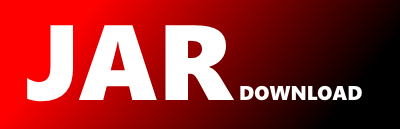
com.oreilly.servlet.HttpUtils Maven / Gradle / Ivy
The newest version!
package com.oreilly.servlet;
import jakarta.servlet.http.HttpServletRequest;
import java.util.Hashtable;
import java.util.StringTokenizer;
public class HttpUtils {
/**
* Parses a query string passed from the client to the
* server and builds a HashTable
object
* with key-value pairs.
* The query string should be in the form of a string
* packaged by the GET or POST method, that is, it
* should have key-value pairs in the form key=value,
* with each pair separated from the next by a & character.
*
* <p>A key can appear more than once in the query string
* with different values. However, the key appears only once in
* the hashtable, with its value being
* an array of strings containing the multiple values sent
* by the query string.
*
* <p>The keys and values in the hashtable are stored in their
* decoded form, so
* any + characters are converted to spaces, and characters
* sent in hexadecimal notation (like %xx) are
* converted to ASCII characters.
*
* @param s a string containing the query to be parsed
*
* @return a HashTable
object built
* from the parsed key-value pairs
*
* @exception IllegalArgumentException if the query string is invalid
*/
public static Hashtable parseQueryString(String s) {
String valArray[] = null;
if (s == null) {
throw new IllegalArgumentException();
}
Hashtable ht = new Hashtable();
StringBuilder sb = new StringBuilder();
StringTokenizer st = new StringTokenizer(s, "&");
while (st.hasMoreTokens()) {
String pair = st.nextToken();
int pos = pair.indexOf('=');
if (pos == -1) {
// XXX
// should give more detail about the illegal argument
throw new IllegalArgumentException();
}
String key = parseName(pair.substring(0, pos), sb);
String val = parseName(pair.substring(pos+1, pair.length()), sb);
if (ht.containsKey(key)) {
String oldVals[] = ht.get(key);
valArray = new String[oldVals.length + 1];
for (int i = 0; i < oldVals.length; i++) {
valArray[i] = oldVals[i];
}
valArray[oldVals.length] = val;
} else {
valArray = new String[1];
valArray[0] = val;
}
ht.put(key, valArray);
}
return ht;
}
/*
* Parse a name in the query string.
*/
private static String parseName(String s, StringBuilder sb) {
sb.setLength(0);
for (int i = 0; i < s.length(); i++) {
char c = s.charAt(i);
switch (c) {
case '+':
sb.append(' ');
break;
case '%':
try {
sb.append((char) Integer.parseInt(s.substring(i+1, i+3),
16));
i += 2;
} catch (NumberFormatException e) {
// XXX
// need to be more specific about illegal arg
throw new IllegalArgumentException();
} catch (StringIndexOutOfBoundsException e) {
String rest = s.substring(i);
sb.append(rest);
if (rest.length()==2)
i++;
}
break;
default:
sb.append(c);
break;
}
}
return sb.toString();
}
/**
*
* Reconstructs the URL the client used to make the request,
* using information in the HttpServletRequest
object.
* The returned URL contains a protocol, server name, port
* number, and server path, but it does not include query
* string parameters.
*
* <p>Because this method returns a StringBuffer
,
* not a string, you can modify the URL easily, for example,
* to append query parameters.
*
* <p>This method is useful for creating redirect messages
* and for reporting errors.
*
* @param req a HttpServletRequest
object
* containing the client's request
*
* @return a StringBuffer
object containing
* the reconstructed URL
*/
public static StringBuffer getRequestURL (HttpServletRequest req) {
StringBuffer url = new StringBuffer();
String scheme = req.getScheme ();
int port = req.getServerPort ();
String urlPath = req.getRequestURI();
//String servletPath = req.getServletPath ();
//String pathInfo = req.getPathInfo ();
url.append (scheme); // http, https
url.append ("://");
url.append (req.getServerName ());
if ((scheme.equals ("http") && port != 80)
|| (scheme.equals ("https") && port != 443)) {
url.append (':');
url.append (req.getServerPort ());
}
//if (servletPath != null)
// url.append (servletPath);
//if (pathInfo != null)
// url.append (pathInfo);
url.append(urlPath);
return url;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy