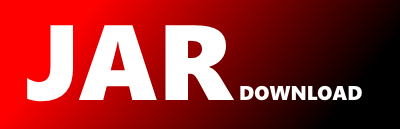
org.hibernate.examples.model.AbstractHibernateEntity Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hibernate-examples Show documentation
Show all versions of hibernate-examples Show documentation
Hibernate 2nd level cache using Redis examples.
The newest version!
package org.hibernate.examples.model;
import org.hibernate.examples.utils.HashTool;
import org.hibernate.examples.utils.ToStringHelper;
import javax.persistence.MappedSuperclass;
import javax.persistence.PostLoad;
import javax.persistence.PostPersist;
import java.io.Serializable;
/**
* Hibernate Entity의 최상위 추상화 클래스입니다.
*
* @author 배성혁 [email protected]
* @since 2013. 11. 27. 오후 2:36
*/
@MappedSuperclass
public abstract class AbstractHibernateEntity
extends AbstractPersistentObject implements HibernateEntity {
abstract public TId getId();
// NOTE: JPA 에서는 #onSave(), #onLoad() 를 @MappedSuperclass 나 @Entity 에 재정의해야한다.
// NOTE: Hibernate 에서는 Interceptor에서 관리하므로, {@link AbstractPersistentObject}에만 정의해도 된다.
@Override
@PostPersist
public final void onSave() {
setPersisted(true);
}
@Override
@PostLoad
public final void onLoad() {
setPersisted(true);
}
@Override
@SuppressWarnings("unchecked")
public boolean equals(Object obj) {
boolean isSampeType = (obj != null) && getClass().equals(obj.getClass());
if (isSampeType) {
HibernateEntity entity = (HibernateEntity) obj;
return hasSameNonDefaultIdAs(entity) ||
((!isPersisted() || !entity.isPersisted()) && hasSameBusinessSignature(entity));
}
return false;
}
@Override
public int hashCode() {
return (getId() == null) ? System.identityHashCode(this)
: HashTool.compute(getId());
}
private boolean hasSameNonDefaultIdAs(HibernateEntity entity) {
if (entity == null) return false;
TId id = getId();
TId entityId = entity.getId();
return (id != null) && (entityId != null) && (id.equals(entityId));
}
private boolean hasSameBusinessSignature(HibernateEntity other) {
boolean notNull = (other != null);
int hash = (getId() != null) ? HashTool.compute(getId()) : hashCode();
if (notNull) {
int otherHash = (other.getId() != null) ? HashTool.compute(other.getId()) : other.hashCode();
return hash == otherHash;
}
return false;
}
@Override
public ToStringHelper buildStringHelper() {
return super.buildStringHelper()
.add("id", getId());
}
private static final long serialVersionUID = 6661386933952675946L;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy