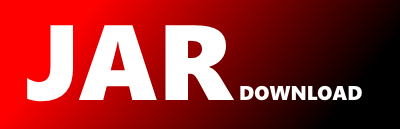
com.hn.pay.alipay.AliPayUtils Maven / Gradle / Ivy
package com.hn.pay.alipay;
import cn.hutool.log.Log;
import cn.hutool.log.LogFactory;
import com.alipay.easysdk.factory.Factory;
import com.alipay.easysdk.kernel.Config;
import com.alipay.easysdk.kernel.util.ResponseChecker;
import com.alipay.easysdk.payment.app.models.AlipayTradeAppPayResponse;
import com.alipay.easysdk.payment.common.models.AlipayTradeQueryResponse;
import com.alipay.easysdk.payment.common.models.AlipayTradeRefundResponse;
import com.alipay.easysdk.payment.page.models.AlipayTradePagePayResponse;
import com.alipay.easysdk.payment.wap.models.AlipayTradeWapPayResponse;
import com.hn.config.HnConfigUtils;
import com.hn.pay.alipay.enums.TradeStatus;
import com.hn.pay.exception.PayException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.PrintWriter;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
/**
* 描述:
* 支付宝工具类
* 支付宝新版sdk https://opendocs.alipay.com/open/54/00y8k9
* @author fei
* 2019-08-19 14:08
*/
public class AliPayUtils {
private static final Log log = LogFactory.get();
/**
* 配置前缀名
*/
private static final String CONFIG_KEY = "pay.alipay";
/**
* 应用id
*/
private static String APPID = HnConfigUtils.getConfig(CONFIG_KEY + ".appid");
/**
* 支付宝公钥
*/
private static String ALIPAY_PUBLIC_KEY = HnConfigUtils.getConfig(CONFIG_KEY + ".publicKey");
/**
* 私钥
*/
private static String PRIVATE_KEY = HnConfigUtils.getConfig(CONFIG_KEY + ".privateKey");
/**
* 网关地址
*/
private static String SERVICE_URL = HnConfigUtils.getConfig(CONFIG_KEY + ".serviceUrl");
/**
* 同步返回地址
*/
private static String NOTIFY_URL = HnConfigUtils.getConfig(CONFIG_KEY + ".notifyUrl");
/**
* 异步通知地址
*/
private static String RETURN_URL = HnConfigUtils.getConfig(CONFIG_KEY + ".returnUrl");
static {
// 1. 设置参数(全局只需设置一次)
Factory.setOptions(getOptions());
}
/**
* app支付
* @param subject 商品的标题/交易标题/订单标题/订单关键字等。
* @param outTradeNo 商户网站唯一订单号
* @param totalAmount 订单总金额,单位为元,精确到小数点后两位,取值范围[0.01,100000000]
* @return app param
*/
public static String appPay(String subject, String outTradeNo, String totalAmount) {
return appPay(subject,outTradeNo,totalAmount,null);
}
/**
* app支付
* @param subject 商品的标题/交易标题/订单标题/订单关键字等。
* @param outTradeNo 商户网站唯一订单号
* @param totalAmount 订单总金额,单位为元,精确到小数点后两位,取值范围[0.01,100000000]
* @return app param
*/
public static String appPay(String subject, String outTradeNo, String totalAmount,String notifyUrl) {
try {
AlipayTradeAppPayResponse response = Factory.Payment.App()
.asyncNotify(notifyUrl)
.pay(subject, outTradeNo, totalAmount);
if (ResponseChecker.success(response)) {
return response.getBody();
} else {
throw new PayException("支付宝app支付失败");
}
} catch (Exception e) {
log.error("支付宝app支付失败[errorMsg:{}]", e.getMessage());
throw new PayException("支付宝app支付失败:" + e.getMessage());
}
}
/**
* wap支付
*
* @param response HttpServletResponse
*/
public static void wapPay(HttpServletResponse response,
String subject, String outTradeNo, String totalAmount,
String quitUrl, String returnUrl) {
wapPay(response,subject,outTradeNo,totalAmount,quitUrl,returnUrl,null);
}
/**
* wap支付
*
* @param response HttpServletResponse
*/
public static void wapPay(HttpServletResponse response,
String subject, String outTradeNo, String totalAmount,
String quitUrl, String returnUrl,String notifyUrl) {
try {
AlipayTradeWapPayResponse payResponse = Factory.Payment.Wap()
.asyncNotify(notifyUrl)
.pay(subject, outTradeNo, totalAmount, quitUrl,returnUrl);
response.setContentType("text/html;charset=utf-8");
PrintWriter out = response.getWriter();
out.write(payResponse.getBody());
out.flush();
out.close();
} catch (Exception e) {
log.error("支付宝wapPay支付失败[errorMsg:{}]", e.getMessage());
throw new PayException("支付宝wapPay支付失败:" + e.getMessage());
}
}
/**
* PC支付
*
* @param response HttpServletResponse
*/
public static void pcPay(HttpServletResponse response, String subject,
String outTradeNo, String totalAmount, String returnUrl) {
try {
AlipayTradePagePayResponse payResponse = Factory.Payment.Page()
.pay(subject, outTradeNo, totalAmount, returnUrl);
response.setContentType("text/html;charset=utf-8");
PrintWriter out = response.getWriter();
out.write(payResponse.getBody());
out.flush();
out.close();
} catch (Exception e) {
log.error("支付宝PC支付失败[errorMsg:{}]", e.getMessage(), e.getMessage());
throw new PayException("支付宝PC支付失败:" + e.getMessage());
}
}
/**
* 交易查询
*
* @param outTradeNo 商户订单号
* @param tradeNo 支付宝交易号
* @return {@link AlipayTradeQueryResponse}
*/
public static AlipayTradeQueryResponse tradeQuery(String outTradeNo, String tradeNo) {
try {
return Factory.Payment.Common().query(outTradeNo);
} catch (Exception e) {
log.error("支付宝交易查询 失败[errorMsg:{}]", e.getMessage(), e.getMessage());
throw new PayException("支付宝交易查询失败:" + e.getMessage());
}
}
/**
* 统一收单交易退款接口
*
* @param outTradeNo 商户交易订单号
* @param refundAmount 退款金额
* @return {@link AlipayTradeRefundResponse}
* @throws PayException 支付宝 Api 异常
*/
public static AlipayTradeRefundResponse tradeRefundToResponse(String outTradeNo, String refundAmount) {
try {
return Factory.Payment.Common().refund(outTradeNo, refundAmount);
} catch (Exception e) {
log.error("统一收单交易退款接口 失败[errorMsg:{}]", e.getMessage(), e.getMessage());
throw new PayException("统一收单交易退款接口失败:" + e.getMessage());
}
}
/**
* 校验签名并且查询交易状态
*
* @param params 支付宝回调参数
* @return true成功 false失败
*/
public static boolean tradeStatusCheck(Map params) {
boolean signCheckFlag = signCheck(params);
if (signCheckFlag) {
// 签名正确
String tradeStatus = params.get("trade_status");
return TradeStatus.TRADE_SUCCESS.equals(tradeStatus);
}
return signCheckFlag;
}
/**
* 签名校验
*
* @param params 支付宝回调参数
* @return true成功 false失败
*/
public static boolean signCheck(Map params) {
try {
return Factory.Payment.Common().verifyNotify(params);
} catch (Exception e) {
log.error("支付宝签名校验 失败[errorMsg:{}]", e.getMessage());
return false;
}
}
/**
* 将异步通知的参数转化为Map
*
* @param request {HttpServletRequest}
* @return 转化后的Map
*/
public static Map toMap(HttpServletRequest request) {
Map params = new HashMap();
Map requestParams = request.getParameterMap();
for (Iterator iter = requestParams.keySet().iterator(); iter.hasNext(); ) {
String name = iter.next();
String[] values = requestParams.get(name);
String valueStr = "";
for (int i = 0; i < values.length; i++) {
valueStr = (i == values.length - 1) ? valueStr + values[i] : valueStr + values[i] + ",";
}
// 乱码解决,这段代码在出现乱码时使用
// valueStr = new String(valueStr.getBytes("ISO-8859-1"), "utf-8");
params.put(name, valueStr);
}
return params;
}
private static Config getOptions() {
Config config = new Config();
config.protocol = "https";
config.gatewayHost = "openapi.alipay.com";
config.signType = "RSA2";
config.appId = APPID;
// 为避免私钥随源码泄露,推荐从文件中读取私钥字符串而不是写入源码中
config.merchantPrivateKey = PRIVATE_KEY;
//注:证书文件路径支持设置为文件系统中的路径或CLASS_PATH中的路径,优先从文件系统中加载,加载失败后会继续尝试从CLASS_PATH中加载
// config.merchantCertPath = "<-- 请填写您的应用公钥证书文件路径,例如:/foo/appCertPublicKey_2019051064521003.crt -->";
// config.alipayCertPath = "<-- 请填写您的支付宝公钥证书文件路径,例如:/foo/alipayCertPublicKey_RSA2.crt -->";
// config.alipayRootCertPath = "<-- 请填写您的支付宝根证书文件路径,例如:/foo/alipayRootCert.crt -->";
//注:如果采用非证书模式,则无需赋值上面的三个证书路径,改为赋值如下的支付宝公钥字符串即可
config.alipayPublicKey = ALIPAY_PUBLIC_KEY;
//可设置异步通知接收服务地址(可选)
config.notifyUrl = NOTIFY_URL;
//可设置AES密钥,调用AES加解密相关接口时需要(可选)
// config.encryptKey = "<-- 请填写您的AES密钥,例如:aa4BtZ4tspm2wnXLb1ThQA== -->";
return config;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy