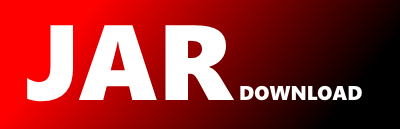
com.github.housepower.misc.ExceptionUtil Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.github.housepower.misc;
import com.github.housepower.exception.ClickHouseException;
import com.github.housepower.exception.ClickHouseSQLException;
import com.github.housepower.settings.ClickHouseErrCode;
import javax.annotation.Nullable;
import java.util.function.BiFunction;
import java.util.function.Function;
import java.util.function.Supplier;
public class ExceptionUtil {
public static RuntimeException unchecked(Exception checked) {
return new RuntimeException(checked);
}
public static Function unchecked(CheckedFunction checked) {
return t -> {
try {
return checked.apply(t);
} catch (Exception rethrow) {
throw unchecked(rethrow);
}
};
}
public static BiFunction unchecked(CheckedBiFunction checked) {
return (t, u) -> {
try {
return checked.apply(t, u);
} catch (Exception rethrow) {
throw unchecked(rethrow);
}
};
}
public static Supplier unchecked(CheckedSupplier checked) {
return () -> {
try {
return checked.get();
} catch (Exception rethrow) {
throw unchecked(rethrow);
}
};
}
public static void rethrowSQLException(CheckedRunnable checked) throws ClickHouseSQLException {
try {
checked.run();
} catch (Exception rethrow) {
int errCode = ClickHouseErrCode.UNKNOWN_ERROR.code();
ClickHouseException ex = ExceptionUtil.recursiveFind(rethrow, ClickHouseException.class);
if (ex != null)
errCode = ex.errCode();
throw new ClickHouseSQLException(errCode, rethrow.getMessage(), rethrow);
}
}
public static T rethrowSQLException(CheckedSupplier checked) throws ClickHouseSQLException {
try {
return checked.get();
} catch (Exception rethrow) {
int errCode = ClickHouseErrCode.UNKNOWN_ERROR.code();
ClickHouseException ex = ExceptionUtil.recursiveFind(rethrow, ClickHouseException.class);
if (ex != null)
errCode = ex.errCode();
throw new ClickHouseSQLException(errCode, rethrow.getMessage(), rethrow);
}
}
@Nullable
@SuppressWarnings("unchecked")
public static T recursiveFind(Throwable th, Class expectedClz) {
Throwable nest = th;
while (nest != null) {
if (expectedClz.isAssignableFrom(nest.getClass())) {
return (T) nest;
}
nest = nest.getCause();
}
return null;
}
@FunctionalInterface
public interface CheckedFunction {
R apply(T t) throws Exception;
}
@FunctionalInterface
public interface CheckedBiFunction {
R apply(T t, U u) throws Exception;
}
@FunctionalInterface
public interface CheckedBiConsumer {
void accept(T t, U u) throws Exception;
}
@FunctionalInterface
public interface CheckedRunnable {
void run() throws Exception;
}
@FunctionalInterface
public interface CheckedSupplier {
T get() throws Exception;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy