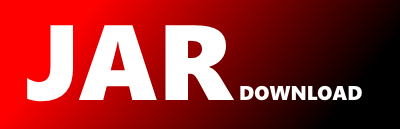
termo.equilibrium.PhaseEquilibria Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of materia Show documentation
Show all versions of materia Show documentation
Thermodynamics properties and
equilibria calculations for
chemical engineering.
//package termo.equilibrium;
//
//import java.util.ArrayList;
//import java.util.HashMap;
//import termo.binaryParameter.BinaryInteractionParameter;
//import termo.component.Component;
//import termo.eos.Cubic;
//
///**
// *
// * @author Hugo Redon Rivera
// */
//public abstract class PhaseEquilibria {
// public abstract EquilibriaPhaseSolution getTemperature(
// double temperatureEstimate,
// double pressureEstimate,
// ArrayList components,
// HashMap mixtureFractionsZ,
// HashMap estimationFractions,
// Cubic eos,
// BinaryInteractionParameter kinteraction,
// double tolerance);
//
// public EquilibriaPhaseSolution getTemperature(
// double pressure,
// HashMap mixtureFracions,
// ArrayList components,
// Cubic eos,
// BinaryInteractionParameter kinteraction,
// double tolerance
// ){
//
// EquilibriaPhaseSolution estimate = getTemperatureEstimate(pressure, mixtureFracions);
// return getTemperature(estimate, components, eos, kinteraction, tolerance);
// }
//
// public EquilibriaPhaseSolution getTemperature(
// EquilibriaPhaseSolution estimate,
// ArrayList components,
// Cubic eos,
// BinaryInteractionParameter kinteraction,
// double tolerance
// ){
// double temperature = estimate.getTemperature();
// double pressure =estimate.getPressure();
// HashMap liquidFractions = estimate.getMixtureFractions();
// HashMap vaporFractions = estimate.getSolutionFractions();
//
//
// return getTemperature(temperature, pressure, components, liquidFractions, vaporFractions, eos, kinteraction, tolerance);
// }
//
// public abstract EquilibriaPhaseSolution getPressure(double temperature,
// double pressure,
// ArrayList components,
// HashMap mixtureFractionsZ,
// HashMap estimationFractions,
// Cubic eos,
// BinaryInteractionParameter kinteraction,
// double tolerance);
//
// private EquilibriaPhaseSolution getPressure(double temperature,
// HashMap mixtureFractions,
// ArrayList components2,
// Cubic cubic,
// BinaryInteractionParameter kinteraction,
// double tol) {
//
// EquilibriaPhaseSolution estimate = getPressureEstimate(temperature, mixtureFractions);
// return getPressure(temperature, estimate, components2, cubic, kinteraction, tol);
// }
//
//
// private EquilibriaPhaseSolution getPressure(double temperature,
// EquilibriaPhaseSolution estimate,
// ArrayList components,
// Cubic eos,
// BinaryInteractionParameter kinteraction,
// double tolerance
// ){
// double pressure = estimate.getPressure();
// HashMap mixtureFractions = estimate.getMixtureFractions();
// HashMap solutionFractions = estimate.getSolutionFractions();
//
// return getPressure(temperature, pressure, components, mixtureFractions, solutionFractions, eos, kinteraction, tolerance);
// }
//
// public abstract EquilibriaPhaseSolution getPressureEstimate(
// double temperature,
// HashMap mixtureFractionsZ
// );
// public abstract EquilibriaPhaseSolution getTemperatureEstimate(
// double pressure,
// HashMap mixtureFractionsZ
// );
//
//
// public ArrayList getTemperatureDiagram(
// double pressure,
// Component component1,
// Component component2,
// BinaryInteractionParameter kinteraction,
// Cubic eos,
// double tolerance) {
// return getTemperatureDiagram(pressure, component1,component2, kinteraction, eos,101, tolerance);
//
//}
////Todo getTemperatureDiagram getPresureDiagram seems to be repetitive
//
// public ArrayList getTemperatureDiagram(
// double pressure,
// Component component1,
// Component component2,
// BinaryInteractionParameter kinteraction,
// Cubic eos,
// int numberCalculations,
// double tolerance) {
// ArrayList components2 = new ArrayList<>();
// components2.add(component1);
// components2.add(component2);
//
//
// ArrayList result = new ArrayList<>();
//
// double step = 1d / numberCalculations;
//
// for(double i= 0; i <= 1; i += step){
// HashMap mixtureFracions = new HashMap<>();
// mixtureFracions.put(component1, i);
// mixtureFracions.put(component2, 1-i);
//
// result.add(getTemperature(pressure, mixtureFracions, components2,eos , kinteraction, tolerance));
//
// }
//
// return result;
//
// }
//
// public ArrayList getPressureDiagram(double temperature,
// Component component1,
// Component component2,
// BinaryInteractionParameter kinteraction,
// Cubic cubic,
// int numberCalculations,
// double tol
// ) {
// ArrayList components2 = new ArrayList<>();
// components2.add(component1);
// components2.add(component2);
//
//
// ArrayList result = new ArrayList<>();
//
// double step = 1d / numberCalculations;
//
// for(double i= 0; i <= 1; i += step){
// HashMap solutionFractions = new HashMap<>();
// solutionFractions.put(component1, i);
// solutionFractions.put(component2, 1-i);
//
// result.add(getPressure(temperature, solutionFractions, components2,cubic , kinteraction, tol));
//
// }
// return result;
// }
// public ArrayList getPressureDiagram(double temperature,
// Component component1,
// Component component2,
// BinaryInteractionParameter kinteraction,
// Cubic cubic,
// double tol
// ) {
// return getPressureDiagram(temperature, component1, component2, kinteraction, cubic, 101, tol);
// }
//
//
//
// public ArrayList getPhaseEnvelope(
// ArrayList components,
// HashMap mixtureFractions,
// BinaryInteractionParameter kinteraction,
// Cubic cubic,
// double tol
// ){
// ArrayList phaseEnvelope = new ArrayList<>();
//
//
//
// return phaseEnvelope;
// }
//}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy