MessageFormat.format("&engine_model_type={0}", map.get("engine_model_type")));
sb.append(MessageFormat.format("&expired={0}", map.get("expired")));
sb.append(MessageFormat.format("&nonce={0}", map.get("nonce")));
sb.append(MessageFormat.format("&projectid={0}", map.get("projectid")));
sb.append(MessageFormat.format("&res_text_format={0}", map.get("res_text_format")));
sb.append(MessageFormat.format("&res_type={0}", map.get("res_type")));
sb.append(MessageFormat.format("&secretid={0}", map.get("secretid")));
sb.append(MessageFormat.format("&source_type={0}", map.get("source_type")));
sb.append(MessageFormat.format("&sub_service_type={0}", map.get("sub_service_type")));
sb.append(MessageFormat.format("×tamp={0}", map.get("timestamp")));
return sb.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy