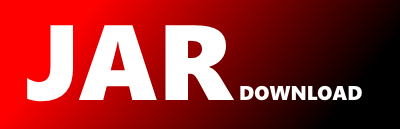
com.github.houbb.keyword.bs.KeywordBs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of keyword Show documentation
Show all versions of keyword Show documentation
The keyword tool for java.(java nlp 分词关键词提取工具。)
package com.github.houbb.keyword.bs;
import com.github.houbb.heaven.support.instance.impl.Instances;
import com.github.houbb.heaven.util.common.ArgUtil;
import com.github.houbb.keyword.api.IKeyword;
import com.github.houbb.keyword.api.IKeywordContext;
import com.github.houbb.keyword.api.IKeywordResult;
import com.github.houbb.keyword.api.impl.Keyword;
import com.github.houbb.keyword.api.impl.KeywordContext;
import com.github.houbb.keyword.support.data.idf.IKeywordIdfData;
import com.github.houbb.keyword.support.data.idf.impl.KeywordIdfDatas;
import com.github.houbb.keyword.support.data.stop.IKeywordStopWordData;
import com.github.houbb.keyword.support.data.stop.impl.KeywordDatas;
import com.github.houbb.keyword.support.result.IKeywordResultHandler;
import com.github.houbb.keyword.support.segment.IKeywordSegment;
import com.github.houbb.keyword.support.segment.impl.KeywordSegments;
import com.github.houbb.keyword.support.topk.IKeywordTopk;
import com.github.houbb.keyword.support.topk.impl.KeywordTopks;
import java.util.List;
/**
* @author binbin.hou
* @since 0.0.1
*/
public final class KeywordBs {
private KeywordBs() {
}
/**
* 新建对象实例
* @return 结果
* @since 0.0.1
*/
public static KeywordBs newInstance() {
return new KeywordBs();
}
/**
* 核心实现
*
* @since 0.0.1
*/
private IKeyword keyword = Instances.singleton(Keyword.class);
/**
* 默认停顿词数据
*
* @since 0.0.1
*/
private IKeywordStopWordData stopWordData = KeywordDatas.system();
/**
* 逆文档频率的默认实现
*
* @since 0.0.1
*/
private IKeywordIdfData idfData = KeywordIdfDatas.defaults();
/**
* 默认分词实现
*
* @since 0.0.1
*/
private IKeywordSegment segment = KeywordSegments.defaults();
/**
* topk 算法
* @since 0.0.1
*/
private IKeywordTopk topk = KeywordTopks.sort();
public KeywordBs stopWordData(IKeywordStopWordData stopWordData) {
ArgUtil.notNull(stopWordData, "stopWordData");
this.stopWordData = stopWordData;
return this;
}
public KeywordBs idfData(IKeywordIdfData idfData) {
ArgUtil.notNull(idfData, "idfData");
this.idfData = idfData;
return this;
}
public KeywordBs segment(IKeywordSegment segment) {
ArgUtil.notNull(segment, "segment");
this.segment = segment;
return this;
}
public KeywordBs topk(IKeywordTopk topk) {
ArgUtil.notNull(topk, "topk");
this.topk = topk;
return this;
}
/**
* 关键词实现
*
* @param text 文本信息
* @param limit 限制数量
* @param handler 处理实现类
* @param 泛型
* @return 关键词列表
* @since 0.0.1
*/
public R keyword(final String text, final int limit,
final IKeywordResultHandler handler) {
IKeywordContext context = buildContext().limit(limit);
List resultList = keyword.keyword(text, context);
return handler.handler(resultList);
}
/**
* 构建上下文
*
* @return 上下文
* @since 0.0.1
*/
private KeywordContext buildContext() {
return KeywordContext.newInstance()
.segment(segment)
.stopWordData(stopWordData)
.idfData(idfData)
.topk(topk);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy