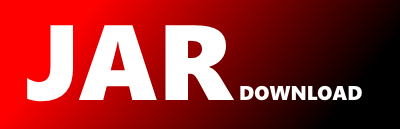
com.github.houbb.config.socket.client.bs.ConfigSocketClientBs Maven / Gradle / Ivy
package com.github.houbb.config.socket.client.bs;
import com.github.houbb.config.socket.client.proxy.ConfigServerProxy;
import com.github.houbb.config.socket.client.rpc.dto.RpcAddress;
import com.github.houbb.config.socket.client.support.invoke.InvokeService;
import com.github.houbb.config.socket.client.support.invoke.impl.DefaultInvokeService;
import com.github.houbb.config.socket.client.support.listener.ConfigNotifyListener;
import com.github.houbb.config.socket.client.support.listener.ConfigNotifyListeners;
import com.github.houbb.config.socket.client.thread.ConfigClientThread;
import com.github.houbb.config.socket.common.util.DelimiterUtil;
import com.github.houbb.heaven.util.common.ArgUtil;
import com.github.houbb.heaven.util.net.NetUtil;
import com.github.houbb.load.balance.api.ILoadBalance;
import com.github.houbb.load.balance.api.impl.LoadBalances;
import java.util.ArrayList;
import java.util.List;
/**
* @author binbin.hou
* @since 1.1.0
*/
public final class ConfigSocketClientBs {
private ConfigSocketClientBs(){}
public static ConfigSocketClientBs newInstance() {
return new ConfigSocketClientBs();
}
/**
* 应用名称
* @since 1.2.0
*/
private String appName = "demo";
/**
* 环境名称
* @since 1.2.0
*/
private String envName = "test";
/**
* 机器标识
* @since 1.2.0
*/
private String machineIp = NetUtil.getLocalHost();
/**
* 服务端地址
*
* 127.0.0.1:9527:1
*
* 第三个是权重,默认为1
*
* 多个之间使用逗号隔开
*/
private String serverAddress = "127.0.0.1:9527";
/**
* 配置监听类
*
* @since 1.0.0
*/
private ConfigNotifyListener listener = ConfigNotifyListeners.defaults();
/**
* 超时时间
* 单位:毫秒
*/
private long timeoutMills = 60 * 1000;
/**
* 粘包处理分隔符
* @since 1.3.0
*/
private String delimiter = DelimiterUtil.DELIMITER;
/**
* 负载均衡
* @since 1.4.0
*/
private ILoadBalance loadBalance = LoadBalances.random();
public ConfigSocketClientBs appName(String appName) {
ArgUtil.notEmpty(appName, "appName");
this.appName = appName;
return this;
}
public ConfigSocketClientBs envName(String envName) {
ArgUtil.notEmpty(envName, "envName");
this.envName = envName;
return this;
}
public ConfigSocketClientBs machineIp(String machineIp) {
ArgUtil.notEmpty(machineIp, "machineIp");
this.machineIp = machineIp;
return this;
}
public ConfigSocketClientBs serverAddress(String serverAddress) {
ArgUtil.notEmpty(serverAddress, "serverAddress");
this.serverAddress = serverAddress;
return this;
}
public ConfigSocketClientBs listener(ConfigNotifyListener listener) {
ArgUtil.notNull(listener, "listener");
this.listener = listener;
return this;
}
public ConfigSocketClientBs timeoutMills(long timeoutMills) {
this.timeoutMills = timeoutMills;
return this;
}
public ConfigSocketClientBs delimiter(String delimiter) {
ArgUtil.notEmpty(delimiter, "delimiter");
this.delimiter = delimiter;
return this;
}
public ConfigSocketClientBs loadBalance(ILoadBalance loadBalance) {
ArgUtil.notNull(loadBalance, "loadBalance");
this.loadBalance = loadBalance;
return this;
}
public ConfigServerProxy proxy() {
List rpcAddressList = getRpcAddressList(this.serverAddress);
ArgUtil.notEmpty(rpcAddressList, "rpcAddressList");
//1. 构建 thread
ConfigClientThread clientThread = new ConfigClientThread();
clientThread.rpcAddressList(rpcAddressList);
clientThread.timeoutMills(timeoutMills);
clientThread.listener(listener);
clientThread.delimiter(delimiter);
clientThread.loadBalance(loadBalance);
//2. 构建 proxy
ConfigServerProxy proxy = new ConfigServerProxy(clientThread);
proxy.setAppName(appName);
proxy.setEnvName(envName);
proxy.setMachineIp(machineIp);
//3. 启动
proxy.init();
return proxy;
}
private List getRpcAddressList(String serverAddress) {
String[] strings = serverAddress.split(",");
List list = new ArrayList<>();
for(String string : strings) {
String[] array = string.split(":");
if(array.length == 3) {
RpcAddress rpcAddress = new RpcAddress(array[0], Integer.parseInt(array[1]), Integer.parseInt(array[2]));
list.add(rpcAddress);
} else if(array.length == 2) {
RpcAddress rpcAddress = new RpcAddress(array[0], Integer.parseInt(array[1]));
list.add(rpcAddress);
}
}
return list;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy