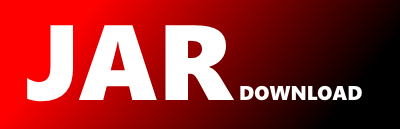
com.pronoia.hapi.hamcrest.terser.AbstractTerserSpecificationValueMatcher Maven / Gradle / Ivy
/**
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.pronoia.hapi.hamcrest.terser;
import ca.uhn.hl7v2.DefaultHapiContext;
import ca.uhn.hl7v2.HL7Exception;
import ca.uhn.hl7v2.model.GenericMessage;
import ca.uhn.hl7v2.model.Message;
import ca.uhn.hl7v2.util.Terser;
import com.pronoia.hapi.HapiTestException;
import org.hamcrest.Description;
import org.hamcrest.TypeSafeDiagnosingMatcher;
import static com.pronoia.hapi.HapiTestUtil.createGenericHapiContext;
import static com.pronoia.hapi.HapiTestUtil.encode;
public abstract class AbstractTerserSpecificationValueMatcher extends TypeSafeDiagnosingMatcher {
static final DefaultHapiContext GENERIC_HAPI_CONTEXT = createGenericHapiContext();
final boolean useGenericMessage;
final String expected;
final String spec;
public AbstractTerserSpecificationValueMatcher(String spec, boolean useGenericMessage, String expected) {
this.useGenericMessage = useGenericMessage;
this.expected = expected;
this.spec = spec;
}
public AbstractTerserSpecificationValueMatcher(String spec, boolean useGenericMessage, Terser expectedTerser) {
this.useGenericMessage = useGenericMessage;
this.spec = spec;
try {
this.expected = convert(expectedTerser).get(spec);
} catch (HL7Exception hapiEx) {
try {
Message message = expectedTerser.getSegment("MSH").getMessage();
throw new HapiTestException("Exception encountered extracting expected value for spec '" + spec + "' from Terser(" + message.getClass().getName() + ")", hapiEx);
} catch (HL7Exception determineMessageEx) {
throw new HapiTestException("Exception encountered extracting expected value for spec '" + spec + "' from Terser", hapiEx);
}
}
}
protected abstract boolean doMatches(String actual);
@Override
protected boolean matchesSafely(Terser actualTerser, Description mismatchDescription) {
String actual = null;
try {
actual = convert(actualTerser).get(spec);
if (!doMatches(actual)) {
mismatchDescription.appendText(spec)
.appendText(" = ")
.appendValue(actual);
return false;
}
} catch (HL7Exception hapiEx) {
mismatchDescription
.appendText("Exception encountered extracting value for '")
.appendText(spec)
.appendText("' spec from Terser");
try {
Message message = actualTerser.getSegment("MSH").getMessage();
mismatchDescription.appendText("(")
.appendText(message.getClass().getName())
.appendText(")");
} catch (HL7Exception determineMessageEx) {
// eat this
}
mismatchDescription.appendText(" ")
.appendValue(hapiEx);
return false;
}
return true;
}
Terser convert(Terser terser) {
try {
if (useGenericMessage) {
Message expectedMessage = terser.getSegment("MSH").getMessage();
if (!(expectedMessage instanceof GenericMessage)) {
Message genericMessage = GENERIC_HAPI_CONTEXT.getPipeParser().parse(encode(expectedMessage));
return new Terser(genericMessage);
}
}
} catch (HL7Exception hapiEx) {
throw new HapiTestException("HAPI Exception encountered converting to GenericMessage", hapiEx);
}
return terser;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy