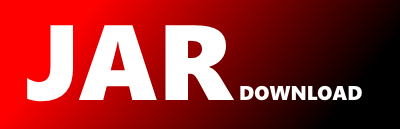
com.github.hippo.netty.HippoClientBootstrapMap Maven / Gradle / Ivy
package com.github.hippo.netty;
import java.util.Map;
import java.util.Optional;
import java.util.concurrent.ConcurrentHashMap;
/**
*
* @author sl
*
*/
public final class HippoClientBootstrapMap {
private HippoClientBootstrapMap() {}
private static final Map> BOOTSTRAPMAP =
new ConcurrentHashMap<>();
public synchronized static void put(String serviceName, String host, int port,
HippoClientBootstrap bootstrap) {
if (!BOOTSTRAPMAP.containsKey(serviceName)) {
Map map = new ConcurrentHashMap<>();
map.put(host + ":" + port, bootstrap);
BOOTSTRAPMAP.putIfAbsent(serviceName, map);
} else {
BOOTSTRAPMAP.get(serviceName).putIfAbsent(host + ":" + port, bootstrap);
}
}
public static Map get(String serviceName) {
return BOOTSTRAPMAP.get(serviceName);
}
public static boolean containsKey(String serviceName) {
return BOOTSTRAPMAP.containsKey(serviceName);
}
public static boolean containsSubKey(String serviceName, String hostAndPort) {
Map map = BOOTSTRAPMAP.get(serviceName);
if (map == null || map.isEmpty()) {
return false;
}
return map.containsKey(hostAndPort);
}
public static void remove(String serviceName, String host, int port) {
Map map = BOOTSTRAPMAP.get(serviceName);
if (map != null) {
map.remove(host + ":" + port);
}
}
public static HippoClientBootstrap getBootstrap(String serviceName) {
Map map = get(serviceName);
if (map == null || map.isEmpty()) {
return null;
}
// like round robin
Optional findFirst = map.values().stream().sorted().findFirst();
if (findFirst.isPresent()) {
HippoClientBootstrap hippoClientBootstrap = findFirst.get();
hippoClientBootstrap.getInvokeTimes().incrementAndGet();
return hippoClientBootstrap;
} else {
return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy