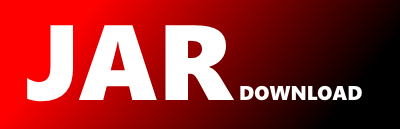
com.github.houbb.config.socket.client.proxy.ConfigServerProxy Maven / Gradle / Ivy
package com.github.houbb.config.socket.client.proxy;
import com.alibaba.fastjson.JSON;
import com.github.houbb.config.socket.client.thread.ConfigClientThread;
import com.github.houbb.config.socket.common.constant.ServiceNameConst;
import com.github.houbb.config.socket.common.req.ClientHeartbeatReq;
import com.github.houbb.config.socket.common.req.ClientRegisterReq;
import com.github.houbb.config.socket.common.req.ClientUnRegisterReq;
import com.github.houbb.config.socket.common.req.QueryPublishedConfigReq;
import com.github.houbb.config.socket.common.resp.ClientRegisterResp;
import com.github.houbb.config.socket.common.resp.ClientUnRegisterResp;
import com.github.houbb.config.socket.common.resp.QueryPublishedConfigResp;
import com.github.houbb.heaven.util.common.ArgUtil;
import com.github.houbb.log.integration.core.Log;
import com.github.houbb.log.integration.core.LogFactory;
import java.util.concurrent.Executors;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.TimeUnit;
/**
* @author binbin.hou
* @since 1.0.0
*/
public class ConfigServerProxy implements IConfigServerProxy {
private static final Log log = LogFactory.getLog(ConfigServerProxy.class);
private final ConfigClientThread clientThread;
/**
* 心跳定时任务
*
* @since 1.2.0
*/
private final ScheduledExecutorService scheduledExecutorService = Executors.newSingleThreadScheduledExecutor();
/**
* 应用名称
* @since 1.2.0
*/
private String appName;
/**
* 环境名称
* @since 1.2.0
*/
private String envName;
/**
* 机器 IP
* @since 1.2.0
*/
private String machineIp;
public void setAppName(String appName) {
this.appName = appName;
}
public void setEnvName(String envName) {
this.envName = envName;
}
public void setMachineIp(String machineIp) {
this.machineIp = machineIp;
}
public ConfigServerProxy(ConfigClientThread clientThread) {
this.clientThread = clientThread;
}
public void init() {
paramCheck();
this.clientThread.start();
// 定时执行心跳
//1S 重发一次心跳
scheduledExecutorService.scheduleAtFixedRate(new Runnable() {
@Override
public void run() {
heartbeat();
}
}, 5, 2, TimeUnit.SECONDS);
}
private void paramCheck() {
ArgUtil.notEmpty(appName, "appName");
ArgUtil.notEmpty(envName, "envName");
ArgUtil.notEmpty(machineIp, "machineIp");
}
public ClientRegisterResp register() {
final ClientRegisterReq req = new ClientRegisterReq();
req.setAppName(appName);
req.setEnvName(envName);
req.setMachineIp(machineIp);
req.setMethodType(ServiceNameConst.REGISTER);
return clientThread.callServer(req, ClientRegisterResp.class);
}
public ClientUnRegisterResp unRegister() {
final ClientUnRegisterReq req = new ClientUnRegisterReq();
req.setAppName(appName);
req.setEnvName(envName);
req.setMachineIp(machineIp);
req.setMethodType(ServiceNameConst.UN_REGISTER);
return clientThread.callServer(req, ClientUnRegisterResp.class);
}
public QueryPublishedConfigResp queryPublishedConfig() {
QueryPublishedConfigReq req = new QueryPublishedConfigReq();
req.setAppName(appName);
req.setEnvName(envName);
req.setMachineIp(machineIp);
req.setMethodType(ServiceNameConst.QUERY_PUBLISHED_CONFIG);
return clientThread.callServer(req, QueryPublishedConfigResp.class);
}
public void heartbeat() {
final ClientHeartbeatReq req = new ClientHeartbeatReq();
req.setAppName(appName);
req.setEnvName(envName);
req.setMachineIp(machineIp);
req.setMethodType(ServiceNameConst.CLIENT_HEARTBEAT);
log.debug("往服务端发送心跳包 {}", JSON.toJSON(req));
clientThread.callServer(req, null);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy