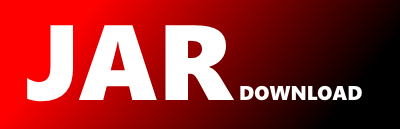
com.github.houbb.redis.client.jedis.pooled.JedisClientPool Maven / Gradle / Ivy
The newest version!
package com.github.houbb.redis.client.jedis.pooled;
import com.github.houbb.heaven.util.common.ArgUtil;
import com.github.houbb.redis.client.api.client.IRedisClient;
import com.github.houbb.redis.client.api.pool.IRedisClientPool;
import com.github.houbb.redis.client.api.pool.IRedisClientPoolConfig;
import com.github.houbb.redis.client.jedis.client.JedisClient;
import redis.clients.jedis.Jedis;
import redis.clients.jedis.JedisPool;
import redis.clients.jedis.JedisPoolConfig;
/**
* @author binbin.hou
* @since 0.0.3
*/
public class JedisClientPool implements IRedisClientPool {
/**
* 池化的配置信息
* @since 0.0.3
*/
private IRedisClientPoolConfig poolConfig;
/**
* 请求地址
* @since 0.0.3
*/
private String host;
/**
* 端口号
* @since 0.0.3
*/
private int port;
/**
* 密码
* @since 0.0.3
*/
private String password;
/**
* 超时时间
* @since 0.0.3
*/
private int timeoutMills = 5000;
/**
* 核心类
* @since 0.0.3
*/
private volatile JedisPool jedisPool = null;
public static JedisClientPool newInstance() {
return new JedisClientPool();
}
/**
* 设置连接池配置
* @param poolConfig 连接池配置
* @return this
* @since 0.0.3
*/
public JedisClientPool poolConfig(IRedisClientPoolConfig poolConfig) {
ArgUtil.notNull(poolConfig, "poolConfig");
this.poolConfig = poolConfig;
return this;
}
public JedisClientPool host(String host) {
this.host = host;
return this;
}
public JedisClientPool port(int port) {
this.port = port;
return this;
}
public JedisClientPool password(String password) {
this.password = password;
return this;
}
public JedisClientPool timeoutMills(int timeoutMills) {
this.timeoutMills = timeoutMills;
return this;
}
@Override
public IRedisClient getRedisClient() {
//DLC
if(jedisPool == null) {
synchronized (this) {
if(jedisPool == null) {
init();
}
}
}
Jedis jedis = this.jedisPool.getResource();
return new JedisClient(jedis, password);
}
/**
* 初始化 jedis 连接信息
* @since 0.0.3
*/
private void init() {
JedisPoolConfig config = new JedisPoolConfig();
config.setBlockWhenExhausted(poolConfig.blockWhenExhausted());
config.setMaxWaitMillis(poolConfig.maxWaitMillis());
config.setMaxTotal(poolConfig.maxTotal());
config.setMinIdle(poolConfig.minIdle());
config.setMaxIdle(poolConfig.maxIdle());
config.setTestOnBorrow(poolConfig.testOnBorrow());
config.setTestOnReturn(poolConfig.testOnReturn());
this.jedisPool = new JedisPool(config, host, port, timeoutMills, password);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy