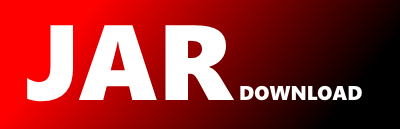
com.pronoia.hapi.hamcrest.MessageMatchers Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hapi-junit Show documentation
Show all versions of hapi-junit Show documentation
A library of JUnit assertions to assist in testing with HAPI messages.
/**
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.pronoia.hapi.hamcrest;
import java.util.regex.Pattern;
import ca.uhn.hl7v2.model.Message;
import ca.uhn.hl7v2.model.Segment;
import com.pronoia.hapi.hamcrest.message.MessageContainsPattern;
import com.pronoia.hapi.hamcrest.message.MessageContainsSegment;
import com.pronoia.hapi.hamcrest.message.MessageIsEqual;
import com.pronoia.hapi.hamcrest.message.MessageMatchesPattern;
import com.pronoia.hapi.hamcrest.message.MessageSegmentContains;
import com.pronoia.hapi.hamcrest.message.MessageSegmentEndsWith;
import com.pronoia.hapi.hamcrest.message.MessageSegmentMatchesPattern;
import com.pronoia.hapi.hamcrest.message.MessageSegmentStartsWith;
public final class MessageMatchers {
private MessageMatchers() {
}
public static MessageIsEqual equalTo(Message expected) {
return equalTo(expected, false);
}
public static MessageIsEqual equalTo(Message expected, boolean ignoreMshTimestamp) {
return new MessageIsEqual(expected, ignoreMshTimestamp);
}
public static MessageIsEqual equalTo(String[] expected) {
return equalTo(expected, false);
}
public static MessageIsEqual equalTo(String[] expected, boolean ignoreMshTimestamp) {
return new MessageIsEqual(expected, ignoreMshTimestamp);
}
public static MessageIsEqual equalTo(String expected) {
return equalTo(expected, false);
}
public static MessageIsEqual equalTo(String expected, boolean ignoreMshTimestamp) {
return new MessageIsEqual(expected, ignoreMshTimestamp);
}
public static MessageContainsPattern containsPattern(String expected) {
return new MessageContainsPattern(expected);
}
public static MessageContainsPattern containsPattern(Pattern expected) {
return new MessageContainsPattern(expected);
}
public static MessageMatchesPattern matchesPattern(String expected) {
return new MessageMatchesPattern(expected);
}
public static MessageMatchesPattern matchesPattern(Pattern expected) {
return new MessageMatchesPattern(expected);
}
public static MessageContainsSegment containsSegment(Segment expected) {
return containsSegment(expected, false);
}
public static MessageContainsSegment containsSegment(Segment expected, boolean ignoreMshTimestamp) {
return new MessageContainsSegment(expected, ignoreMshTimestamp);
}
public static MessageContainsSegment containsSegment(String expected) {
return containsSegment(expected, false);
}
public static MessageContainsSegment containsSegment(String expected, boolean ignoreMshTimestamp) {
return new MessageContainsSegment(expected, ignoreMshTimestamp);
}
public static MessageSegmentStartsWith containsSegmentStartingWith(String expected) {
return new MessageSegmentStartsWith(expected);
}
public static MessageSegmentEndsWith containsSegmentEndingWith(String name, String expected) {
return new MessageSegmentEndsWith(name, expected);
}
public static MessageSegmentContains containsSegmentContaining(String name, String expected) {
return new MessageSegmentContains(name, expected);
}
public static MessageSegmentMatchesPattern containsSegmentMatchingPattern(String name, String expected) {
return new MessageSegmentMatchesPattern(name, expected);
}
public static MessageSegmentMatchesPattern containsSegmentMatchingPattern(String name, Pattern expected) {
return new MessageSegmentMatchesPattern(name, expected);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy