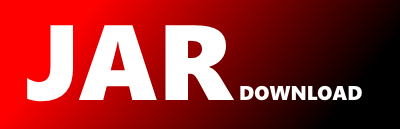
com.github.houbb.config.socket.client.rpc.handler.ChannelHandlers Maven / Gradle / Ivy
package com.github.houbb.config.socket.client.rpc.handler;
import com.github.houbb.config.socket.client.handler.ConfigClientHandler;
import com.github.houbb.config.socket.client.rpc.client.DefaultNettyClient;
import com.github.houbb.config.socket.client.rpc.dto.RpcAddress;
import com.github.houbb.config.socket.client.rpc.dto.RpcChannelFuture;
import com.github.houbb.heaven.util.guava.Guavas;
import com.github.houbb.heaven.util.util.CollectionUtil;
import io.netty.channel.Channel;
import io.netty.channel.ChannelFuture;
import io.netty.channel.ChannelHandler;
import io.netty.channel.ChannelInitializer;
import io.netty.handler.codec.serialization.ClassResolvers;
import io.netty.handler.codec.serialization.ObjectDecoder;
import io.netty.handler.codec.serialization.ObjectEncoder;
import io.netty.handler.logging.LogLevel;
import io.netty.handler.logging.LoggingHandler;
import java.util.List;
/**
* channel 工具类
* @author binbin.hou
* @since 0.0.8
*/
public final class ChannelHandlers {
private ChannelHandlers(){}
/**
* 包含默认 object 编码解码的 handler
* @param channelHandlers 用户自定义 handler
* @return channel handler
* @since 0.0.8
*/
public static ChannelHandler objectCodecHandler(final ChannelHandler ... channelHandlers) {
return new ChannelInitializer() {
@Override
protected void initChannel(Channel ch) throws Exception {
ch.pipeline()
// 解码 bytes=>resp
.addLast(new ObjectDecoder(Integer.MAX_VALUE, ClassResolvers.cacheDisabled(null)))
// request=>bytes
.addLast(new ObjectEncoder())
.addLast(channelHandlers);
}
};
}
/**
* 日志 handler
* @param channelHandlers channel
* @return 结果
* @since 1.1.0
*/
public static ChannelHandler logHandler(final ChannelHandler ... channelHandlers) {
return new ChannelInitializer(){
@Override
protected void initChannel(Channel ch) throws Exception {
ch.pipeline()
.addLast(new LoggingHandler(LogLevel.INFO))
.addLast(channelHandlers);
}
};
}
/**
* 包含默认 object 编码解码的 + 日志 handler
* @param channelHandlers 用户自定义 handler
* @return channel handler
* @since 0.0.8
*/
public static ChannelHandler objectCodecLogHandler(final ChannelHandler ... channelHandlers) {
final ChannelHandler objectCodecHandler = objectCodecHandler(channelHandlers);
return new ChannelInitializer() {
@Override
protected void initChannel(Channel ch) throws Exception {
ch.pipeline()
.addLast(new LoggingHandler(LogLevel.INFO))
.addLast(objectCodecHandler)
.addLast(channelHandlers);
}
};
}
/**
* 获取处理后的channel future 列表信息
* (1)权重
* (2)client 链接信息
* (3)地址信息
* @param rpcAddressList 地址信息列表
* @param handlerFactory 构建工厂
* @return 信息列表
* @since 0.0.9
*/
public static List channelFutureList(final List rpcAddressList, final ChannelHandlerFactory handlerFactory) {
final ChannelHandler channelHandler = handlerFactory.handler();
return channelFutureList(rpcAddressList, channelHandler);
}
/**
* 获取处理后的channel future 列表信息
* (1)权重
* (2)client 链接信息
* (3)地址信息
* @param rpcAddressList 地址信息列表
* @param channelHandler 构建工厂
* @return 信息列表
* @since 0.0.9
*/
public static List channelFutureList(final List rpcAddressList, final ChannelHandler channelHandler) {
List resultList = Guavas.newArrayList();
if(CollectionUtil.isNotEmpty(rpcAddressList)) {
for(RpcAddress rpcAddress : rpcAddressList) {
// 循环中每次都需要一个新的 handler
RpcChannelFuture future = RpcChannelFuture.newInstance();
DefaultNettyClient nettyClient = DefaultNettyClient.newInstance(rpcAddress.address(), rpcAddress.port(), channelHandler);
ChannelFuture channelFuture = nettyClient.call();
future.channelFuture(channelFuture).address(rpcAddress);
resultList.add(future);
}
}
return resultList;
}
/**
* 获取处理后的channel future 信息
* (1)权重
* (2)client 链接信息
* (3)地址信息
* @param rpcAddress 地址信息
* @param handlerFactory 构建工厂
* @return 信息列表
* @since 0.1.6
*/
public static RpcChannelFuture channelFuture(final RpcAddress rpcAddress, final ChannelHandlerFactory handlerFactory) {
final ChannelHandler channelHandler = handlerFactory.handler();
// 循环中每次都需要一个新的 handler
RpcChannelFuture future = RpcChannelFuture.newInstance();
DefaultNettyClient nettyClient = DefaultNettyClient.newInstance(rpcAddress.address(), rpcAddress.port(), channelHandler);
ChannelFuture channelFuture = nettyClient.call();
future.channelFuture(channelFuture)
.address(rpcAddress);
return future;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy