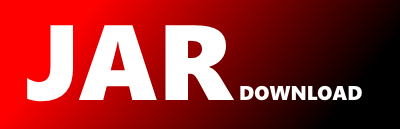
com.github.housepower.jdbc.ClickHouseConnection Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.github.housepower.jdbc;
import com.github.housepower.client.NativeClient;
import com.github.housepower.client.NativeContext;
import com.github.housepower.client.SessionState;
import com.github.housepower.data.Block;
import com.github.housepower.data.DataTypeFactory;
import com.github.housepower.jdbc.statement.ClickHousePreparedInsertStatement;
import com.github.housepower.jdbc.statement.ClickHousePreparedQueryStatement;
import com.github.housepower.jdbc.statement.ClickHouseStatement;
import com.github.housepower.jdbc.wrapper.SQLConnection;
import com.github.housepower.log.Logger;
import com.github.housepower.log.LoggerFactory;
import com.github.housepower.misc.Validate;
import com.github.housepower.protocol.HelloResponse;
import com.github.housepower.settings.ClickHouseConfig;
import com.github.housepower.settings.ClickHouseDefines;
import com.github.housepower.stream.QueryResult;
import javax.annotation.Nullable;
import java.net.InetSocketAddress;
import java.sql.Array;
import java.sql.ClientInfoStatus;
import java.sql.Connection;
import java.sql.DatabaseMetaData;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLClientInfoException;
import java.sql.SQLException;
import java.sql.SQLWarning;
import java.sql.Statement;
import java.sql.Struct;
import java.time.Duration;
import java.time.ZoneId;
import java.util.HashMap;
import java.util.Map;
import java.util.Properties;
import java.util.concurrent.Executor;
import java.util.concurrent.atomic.AtomicBoolean;
import java.util.concurrent.atomic.AtomicReference;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import static com.github.housepower.jdbc.ClickhouseJdbcUrlParser.PORT_DELIMITER;
public class ClickHouseConnection implements SQLConnection {
private static final Logger LOG = LoggerFactory.getLogger(ClickHouseConnection.class);
private static final Pattern VALUES_REGEX = Pattern.compile("[Vv][Aa][Ll][Uu][Ee][Ss]\\s*\\(");
private final AtomicBoolean isClosed;
private final AtomicReference cfg;
// TODO move to NativeClient
private final AtomicReference state = new AtomicReference<>(SessionState.IDLE);
private volatile NativeContext nativeCtx;
protected ClickHouseConnection(ClickHouseConfig cfg, NativeContext nativeCtx) {
this.isClosed = new AtomicBoolean(false);
this.cfg = new AtomicReference<>(cfg);
this.nativeCtx = nativeCtx;
}
public ClickHouseConfig cfg() {
return cfg.get();
}
public NativeContext.ServerContext serverContext() {
return nativeCtx.serverCtx();
}
public NativeContext.ClientContext clientContext() {
return nativeCtx.clientCtx();
}
@Override
public void setAutoCommit(boolean autoCommit) throws SQLException {
}
@Override
public boolean getAutoCommit() throws SQLException {
return true;
}
@Override
public void commit() throws SQLException {
}
@Override
public void rollback() throws SQLException {
}
@Override
public void setReadOnly(boolean readOnly) throws SQLException {
}
@Override
public boolean isReadOnly() throws SQLException {
return false;
}
@Override
public Map> getTypeMap() throws SQLException {
return null;
}
@Override
public void setTypeMap(Map> map) throws SQLException {
}
@Override
public void setHoldability(int holdability) throws SQLException {
}
@Override
public int getHoldability() throws SQLException {
return ResultSet.CLOSE_CURSORS_AT_COMMIT;
}
@Override
public void setNetworkTimeout(Executor executor, int milliseconds) throws SQLException {
}
@Override
public int getNetworkTimeout() throws SQLException {
return 0;
}
@Override
public void abort(Executor executor) throws SQLException {
this.close();
}
@Override
public void close() throws SQLException {
if (!isClosed() && isClosed.compareAndSet(false, true)) {
NativeClient nativeClient = nativeCtx.nativeClient();
nativeClient.disconnect();
}
}
@Override
public boolean isClosed() throws SQLException {
return isClosed.get();
}
@Override
public Statement createStatement() throws SQLException {
Validate.isTrue(!isClosed(), "Unable to create Statement, because the connection is closed.");
return new ClickHouseStatement(this, nativeCtx);
}
@Override
public PreparedStatement prepareStatement(String query) throws SQLException {
Validate.isTrue(!isClosed(), "Unable to create PreparedStatement, because the connection is closed.");
Matcher matcher = VALUES_REGEX.matcher(query);
return matcher.find() ? new ClickHousePreparedInsertStatement(matcher.end() - 1, query, this, nativeCtx) :
new ClickHousePreparedQueryStatement(this, nativeCtx, query);
}
@Override
public PreparedStatement prepareStatement(String sql, int resultSetType, int resultSetConcurrency) throws SQLException {
return this.prepareStatement(sql);
}
@Override
public void setClientInfo(Properties properties) throws SQLClientInfoException {
try {
cfg.set(ClickHouseConfig.Builder.builder(cfg.get()).withProperties(properties).build());
} catch (Exception ex) {
Map failed = new HashMap<>();
for (Map.Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy