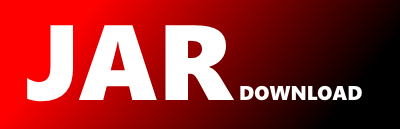
com.github.houbb.config.socket.client.thread.ConfigClientThread Maven / Gradle / Ivy
/*
* Copyright (c) 2019. houbinbin Inc.
* rpc All rights reserved.
*/
package com.github.houbb.config.socket.client.thread;
import com.alibaba.fastjson.JSON;
import com.github.houbb.config.socket.client.handler.ConfigClientHandler;
import com.github.houbb.config.socket.client.rpc.dto.RpcAddress;
import com.github.houbb.config.socket.client.rpc.dto.RpcChannelFuture;
import com.github.houbb.config.socket.client.rpc.handler.ChannelHandlers;
import com.github.houbb.config.socket.client.support.invoke.InvokeService;
import com.github.houbb.config.socket.client.support.invoke.impl.DefaultInvokeService;
import com.github.houbb.config.socket.client.support.listener.ConfigNotifyListener;
import com.github.houbb.config.socket.common.exception.ConfigTimeoutException;
import com.github.houbb.config.socket.common.exception.RespCode;
import com.github.houbb.config.socket.common.req.CommonReq;
import com.github.houbb.config.socket.common.resp.CommonResp;
import com.github.houbb.config.socket.common.rpc.RpcMessageDto;
import com.github.houbb.config.socket.common.util.BeanUtils;
import com.github.houbb.heaven.util.id.impl.Ids;
import com.github.houbb.log.integration.core.Log;
import com.github.houbb.log.integration.core.LogFactory;
import io.netty.bootstrap.Bootstrap;
import io.netty.buffer.ByteBuf;
import io.netty.buffer.Unpooled;
import io.netty.channel.*;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.nio.NioSocketChannel;
import io.netty.handler.logging.LogLevel;
import io.netty.handler.logging.LoggingHandler;
import java.util.List;
/**
* rpc 客户端
*
* Created: 2019/10/16 11:21 下午
* Project: rpc
*
* @author houbinbin
* @since 0.0.2
*/
public class ConfigClientThread {
private static final Log log = LogFactory.getLog(ConfigClientThread.class);
private List rpcAddressList;
/**
* 客户端处理 handler
* @since 0.0.4
*/
private ConfigClientHandler channelHandler;
/**
* 调用服务管理
* @since 1.0.0
*/
private final InvokeService invokeService = new DefaultInvokeService();
/**
* 配置监听类
*/
private ConfigNotifyListener listener;
private List rpcChannelFutures;
/**
* 超时时间
* 单位:毫秒
*/
private long timeoutMills;
public void start() {
this.channelHandler = new ConfigClientHandler(invokeService, listener);
this.rpcChannelFutures = ChannelHandlers.channelFutureList(rpcAddressList, channelHandler);
}
private Channel getChannel() {
return this.rpcChannelFutures.get(0).channelFuture().channel();
}
public ConfigClientThread rpcAddressList(List rpcAddressList) {
this.rpcAddressList = rpcAddressList;
return this;
}
public ConfigClientThread timeoutMills(long timeoutMills) {
this.timeoutMills = timeoutMills;
return this;
}
public ConfigClientThread listener(ConfigNotifyListener listener) {
this.listener = listener;
return this;
}
/**
* 开始运行
*/
@Deprecated
public void start2() {
// 启动服务端
log.info("RPC 服务开始启动客户端");
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
Bootstrap bootstrap = new Bootstrap();
ChannelFuture channelFuture = bootstrap.group(workerGroup)
.channel(NioSocketChannel.class)
.option(ChannelOption.SO_KEEPALIVE, true)
.handler(new ChannelInitializer(){
@Override
protected void initChannel(Channel ch) throws Exception {
channelHandler = new ConfigClientHandler(invokeService, listener);
ch.pipeline()
.addLast(new LoggingHandler(LogLevel.INFO))
.addLast(channelHandler);
}
})
.connect("127.0.0.1", 8888)
.syncUninterruptibly();
log.info("RPC 服务启动客户端完成,监听端口:" + 8888);
} catch (Exception e) {
log.error("RPC 客户端遇到异常", e);
throw new RuntimeException(e);
}
// 不要关闭线程池!!!
}
public R callServer(T commonReq, Class respClass) {
final String traceId = Ids.uuid32();
commonReq.setTraceId(traceId);
commonReq.setRequestTime(System.currentTimeMillis());
RpcMessageDto rpcMessageDto = new RpcMessageDto();
BeanUtils.copyProperties(commonReq, rpcMessageDto);
rpcMessageDto.setJson(JSON.toJSONString(commonReq));
// 请求类
rpcMessageDto.setRequest(true);
// 关闭当前线程,以获取对应的信息
// 使用序列化的方式
final byte[] bytes = JSON.toJSONBytes(rpcMessageDto);
ByteBuf byteBuf = Unpooled.copiedBuffer(bytes);
// 添加调用服务
invokeService.addRequest(traceId, timeoutMills);
// 遍历 channel
Channel channel = getChannel();
channel.writeAndFlush(byteBuf);
log.info("client 发送消息 {}", JSON.toJSON(commonReq));
// channel.closeFuture().syncUninterruptibly();
if(respClass == null) {
log.debug("当前消息为 oneway 消息,忽略响应");
return null;
} else {
//channelHandler 中获取对应的响应
RpcMessageDto messageDto = invokeService.getResponse(commonReq.getTraceId());
if(RespCode.TIMEOUT.getCode().equals(messageDto.getRespCode())) {
throw new ConfigTimeoutException();
}
String respJson = messageDto.getJson();
return JSON.parseObject(respJson, respClass);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy