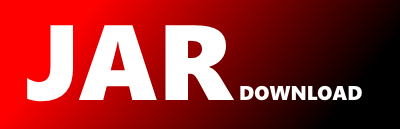
com.github.htfv.maven.plugins.testing.internal.MavenTestClassLoader Maven / Gradle / Ivy
package com.github.htfv.maven.plugins.testing.internal;
import java.io.File;
import java.io.IOException;
import java.net.URL;
import java.util.ArrayList;
import java.util.Enumeration;
import java.util.List;
/**
* {@link ClassLoader} implementation which hides resources from the workspace
* artifacts. This is mainly required to remove
* {@code META-INF/plexus/components.xml} from the system and core class
* loaders. If it is not done, the extensions may be executed twice: first from
* the core class loader, then from the plugin class loader.
*
* @author htfv (Aliaksei Lahachou)
*/
public class MavenTestClassLoader extends ClassLoader
{
/**
* Build a list of URLs under which all resources are hidden.
*
* @return The list of URLs.
*/
private static List getHiddenResourceURIs()
{
List hiddenResourceURIs = new ArrayList();
hiddenResourceURIs.add(new File("").toURI().toString());
return hiddenResourceURIs;
}
/**
* The list of URLs under which all resources are hidden.
*/
private final List hiddenResourceURIs;
/**
* Constructs a new instance of {@link MavenTestClassLoader}.
*
* @param parentClassLoader
* the parent {@link ClassLoader}.
*/
public MavenTestClassLoader(final ClassLoader parentClassLoader)
{
super(parentClassLoader);
//
// Build the list of URLs now, because later it may cause recursive
// class loading.
//
this.hiddenResourceURIs = getHiddenResourceURIs();
}
@Override
public Enumeration getResources(final String name) throws IOException
{
Enumeration resources = super.getResources(name);
resources = new AbstractFilteredEnumeration(resources)
{
@Override
protected boolean shouldIncludeElement(final URL element)
{
for (String hiddenResourceURI : hiddenResourceURIs)
{
if (element.toString().startsWith(hiddenResourceURI))
{
return false;
}
}
return true;
}
};
return resources;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy