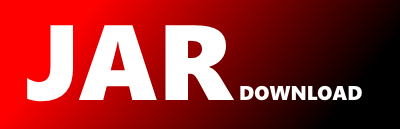
com.pronoia.splunk.eventcollector.EventDeliveryHttpException Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of splunk.httpec Show documentation
Show all versions of splunk.httpec Show documentation
A library to assist in building property-formatted JSON payloads for Splunk Events, and delivering those events to a Splunk HTTP
Event Collector
The newest version!
package com.pronoia.splunk.eventcollector;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.util.EntityUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class EventDeliveryHttpException extends EventDeliveryException {
static final Logger LOG = LoggerFactory.getLogger(EventDeliveryHttpException.class);
final int httpStatusCode;
final String httpReasonPhrase;
final Map splunkResponse;
public EventDeliveryHttpException(String event, HttpResponse response, String responseBody) {
super(event, String.format("Post failed with response %s", responseBody));
this.httpStatusCode = response.getStatusLine().getStatusCode();
this.httpReasonPhrase = response.getStatusLine().getReasonPhrase();
this.splunkResponse = parseResponseBody(responseBody);
}
public EventDeliveryHttpException(String event, HttpResponse response) {
this(event, response, extractResponseBody(response));
}
public int getHttpStatusCode() {
return httpStatusCode;
}
public String getHttpReasonPhrase() {
return httpReasonPhrase;
}
/**
* Get the status code returned from Splunk.
*
* @return the status code extracted from the HTTP response, or -1 if the code could not be extracted.
*
* @see Splunk HTTP Event Collector Documentation
*/
public int getSplunkStatusCode() {
int answer = -1;
if (splunkResponse.containsKey("code")) {
Object codeObj = splunkResponse.get("code");
try {
answer = Integer.parseInt(codeObj.toString());
} catch (Exception parseEx) {
LOG.warn("Failed to parse integer from %s - returning %d", codeObj, answer);
}
}
return answer;
}
public String getSplunkStatusMessage() {
String answer = "";
if (splunkResponse.containsKey("text")) {
Object textObj = splunkResponse.get("text");
if (textObj != null) {
answer = textObj.toString();
}
}
return answer;
}
static String extractResponseBody(HttpResponse response) {
String responseBody;
if (response == null) {
responseBody = "";
} else {
HttpEntity responseEntity = response.getEntity();
if (responseEntity == null) {
responseBody = "";
} else {
try {
responseBody = EntityUtils.toString(responseEntity);
if (responseBody == null) {
responseBody = "";
} else if (responseBody.isEmpty()) {
responseBody = "";
}
} catch (IOException entityEx) {
responseBody = String.format("", entityEx.getClass().getSimpleName(), entityEx.getMessage());
}
}
}
return responseBody;
}
static Map parseResponseBody(String responseBody) {
Map answer;
ObjectMapper mapper = new ObjectMapper();
try {
answer = mapper.readValue(responseBody, new TypeReference
© 2015 - 2025 Weber Informatics LLC | Privacy Policy