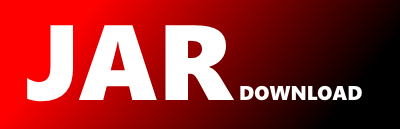
com.pronoia.hapi.hamcrest.message.MessageIsEqual Maven / Gradle / Ivy
/**
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.pronoia.hapi.hamcrest.message;
import ca.uhn.hl7v2.model.Message;
import com.pronoia.hapi.HapiTestUtil;
import org.hamcrest.Description;
import org.hamcrest.TypeSafeDiagnosingMatcher;
public class MessageIsEqual extends TypeSafeDiagnosingMatcher {
final boolean ignoreMshTimestamp;
final String[] expected;
int mismatchedSegmentIndex = -1;
public MessageIsEqual(Message expected, boolean ignoreMshTimestamp) {
this.ignoreMshTimestamp = ignoreMshTimestamp;
this.expected = HapiTestUtil.convertToArrayOfSegmentStrings(expected);
if (ignoreMshTimestamp) {
this.expected[0] = HapiTestUtil.stripMshTimestamp(this.expected[0]);
}
}
public MessageIsEqual(String[] expected, boolean ignoreMshTimestamp) {
this.ignoreMshTimestamp = ignoreMshTimestamp;
this.expected = expected;
if (ignoreMshTimestamp) {
this.expected[0] = HapiTestUtil.stripMshTimestamp(this.expected[0]);
}
}
public MessageIsEqual(String expected, boolean ignoreMshTimestamp) {
this.ignoreMshTimestamp = ignoreMshTimestamp;
this.expected = expected.split("\r");
if (ignoreMshTimestamp) {
this.expected[0] = HapiTestUtil.stripMshTimestamp(this.expected[0]);
}
}
@Override
protected boolean matchesSafely(Message actualMessage, Description mismatchDescription) {
String[] actual = HapiTestUtil.convertToArrayOfSegmentStrings(actualMessage);
if (ignoreMshTimestamp) {
actual[0] = HapiTestUtil.stripMshTimestamp(actual[0]);
}
if (expected.length != actual.length) {
mismatchDescription.appendText(actual.length + " Segments\n");
for (String segment : actual) {
mismatchDescription.appendText(segment)
.appendText("\n");
}
return false;
}
for (int segmentIndex = 0; segmentIndex < expected.length; ++segmentIndex) {
int indexOfDifference = HapiTestUtil.indexOfDifference(expected[segmentIndex], actual[segmentIndex]);
if (indexOfDifference >= 0) {
mismatchedSegmentIndex = segmentIndex;
mismatchDescription.appendText("Segment #")
.appendText(Integer.toString(segmentIndex + 1))
.appendText(" = ")
.appendValue(actual[segmentIndex])
.appendText("\n\tMismatch starting at index ")
.appendText(Integer.toString(indexOfDifference))
.appendText(" (zero-based)");
return false;
}
}
return true;
}
@Override
public void describeTo(Description description) {
if (mismatchedSegmentIndex >= 0) {
description.appendText("Segment #")
.appendText(Integer.toString(mismatchedSegmentIndex + 1))
.appendText(" = ")
.appendValue(expected[mismatchedSegmentIndex]);
} else {
description.appendText(expected.length + " Segments\n");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy