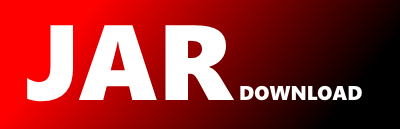
com.pronoia.hapi.GenericMessageUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hapi-junit Show documentation
Show all versions of hapi-junit Show documentation
A library of JUnit assertions to assist in testing with HAPI messages.
/**
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.pronoia.hapi;
import ca.uhn.hl7v2.HL7Exception;
import ca.uhn.hl7v2.model.GenericMessage;
import ca.uhn.hl7v2.model.Message;
import ca.uhn.hl7v2.model.Segment;
import ca.uhn.hl7v2.model.Structure;
import ca.uhn.hl7v2.parser.EncodingCharacters;
import ca.uhn.hl7v2.util.Terser;
public class GenericMessageUtil extends MessageUtil {
public GenericMessageUtil() {
super(HapiTestUtil.createGenericHapiContext());
}
@Override
public GenericMessage parse(final String er7Data) {
try {
return (GenericMessage)hapiContext.getPipeParser().parse(er7Data);
} catch (Exception hl7Ex) {
final String exceptionMessage = String.format("Exception encountered while attempting to parse message from <%s>", er7Data);
throw new HapiTestException(exceptionMessage, hl7Ex);
}
}
public String[] convertToArrayOfSegmentStrings(final GenericMessage message) {
return convertToArrayOfSegmentStrings(encode(message));
}
public String encode(GenericMessage message) {
try {
return message.encode();
} catch (HL7Exception encodeEx) {
String exceptionMessage = String.format("Exception encountered encoding %s message", message.getClass());
throw new HapiTestException(exceptionMessage, encodeEx);
}
}
public String encode(GenericMessage message, String segmentName) {
Segment segment = findNonEmptySegment(segmentName, message);
try {
// TODO: Remove this workaround once https://github.com/hapifhir/hapi-hl7v2/issues/15 is addressed
Segment mshSegment = (Segment)message.get("MSH");
char fieldSeparator = Terser.get(mshSegment, 1, 0, 1, 1).charAt(0);
String delimiters = Terser.get(mshSegment, 2, 0, 1, 1);
EncodingCharacters enc = new EncodingCharacters(fieldSeparator, delimiters);
return message.getParser().doEncode(segment, enc);
} catch (HL7Exception genericEncodeEx) {
String exceptionMessage = String.format("Exception encountered encoding <%s> message <%s - %s> segment using parser <%s>",
message.getClass(), segmentName, segment.getClass(), message.getParser().getClass());
throw new HapiTestException(exceptionMessage, genericEncodeEx);
}
}
public T parse(final String er7Data, Class messageClass) {
try {
Message message = hapiContext.newMessage(messageClass);
message.parse(er7Data);
return (T)message;
} catch (Exception hl7Ex) {
final String exceptionMessage = String.format("Exception encountered while attempting to parse %s message from <%s>",
messageClass, er7Data);
throw new HapiTestException(exceptionMessage, hl7Ex);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy