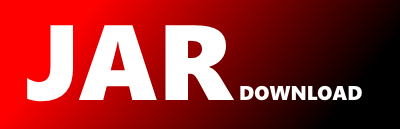
com.pronoia.hapi.MessageUtil Maven / Gradle / Ivy
/**
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.pronoia.hapi;
import java.io.IOException;
import ca.uhn.hl7v2.DefaultHapiContext;
import ca.uhn.hl7v2.HL7Exception;
import ca.uhn.hl7v2.Version;
import ca.uhn.hl7v2.model.AbstractMessage;
import ca.uhn.hl7v2.model.Message;
import ca.uhn.hl7v2.model.Segment;
import ca.uhn.hl7v2.parser.EncodingCharacters;
import ca.uhn.hl7v2.util.SegmentFinder;
import ca.uhn.hl7v2.util.Terser;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public abstract class MessageUtil {
protected Logger log = LoggerFactory.getLogger(this.getClass());
protected final DefaultHapiContext hapiContext;
public MessageUtil(DefaultHapiContext hapiContext) {
this.hapiContext = hapiContext;
}
public MessageUtil(DefaultHapiContext hapiContext, EncodingCharacters encodingCharacters) {
this.hapiContext = hapiContext;
}
public DefaultHapiContext getHapiContext() {
return hapiContext;
}
public abstract Message parse(String er7Data);
public T createMessage(Class messageClass) {
try {
return hapiContext.newMessage(messageClass);
} catch (HL7Exception createEx) {
String exceptionMessage = String.format("Failed to create new message based on information in %s message class", messageClass.getName());
throw new HapiTestException(exceptionMessage, createEx);
}
}
public T createMessage(T messageType) {
try {
Terser messageTypeTerser = new Terser(messageType);
return (T) createMessage(messageTypeTerser.get("MSH-9-1"), messageTypeTerser.get("MSH-9-2"), messageType.getVersion());
} catch (HL7Exception createEx) {
String exceptionMessage = String.format("Failed to create new message based on information in %s message class", messageType.getClass());
throw new HapiTestException(exceptionMessage, createEx);
}
}
public T createMessage(Class messageClass, U messageType) {
try {
AbstractMessage newMessage = (AbstractMessage)hapiContext.newMessage(messageClass);
Terser messageTypeTerser = new Terser(messageType);
newMessage.initQuickstart(messageTypeTerser.get("MSH-9-1"), messageTypeTerser.get("MSH-9-2"), messageType.getVersion());
return (T) newMessage;
} catch (IOException | HL7Exception createEx) {
String exceptionMessage = String.format("Failed to create new message based on information in %s message class", messageType.getClass());
throw new HapiTestException(exceptionMessage, createEx);
}
}
public Message createMessage(String messageType, String triggerEvent, String version) {
return createMessage(messageType, triggerEvent, Version.versionOf(version));
}
public Message createMessage(String messageType, String triggerEvent, Version version) {
Message message = null;
try {
message = hapiContext.newMessage(messageType, triggerEvent, version);
} catch (HL7Exception createEx) {
String exceptionMessage = String.format("Failed to create new message for %s message type, %s trigger event and %s version",
messageType, triggerEvent, version);
throw new HapiTestException(exceptionMessage, createEx);
}
return message;
}
public Terser createTerser(final Message message) {
return new Terser(message);
}
public Terser createTerser(final String er7Data) {
return createTerser(parse(er7Data));
}
public String[] convertToArrayOfSegmentStrings(final String message) {
return message.split("\r");
}
public Segment findSegment(final String segmentName, final Message message) {
return findSegment(segmentName, 0, message);
}
public Segment findSegment(final String segmentName, int repetition, final Message message) {
try {
return new SegmentFinder(message).findSegment(segmentName, repetition);
} catch (HL7Exception hl7Ex) {
final String exceptionMessage = String.format("Exception encountered while attempting to find %s segment repetition %d in %s message",
segmentName, repetition, message.getClass().getSimpleName());
throw new HapiTestException(exceptionMessage, hl7Ex);
}
}
public Segment findNonEmptySegment(final String segmentName, final Message message) {
return findNonEmptySegment(segmentName, 0, message);
}
public Segment findNonEmptySegment(final String segmentName, int repetition, final Message message) {
SegmentFinder segmentFinder = new SegmentFinder(message);
Segment segment;
try {
do {
segment = segmentFinder.findSegment(segmentName, repetition);
} while (segment == null || segment.isEmpty());
} catch (HL7Exception hl7Ex) {
final String exceptionMessage = String.format("Exception encountered while attempting to find a non-empty %s segment repetition %d in %s message",
segmentName, repetition, message.getClass().getSimpleName());
throw new HapiTestException(exceptionMessage, hl7Ex);
}
return segment;
}
public Segment findOptionalSegment(final String segmentName, final Message message) {
return findOptionalSegment(segmentName, 0, message);
}
public Segment findOptionalSegment(final String segmentName, int repetition, final Message message) {
try {
return new SegmentFinder(message).findSegment(segmentName, repetition);
} catch (HL7Exception hl7Ex) {
log.debug("Exception encountered while attempting to find {} segment repetition {} in {} message - returning null",
segmentName, repetition, message.getClass().getSimpleName());
return null;
}
}
public Segment findOptionalNonEmptySegment(final String segmentName, final Message message) {
return findOptionalNonEmptySegment(segmentName, 0, message);
}
public Segment findOptionalNonEmptySegment(final String segmentName, int repetition, final Message message) {
SegmentFinder segmentFinder = new SegmentFinder(message);
Segment segment;
try {
do {
segment = segmentFinder.findSegment(segmentName, repetition);
} while (segment != null && segment.isEmpty());
} catch (HL7Exception hl7Ex) {
log.debug("Exception encountered while attempting to find non-empty {} segment repetition {} in {} message - returning null",
segmentName, repetition, message.getClass().getSimpleName());
return null;
}
return segment;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy