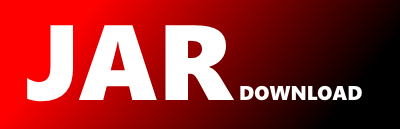
util.collection.ListMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xfvrp Show documentation
Show all versions of xfvrp Show documentation
Solver for realistic vehicle routing problems
package util.collection;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Set;
import util.Copyable;
/**
* Copyright (c) 2012-present Holger Schneider
* All rights reserved.
*
* This source code is licensed under the MIT License (MIT) found in the
* LICENSE file in the root directory of this source tree.
*
*
* @author hschneid
*
*/
public class ListMap implements Copyable> {
private static final int DEFAULT_LIST_SIZE = 10;
private HashMap> map;
private ListMap() {
map = new HashMap<>();
}
private ListMap(int size) {
map = new HashMap<>(size);
}
public static ListMap create() {
return new ListMap<>();
}
public static ListMap create(int size) {
return new ListMap<>(size);
}
public void put(K key, E element) {
List list = map.get(key);
if(list != null) {
map.get(key).add(element);
} else {
list = createList(DEFAULT_LIST_SIZE);
list.add(element);
map.put(key, list);
}
}
public void putAll(K key, List list) {
if(map.containsKey(key))
map.get(key).addAll(list);
else
map.put(key, list);
}
public void remove(K key) {
map.remove(key);
}
public void remove(K key, E element) {
if(map.containsKey(key))
map.get(key).remove(element);
}
public List get(K key) {
return map.get(key);
}
public boolean containsKey(K key) {
return map.containsKey(key);
}
public Set keySet() {
return map.keySet();
}
public Collection> values() {
return map.values();
}
public int size() {
return map.size();
}
public void clear() {
map.clear();
}
public Iterator iterator(K key) {
if(map.containsKey(key))
return map.get(key).iterator();
return null;
}
/*
* (non-Javadoc)
* @see da.util.Copyable#copy()
*/
@Override
public ListMap copy() {
ListMap copy = new ListMap<>(size());
for (K key : keySet()) {
List list = get(key);
if(list != null) {
List newList = createList(list.size());
newList.addAll(list);
copy.putAll(key, newList);
} else {
copy.put(key, null);
}
}
return copy;
}
private List createList(int size) {
return new ArrayList<>(size);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy