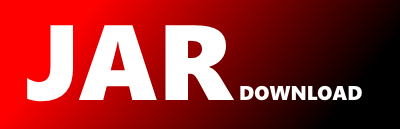
com.hn.opencv.PerspectiveTransform Maven / Gradle / Ivy
package com.hn.opencv;
import org.opencv.core.*;
import org.opencv.imgcodecs.Imgcodecs;
import org.opencv.imgproc.Imgproc;
import org.opencv.utils.Converters;
import java.util.List;
/**
* opencv 透视变化
* @author fei
*/
public class PerspectiveTransform {
public static void main(String[] args) {
// 原图地址
String srcImgPath = args[0];
// 四个点的坐标
String x1 = args[1];
String x2 = args[2];
String x3 = args[3];
String x4 = args[4];
// 变化后图片地址
String destImgPath = args[5];
System.loadLibrary(Core.NATIVE_LIBRARY_NAME);
Mat src = Imgcodecs.imread(srcImgPath);
// 读取图像到矩阵中,取灰度图像
if (src.empty()) {
return;
}
try {
Mat dst = new Mat();
List listSrcs = java.util.Arrays.asList(getPoint(x1), getPoint(x2),
getPoint(x3), getPoint(x4));
Mat srcPoints = Converters.vector_Point_to_Mat(listSrcs, CvType.CV_32F);
List listDsts = java.util.Arrays.asList(new Point(0, 0), new Point(1011, 0),
new Point(1011, 638), new Point(0, 638));
Mat dstPoints = Converters.vector_Point_to_Mat(listDsts, CvType.CV_32F);
Mat perspectiveMmat = Imgproc.getPerspectiveTransform(srcPoints, dstPoints);
Size size = new Size(new Point(1011, 638));
Imgproc.warpPerspective(src, dst, perspectiveMmat, size, Imgproc.INTER_LINEAR);
Mat gray = gray(dst);
Imgcodecs.imwrite(destImgPath, gray);
} catch (Exception e) {
e.printStackTrace();
}
}
private static Point getPoint(String points) {
if (points == null || "".equals(points)) {
throw new NullPointerException("坐标参数为空");
}
String[] split = points.split(",");
if (split.length == 0) {
throw new NullPointerException("坐标参数为空");
}
return new Point(Double.valueOf(split[0]), Double.valueOf(split[1]));
}
/**
* 作用:灰度话
*
* @param src Mat矩阵图像
* @return Mat
*/
public static Mat gray(Mat src) {
Mat gray = new Mat();
if (src.channels() == 3) {
Imgproc.cvtColor(src, gray, Imgproc.COLOR_BGR2GRAY);
src = gray;
} else {
System.out.println("The Image File Is Not The RGB File!");
}
return src;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy