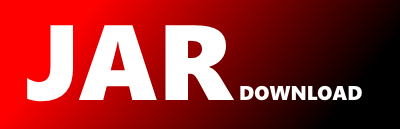
math.FastMath Maven / Gradle / Ivy
/*
* Copyright 2013 SPZ
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package math;
import java.util.Random;
/**
* The class {@code FastMath} contains methods for performing basic numeric
* operations such as the elementary exponential, logarithm, square root, and
* trigonometric functions.
*
* FastMath is a drop-in replacement for {@link Math}. This means that for any
* method in {@code java.lang.Math} (say {@code Math.sin(x)} or
* {@code Math.cbrt(y)}), user can directly change the class and use the methods
* as is (using {@code FastMath.sin(x)} or {@code FastMath.cbrt(y)} in the
* previous example).
*/
public final class FastMath {
/*
* Don't let anyone instantiate this class.
*/
private FastMath() {
throw new AssertionError();
}
/**
* Returns the trigonometric sine of an angle. Special cases:
*
* - If the argument is NaN or an infinity, then the result is NaN.
*
- If the argument is zero, then the result is a zero with the same sign
* as the argument.
*
*
*
* The computed result must be within 1 ulp of the exact result. Results
* must be semi-monotonic.
*
* @param a
* an angle, in radians.
* @return the sine of the argument.
*/
public static double sin(double a) {
return JafamaFastMath.sin(a);
}
/**
* Returns the trigonometric cosine of an angle. Special cases:
*
* - If the argument is NaN or an infinity, then the result is NaN.
*
*
*
* The computed result must be within 1 ulp of the exact result. Results
* must be semi-monotonic.
*
* @param a
* an angle, in radians.
* @return the cosine of the argument.
*/
public static double cos(double a) {
return JafamaFastMath.cos(a);
}
/**
* Returns the trigonometric tangent of an angle. Special cases:
*
* - If the argument is NaN or an infinity, then the result is NaN.
*
- If the argument is zero, then the result is a zero with the same sign
* as the argument.
*
*
*
* The computed result must be within 1 ulp of the exact result. Results
* must be semi-monotonic.
*
* @param a
* an angle, in radians.
* @return the tangent of the argument.
*/
public static double tan(double a) {
// StrictMath tan() can sometimes be faster than the intrinsic from
// java.lang.Math
return StrictMath.tan(a);
// that's a bit inaccurate around PI / 2
// return sin(a) / cos(a);
}
/**
* Returns the arc sine of a value; the returned angle is in the range
* -pi/2 through pi/2. Special cases:
*
* - If the argument is NaN or its absolute value is greater than 1, then
* the result is NaN.
*
- If the argument is zero, then the result is a zero with the same sign
* as the argument.
*
*
*
* The computed result must be within 1 ulp of the exact result. Results
* must be semi-monotonic.
*
* @param a
* the value whose arc sine is to be returned.
* @return the arc sine of the argument.
*/
public static double asin(double a) {
return JafamaFastMath.asin(a);
}
/**
* Returns the arc cosine of a value; the returned angle is in the range 0.0
* through pi. Special case:
*
* - If the argument is NaN or its absolute value is greater than 1, then
* the result is NaN.
*
*
*
* The computed result must be within 1 ulp of the exact result. Results
* must be semi-monotonic.
*
* @param a
* the value whose arc cosine is to be returned.
* @return the arc cosine of the argument.
*/
public static double acos(double a) {
return JafamaFastMath.acos(a);
}
/**
* Returns the arc tangent of a value; the returned angle is in the range
* -pi/2 through pi/2. Special cases:
*
* - If the argument is NaN, then the result is NaN.
*
- If the argument is zero, then the result is a zero with the same sign
* as the argument.
*
*
*
* The computed result must be within 1 ulp of the exact result. Results
* must be semi-monotonic.
*
* @param a
* the value whose arc tangent is to be returned.
* @return the arc tangent of the argument.
*/
public static double atan(double a) {
return JafamaFastMath.atan(a);
}
/**
* Converts an angle measured in degrees to an approximately equivalent
* angle measured in radians. The conversion from degrees to radians is
* generally inexact.
*
* @param angdeg
* an angle, in degrees
* @return the measurement of the angle {@code angdeg} in radians.
* @since 1.2
*/
public static double toRadians(double angdeg) {
return Math.toRadians(angdeg);
}
/**
* Converts an angle measured in radians to an approximately equivalent
* angle measured in degrees. The conversion from radians to degrees is
* generally inexact; users should not expect
* {@code cos(toRadians(90.0))} to exactly equal {@code 0.0}.
*
* @param angrad
* an angle, in radians
* @return the measurement of the angle {@code angrad} in degrees.
* @since 1.2
*/
public static double toDegrees(double angrad) {
return Math.toDegrees(angrad);
}
/**
* Returns Euler's number e raised to the power of a {@code double}
* value. Special cases:
*
* - If the argument is NaN, the result is NaN.
*
- If the argument is positive infinity, then the result is positive
* infinity.
*
- If the argument is negative infinity, then the result is positive
* zero.
*
*
*
* The computed result must be within 1 ulp of the exact result. Results
* must be semi-monotonic.
*
* @param a
* the exponent to raise e to.
* @return the value e{@code a}, where e is the
* base of the natural logarithms.
*/
public static double exp(double a) {
return CommonsAccurateMath.exp(a);
}
/**
* Returns the natural logarithm (base e) of a {@code double} value.
* Special cases:
*
* - If the argument is NaN or less than zero, then the result is NaN.
*
- If the argument is positive infinity, then the result is positive
* infinity.
*
- If the argument is positive zero or negative zero, then the result is
* negative infinity.
*
*
*
* The computed result must be within 1 ulp of the exact result. Results
* must be semi-monotonic.
*
* @param a
* a value
* @return the value ln {@code a}, the natural logarithm of {@code a}.
*/
public static double log(double a) {
// take advantage of the very good log() intrinsic for java.lang.Math
return Math.log(a);
}
/**
* Returns the base 10 logarithm of a {@code double} value. Special cases:
*
*
* - If the argument is NaN or less than zero, then the result is NaN.
*
- If the argument is positive infinity, then the result is positive
* infinity.
*
- If the argument is positive zero or negative zero, then the result is
* negative infinity.
*
- If the argument is equal to 10n for integer
* n, then the result is n.
*
*
*
* The computed result must be within 1 ulp of the exact result. Results
* must be semi-monotonic.
*
* @param a
* a value
* @return the base 10 logarithm of {@code a}.
* @since 1.5
*/
public static double log10(double a) {
// take advantage of the very good log10() intrinsic for java.lang.Math
return Math.log10(a);
}
/**
* Returns the correctly rounded positive square root of a {@code double}
* value. Special cases:
*
* - If the argument is NaN or less than zero, then the result is NaN.
*
- If the argument is positive infinity, then the result is positive
* infinity.
*
- If the argument is positive zero or negative zero, then the result is
* the same as the argument.
*
* Otherwise, the result is the {@code double} value closest to the true
* mathematical square root of the argument value.
*
* @param a
* a value.
* @return the positive square root of {@code a}. If the argument is NaN or
* less than zero, the result is NaN.
*/
public static double sqrt(double a) {
// it doesn't get any better than sqrt() from java.lang.Math
return Math.sqrt(a);
}
/**
* Returns the cube root of a {@code double} value. For positive finite
* {@code x}, {@code cbrt(-x) ==
* -cbrt(x)}; that is, the cube root of a negative value is the negative of
* the cube root of that value's magnitude.
*
* Special cases:
*
*
*
* - If the argument is NaN, then the result is NaN.
*
*
- If the argument is infinite, then the result is an infinity with the
* same sign as the argument.
*
*
- If the argument is zero, then the result is a zero with the same sign
* as the argument.
*
*
*
*
* The computed result must be within 1 ulp of the exact result.
*
* @param a
* a value.
* @return the cube root of {@code a}.
* @since 1.5
*/
public static double cbrt(double a) {
return CommonsAccurateMath.cbrt(a);
}
/**
* Computes the remainder operation on two arguments as prescribed by the
* IEEE 754 standard. The remainder value is mathematically equal to
* f1 - f2
× n, where n
* is the mathematical integer closest to the exact mathematical value of
* the quotient {@code f1/f2}, and if two mathematical integers are equally
* close to {@code f1/f2}, then n is the integer that is even. If the
* remainder is zero, its sign is the same as the sign of the first
* argument. Special cases:
*
* - If either argument is NaN, or the first argument is infinite, or the
* second argument is positive zero or negative zero, then the result is
* NaN.
*
- If the first argument is finite and the second argument is infinite,
* then the result is the same as the first argument.
*
*
* @param f1
* the dividend.
* @param f2
* the divisor.
* @return the remainder when {@code f1} is divided by {@code f2}.
*/
public static double IEEEremainder(double f1, double f2) {
return Math.IEEEremainder(f1, f2);
}
/**
* Returns the smallest (closest to negative infinity) {@code double} value
* that is greater than or equal to the argument and is equal to a
* mathematical integer. Special cases:
*
* - If the argument value is already equal to a mathematical integer,
* then the result is the same as the argument.
*
- If the argument is NaN or an infinity or positive zero or negative
* zero, then the result is the same as the argument.
*
- If the argument value is less than zero but greater than -1.0, then
* the result is negative zero.
*
* Note that the value of {@code Math.ceil(x)} is exactly the value of
* {@code -Math.floor(-x)}.
*
*
* @param a
* a value.
* @return the smallest (closest to negative infinity) floating-point value
* that is greater than or equal to the argument and is equal to a
* mathematical integer.
*/
public static double ceil(double a) {
return Math.ceil(a);
}
/**
* Returns the largest (closest to positive infinity) {@code double} value
* that is less than or equal to the argument and is equal to a mathematical
* integer. Special cases:
*
* - If the argument value is already equal to a mathematical integer,
* then the result is the same as the argument.
*
- If the argument is NaN or an infinity or positive zero or negative
* zero, then the result is the same as the argument.
*
*
* @param a
* a value.
* @return the largest (closest to positive infinity) floating-point value
* that less than or equal to the argument and is equal to a
* mathematical integer.
*/
public static double floor(double a) {
return Math.floor(a);
}
/**
* Returns the {@code double} value that is closest in value to the argument
* and is equal to a mathematical integer. If two {@code double} values that
* are mathematical integers are equally close, the result is the integer
* value that is even. Special cases:
*
* - If the argument value is already equal to a mathematical integer,
* then the result is the same as the argument.
*
- If the argument is NaN or an infinity or positive zero or negative
* zero, then the result is the same as the argument.
*
*
* @param a
* a {@code double} value.
* @return the closest floating-point value to {@code a} that is equal to a
* mathematical integer.
*/
public static double rint(double a) {
return Math.rint(a);
}
/**
* Returns the angle theta from the conversion of rectangular
* coordinates ({@code x}, {@code y}) to polar coordinates
* (r, theta). This method computes the phase theta by
* computing an arc tangent of {@code y/x} in the range of -pi to
* pi. Special cases:
*
* - If either argument is NaN, then the result is NaN.
*
- If the first argument is positive zero and the second argument is
* positive, or the first argument is positive and finite and the second
* argument is positive infinity, then the result is positive zero.
*
- If the first argument is negative zero and the second argument is
* positive, or the first argument is negative and finite and the second
* argument is positive infinity, then the result is negative zero.
*
- If the first argument is positive zero and the second argument is
* negative, or the first argument is positive and finite and the second
* argument is negative infinity, then the result is the {@code double}
* value closest to pi.
*
- If the first argument is negative zero and the second argument is
* negative, or the first argument is negative and finite and the second
* argument is negative infinity, then the result is the {@code double}
* value closest to -pi.
*
- If the first argument is positive and the second argument is positive
* zero or negative zero, or the first argument is positive infinity and the
* second argument is finite, then the result is the {@code double} value
* closest to pi/2.
*
- If the first argument is negative and the second argument is positive
* zero or negative zero, or the first argument is negative infinity and the
* second argument is finite, then the result is the {@code double} value
* closest to -pi/2.
*
- If both arguments are positive infinity, then the result is the
* {@code double} value closest to pi/4.
*
- If the first argument is positive infinity and the second argument is
* negative infinity, then the result is the {@code double} value closest to
* 3*pi/4.
*
- If the first argument is negative infinity and the second argument is
* positive infinity, then the result is the {@code double} value closest to
* -pi/4.
*
- If both arguments are negative infinity, then the result is the
* {@code double} value closest to -3*pi/4.
*
*
*
* The computed result must be within 2 ulps of the exact result. Results
* must be semi-monotonic.
*
* @param y
* the ordinate coordinate
* @param x
* the abscissa coordinate
* @return the theta component of the point
* (r, theta) in polar coordinates that
* corresponds to the point (x, y) in Cartesian
* coordinates.
*/
public static double atan2(double y, double x) {
return JafamaFastMath.atan2(y, x);
}
/**
* Returns the value of the first argument raised to the power of the second
* argument. Special cases:
*
*
* - If the second argument is positive or negative zero, then the result
* is 1.0.
*
- If the second argument is 1.0, then the result is the same as the
* first argument.
*
- If the second argument is NaN, then the result is NaN.
*
- If the first argument is NaN and the second argument is nonzero, then
* the result is NaN.
*
*
- If
*
* - the absolute value of the first argument is greater than 1 and the
* second argument is positive infinity, or
*
- the absolute value of the first argument is less than 1 and the
* second argument is negative infinity,
*
* then the result is positive infinity.
*
* - If
*
* - the absolute value of the first argument is greater than 1 and the
* second argument is negative infinity, or
*
- the absolute value of the first argument is less than 1 and the
* second argument is positive infinity,
*
* then the result is positive zero.
*
* - If the absolute value of the first argument equals 1 and the second
* argument is infinite, then the result is NaN.
*
*
- If
*
* - the first argument is positive zero and the second argument is
* greater than zero, or
*
- the first argument is positive infinity and the second argument is
* less than zero,
*
* then the result is positive zero.
*
* - If
*
* - the first argument is positive zero and the second argument is less
* than zero, or
*
- the first argument is positive infinity and the second argument is
* greater than zero,
*
* then the result is positive infinity.
*
* - If
*
* - the first argument is negative zero and the second argument is
* greater than zero but not a finite odd integer, or
*
- the first argument is negative infinity and the second argument is
* less than zero but not a finite odd integer,
*
* then the result is positive zero.
*
* - If
*
* - the first argument is negative zero and the second argument is a
* positive finite odd integer, or
*
- the first argument is negative infinity and the second argument is a
* negative finite odd integer,
*
* then the result is negative zero.
*
* - If
*
* - the first argument is negative zero and the second argument is less
* than zero but not a finite odd integer, or
*
- the first argument is negative infinity and the second argument is
* greater than zero but not a finite odd integer,
*
* then the result is positive infinity.
*
* - If
*
* - the first argument is negative zero and the second argument is a
* negative finite odd integer, or
*
- the first argument is negative infinity and the second argument is a
* positive finite odd integer,
*
* then the result is negative infinity.
*
* - If the first argument is finite and less than zero
*
* - if the second argument is a finite even integer, the result is equal
* to the result of raising the absolute value of the first argument to the
* power of the second argument
*
*
- if the second argument is a finite odd integer, the result is equal
* to the negative of the result of raising the absolute value of the first
* argument to the power of the second argument
*
*
- if the second argument is finite and not an integer, then the result
* is NaN.
*
*
* - If both arguments are integers, then the result is exactly equal to
* the mathematical result of raising the first argument to the power of the
* second argument if that result can in fact be represented exactly as a
* {@code double} value.
*
*
*
* (In the foregoing descriptions, a floating-point value is considered to
* be an integer if and only if it is finite and a fixed point of the method
* {@link #ceil ceil} or, equivalently, a fixed point of the method
* {@link #floor floor}. A value is a fixed point of a one-argument method
* if and only if the result of applying the method to the value is equal to
* the value.)
*
*
* The computed result must be within 1 ulp of the exact result. Results
* must be semi-monotonic.
*
* @param a
* the base.
* @param b
* the exponent.
* @return the value {@code a}{@code b}.
*/
public static double pow(double a, double b) {
return CommonsAccurateMath.pow(a, b);
}
/**
* Returns the closest {@code int} to the argument, with ties rounding up.
*
*
* Special cases:
*
* - If the argument is NaN, the result is 0.
*
- If the argument is negative infinity or any value less than or equal
* to the value of {@code Integer.MIN_VALUE}, the result is equal to the
* value of {@code Integer.MIN_VALUE}.
*
- If the argument is positive infinity or any value greater than or
* equal to the value of {@code Integer.MAX_VALUE}, the result is equal to
* the value of {@code Integer.MAX_VALUE}.
*
*
* @param a
* a floating-point value to be rounded to an integer.
* @return the value of the argument rounded to the nearest {@code int}
* value.
* @see java.lang.Integer#MAX_VALUE
* @see java.lang.Integer#MIN_VALUE
*/
public static int round(float a) {
return Math.round(a);
}
/**
* Returns the closest {@code long} to the argument, with ties rounding up.
*
*
* Special cases:
*
* - If the argument is NaN, the result is 0.
*
- If the argument is negative infinity or any value less than or equal
* to the value of {@code Long.MIN_VALUE}, the result is equal to the value
* of {@code Long.MIN_VALUE}.
*
- If the argument is positive infinity or any value greater than or
* equal to the value of {@code Long.MAX_VALUE}, the result is equal to the
* value of {@code Long.MAX_VALUE}.
*
*
* @param a
* a floating-point value to be rounded to a {@code long}.
* @return the value of the argument rounded to the nearest {@code long}
* value.
* @see java.lang.Long#MAX_VALUE
* @see java.lang.Long#MIN_VALUE
*/
public static long round(double a) {
return Math.round(a);
}
/**
* Returns a {@code double} value with a positive sign, greater than or
* equal to {@code 0.0} and less than {@code 1.0}. Returned values are
* chosen pseudorandomly with (approximately) uniform distribution from that
* range.
*
*
* This method delegates to {@link Math#random()}.
*
*
* This method is properly synchronized to allow correct use by more than
* one thread. However, if many threads need to generate pseudorandom
* numbers at a great rate, it may reduce contention for each thread to have
* its own pseudorandom-number generator.
*
* @return a pseudorandom {@code double} greater than or equal to
* {@code 0.0} and less than {@code 1.0}.
* @see Random#nextDouble()
*/
public static double random() {
return Math.random();
}
/**
* Returns the absolute value of an {@code int} value. If the argument is
* not negative, the argument is returned. If the argument is negative, the
* negation of the argument is returned.
*
*
* Note that if the argument is equal to the value of
* {@link Integer#MIN_VALUE}, the most negative representable {@code int}
* value, the result is that same value, which is negative.
*
* @param a
* the argument whose absolute value is to be determined
* @return the absolute value of the argument.
*/
public static int abs(int a) {
return (a ^ (a >> 31)) - (a >> 31);
}
/**
* Returns the absolute value of a {@code long} value. If the argument is
* not negative, the argument is returned. If the argument is negative, the
* negation of the argument is returned.
*
*
* Note that if the argument is equal to the value of {@link Long#MIN_VALUE}
* , the most negative representable {@code long} value, the result is that
* same value, which is negative.
*
* @param a
* the argument whose absolute value is to be determined
* @return the absolute value of the argument.
*/
public static long abs(long a) {
return (a ^ (a >> 63)) - (a >> 63);
}
/**
* Returns the absolute value of a {@code float} value. If the argument is
* not negative, the argument is returned. If the argument is negative, the
* negation of the argument is returned. Special cases:
*
* - If the argument is positive zero or negative zero, the result is
* positive zero.
*
- If the argument is infinite, the result is positive infinity.
*
- If the argument is NaN, the result is NaN.
*
* In other words, the result is the same as the value of the expression:
*
* {@code Float.intBitsToFloat(0x7fffffff & Float.floatToIntBits(a))}
*
* @param a
* the argument whose absolute value is to be determined
* @return the absolute value of the argument.
*/
public static float abs(float a) {
return Math.abs(a);
}
/**
* Returns the absolute value of a {@code double} value. If the argument is
* not negative, the argument is returned. If the argument is negative, the
* negation of the argument is returned. Special cases:
*
* - If the argument is positive zero or negative zero, the result is
* positive zero.
*
- If the argument is infinite, the result is positive infinity.
*
- If the argument is NaN, the result is NaN.
*
* In other words, the result is the same as the value of the expression:
*
* {@code Double.longBitsToDouble((Double.doubleToLongBits(a)<<1)>>>1)}
*
* @param a
* the argument whose absolute value is to be determined
* @return the absolute value of the argument.
*/
public static double abs(double a) {
return Math.abs(a);
}
/**
* Returns the greater of two {@code int} values. That is, the result is the
* argument closer to the value of {@link Integer#MAX_VALUE}. If the
* arguments have the same value, the result is that same value.
*
* @param a
* an argument.
* @param b
* another argument.
* @return the larger of {@code a} and {@code b}.
*/
public static int max(int a, int b) {
return Math.max(a, b);
}
/**
* Returns the greater of two {@code long} values. That is, the result is
* the argument closer to the value of {@link Long#MAX_VALUE}. If the
* arguments have the same value, the result is that same value.
*
* @param a
* an argument.
* @param b
* another argument.
* @return the larger of {@code a} and {@code b}.
*/
public static long max(long a, long b) {
return Math.max(a, b);
}
/**
* Returns the greater of two {@code float} values. That is, the result is
* the argument closer to positive infinity. If the arguments have the same
* value, the result is that same value. If either value is NaN, then the
* result is NaN. Unlike the numerical comparison operators, this method
* considers negative zero to be strictly smaller than positive zero. If one
* argument is positive zero and the other negative zero, the result is
* positive zero.
*
* @param a
* an argument.
* @param b
* another argument.
* @return the larger of {@code a} and {@code b}.
*/
public static float max(float a, float b) {
return Math.max(a, b);
}
/**
* Returns the greater of two {@code double} values. That is, the result is
* the argument closer to positive infinity. If the arguments have the same
* value, the result is that same value. If either value is NaN, then the
* result is NaN. Unlike the numerical comparison operators, this method
* considers negative zero to be strictly smaller than positive zero. If one
* argument is positive zero and the other negative zero, the result is
* positive zero.
*
* @param a
* an argument.
* @param b
* another argument.
* @return the larger of {@code a} and {@code b}.
*/
public static double max(double a, double b) {
return Math.max(a, b);
}
/**
* Returns the smaller of two {@code int} values. That is, the result the
* argument closer to the value of {@link Integer#MIN_VALUE}. If the
* arguments have the same value, the result is that same value.
*
* @param a
* an argument.
* @param b
* another argument.
* @return the smaller of {@code a} and {@code b}.
*/
public static int min(int a, int b) {
return Math.min(a, b);
}
/**
* Returns the smaller of two {@code long} values. That is, the result is
* the argument closer to the value of {@link Long#MIN_VALUE}. If the
* arguments have the same value, the result is that same value.
*
* @param a
* an argument.
* @param b
* another argument.
* @return the smaller of {@code a} and {@code b}.
*/
public static long min(long a, long b) {
return Math.min(a, b);
}
/**
* Returns the smaller of two {@code float} values. That is, the result is
* the value closer to negative infinity. If the arguments have the same
* value, the result is that same value. If either value is NaN, then the
* result is NaN. Unlike the numerical comparison operators, this method
* considers negative zero to be strictly smaller than positive zero. If one
* argument is positive zero and the other is negative zero, the result is
* negative zero.
*
* @param a
* an argument.
* @param b
* another argument.
* @return the smaller of {@code a} and {@code b}.
*/
public static float min(float a, float b) {
return Math.min(a, b);
}
/**
* Returns the smaller of two {@code double} values. That is, the result is
* the value closer to negative infinity. If the arguments have the same
* value, the result is that same value. If either value is NaN, then the
* result is NaN. Unlike the numerical comparison operators, this method
* considers negative zero to be strictly smaller than positive zero. If one
* argument is positive zero and the other is negative zero, the result is
* negative zero.
*
* @param a
* an argument.
* @param b
* another argument.
* @return the smaller of {@code a} and {@code b}.
*/
public static double min(double a, double b) {
return Math.min(a, b);
}
/**
* Returns the hyperbolic sine of a {@code double} value. The hyperbolic
* sine of x is defined to be
* (ex - e-x)/2 where e is
* {@linkplain Math#E Euler's number}.
*
*
* Special cases:
*
*
* - If the argument is NaN, then the result is NaN.
*
*
- If the argument is infinite, then the result is an infinity with the
* same sign as the argument.
*
*
- If the argument is zero, then the result is a zero with the same sign
* as the argument.
*
*
*
*
* The computed result must be within 2.5 ulps of the exact result.
*
* @param x
* The number whose hyperbolic sine is to be returned.
* @return The hyperbolic sine of {@code x}.
* @since 1.5
*/
public static double sinh(double x) {
return CommonsAccurateMath.sinh(x);
}
/**
* Returns the hyperbolic cosine of a {@code double} value. The hyperbolic
* cosine of x is defined to be
* (ex + e-x)/2 where e is
* {@linkplain Math#E Euler's number}.
*
*
* Special cases:
*
*
* - If the argument is NaN, then the result is NaN.
*
*
- If the argument is infinite, then the result is positive infinity.
*
*
- If the argument is zero, then the result is {@code 1.0}.
*
*
*
*
* The computed result must be within 2.5 ulps of the exact result.
*
* @param x
* The number whose hyperbolic cosine is to be returned.
* @return The hyperbolic cosine of {@code x}.
* @since 1.5
*/
public static double cosh(double x) {
return CommonsAccurateMath.cosh(x);
}
/**
* Returns the hyperbolic tangent of a {@code double} value. The hyperbolic
* tangent of x is defined to be
* (ex - e-
* x)/(ex + e-x), in other
* words, {@linkplain Math#sinh sinh(x)}/{@linkplain Math#cosh
* cosh(x)}. Note that the absolute value of the exact tanh is always
* less than 1.
*
*
* Special cases:
*
*
* - If the argument is NaN, then the result is NaN.
*
*
- If the argument is zero, then the result is a zero with the same sign
* as the argument.
*
*
- If the argument is positive infinity, then the result is {@code +1.0}.
*
*
- If the argument is negative infinity, then the result is {@code -1.0}.
*
*
*
*
* The computed result must be within 2.5 ulps of the exact result. The
* result of {@code tanh} for any finite input must have an absolute value
* less than or equal to 1. Note that once the exact result of tanh is
* within 1/2 of an ulp of the limit value of ±1, correctly signed
* ±{@code 1.0} should be returned.
*
* @param x
* The number whose hyperbolic tangent is to be returned.
* @return The hyperbolic tangent of {@code x}.
* @since 1.5
*/
public static double tanh(double x) {
return CommonsAccurateMath.tanh(x);
}
/**
* Returns sqrt(x2 +y2) without
* intermediate overflow or underflow.
*
*
* Special cases:
*
*
* - If either argument is infinite, then the result is positive infinity.
*
*
- If either argument is NaN and neither argument is infinite, then the
* result is NaN.
*
*
*
*
* The computed result must be within 1 ulp of the exact result. If one
* parameter is held constant, the results must be semi-monotonic in the
* other parameter.
*
* @param x
* a value
* @param y
* a value
* @return sqrt(x2 +y2) without
* intermediate overflow or underflow
* @since 1.5
*/
public static double hypot(double x, double y) {
return JafamaFastMath.hypot(x, y);
}
/**
* Returns ex -1. Note that for values of x
* near 0, the exact sum of {@code expm1(x)} + 1 is much closer to
* the true result of ex than {@code exp(x)}.
*
*
* Special cases:
*
* - If the argument is NaN, the result is NaN.
*
*
- If the argument is positive infinity, then the result is positive
* infinity.
*
*
- If the argument is negative infinity, then the result is -1.0.
*
*
- If the argument is zero, then the result is a zero with the same sign
* as the argument.
*
*
*
*
* The computed result must be within 1 ulp of the exact result. Results
* must be semi-monotonic. The result of {@code expm1} for any finite input
* must be greater than or equal to {@code -1.0}. Note that once the exact
* result of e{@code x} - 1 is within 1/2 ulp of
* the limit value -1, {@code -1.0} should be returned.
*
* @param x
* the exponent to raise e to in the computation of
* e{@code x} -1.
* @return the value e{@code x} - 1.
* @since 1.5
*/
public static double expm1(double x) {
return Math.expm1(x);
}
/**
* Returns the natural logarithm of the sum of the argument and 1. Note that
* for small values {@code x}, the result of {@code log1p(x)} is much closer
* to the true result of ln(1 + {@code x}) than the floating-point
* evaluation of {@code log(1.0+x)}.
*
*
* Special cases:
*
*
*
* - If the argument is NaN or less than -1, then the result is NaN.
*
*
- If the argument is positive infinity, then the result is positive
* infinity.
*
*
- If the argument is negative one, then the result is negative
* infinity.
*
*
- If the argument is zero, then the result is a zero with the same sign
* as the argument.
*
*
*
*
* The computed result must be within 1 ulp of the exact result. Results
* must be semi-monotonic.
*
* @param x
* a value
* @return the value ln({@code x} + 1), the natural log of
* {@code x} + 1
* @since 1.5
*/
public static double log1p(double x) {
return JafamaFastMath.log1p(x);
}
/**
* Returns the size of an ulp of the argument. An ulp of a {@code double}
* value is the positive distance between this floating-point value and the
* {@code double} value next larger in magnitude. Note that for non-NaN
* x, ulp(-x) == ulp(x)
.
*
*
* Special Cases:
*
* - If the argument is NaN, then the result is NaN.
*
- If the argument is positive or negative infinity, then the result is
* positive infinity.
*
- If the argument is positive or negative zero, then the result is
* {@code Double.MIN_VALUE}.
*
- If the argument is ±{@code Double.MAX_VALUE}, then the result
* is equal to 2971.
*
*
* @param d
* the floating-point value whose ulp is to be returned
* @return the size of an ulp of the argument
* @since 1.5
*/
public static double ulp(double d) {
return Math.ulp(d);
}
/**
* Returns the size of an ulp of the argument. An ulp of a {@code float}
* value is the positive distance between this floating-point value and the
* {@code float} value next larger in magnitude. Note that for non-NaN
* x, ulp(-x) == ulp(x)
.
*
*
* Special Cases:
*
* - If the argument is NaN, then the result is NaN.
*
- If the argument is positive or negative infinity, then the result is
* positive infinity.
*
- If the argument is positive or negative zero, then the result is
* {@code Float.MIN_VALUE}.
*
- If the argument is ±{@code Float.MAX_VALUE}, then the result
* is equal to 2104.
*
*
* @param f
* the floating-point value whose ulp is to be returned
* @return the size of an ulp of the argument
* @since 1.5
*/
public static float ulp(float f) {
return Math.ulp(f);
}
/**
* Returns the signum function of the argument; zero if the argument is
* zero, 1.0 if the argument is greater than zero, -1.0 if the argument is
* less than zero.
*
*
* Special Cases:
*
* - If the argument is NaN, then the result is NaN.
*
- If the argument is positive zero or negative zero, then the result is
* the same as the argument.
*
*
* @param d
* the floating-point value whose signum is to be returned
* @return the signum function of the argument
* @since 1.5
*/
public static double signum(double d) {
return Math.signum(d);
}
/**
* Returns the signum function of the argument; zero if the argument is
* zero, 1.0f if the argument is greater than zero, -1.0f if the argument is
* less than zero.
*
*
* Special Cases:
*
* - If the argument is NaN, then the result is NaN.
*
- If the argument is positive zero or negative zero, then the result is
* the same as the argument.
*
*
* @param f
* the floating-point value whose signum is to be returned
* @return the signum function of the argument
* @since 1.5
*/
public static float signum(float f) {
return Math.signum(f);
}
/**
* Returns the first floating-point argument with the sign of the second
* floating-point argument. Note that unlike the
* {@link StrictMath#copySign(double, double) StrictMath.copySign} method,
* this method does not require NaN {@code sign} arguments to be treated as
* positive values; implementations are permitted to treat some NaN
* arguments as positive and other NaN arguments as negative to allow
* greater performance.
*
* @param magnitude
* the parameter providing the magnitude of the result
* @param sign
* the parameter providing the sign of the result
* @return a value with the magnitude of {@code magnitude} and the sign of
* {@code sign}.
* @since 1.6
*/
public static double copySign(double magnitude, double sign) {
return Math.copySign(magnitude, sign);
}
/**
* Returns the first floating-point argument with the sign of the second
* floating-point argument. Note that unlike the
* {@link StrictMath#copySign(float, float) StrictMath.copySign} method,
* this method does not require NaN {@code sign} arguments to be treated as
* positive values; implementations are permitted to treat some NaN
* arguments as positive and other NaN arguments as negative to allow
* greater performance.
*
* @param magnitude
* the parameter providing the magnitude of the result
* @param sign
* the parameter providing the sign of the result
* @return a value with the magnitude of {@code magnitude} and the sign of
* {@code sign}.
* @since 1.6
*/
public static float copySign(float magnitude, float sign) {
return Math.copySign(magnitude, sign);
}
/**
* Returns the unbiased exponent used in the representation of a
* {@code float}. Special cases:
*
*
* - If the argument is NaN or infinite, then the result is
* {@link Float#MAX_EXPONENT} + 1.
*
- If the argument is zero or subnormal, then the result is
* {@link Float#MIN_EXPONENT} -1.
*
*
* @param f
* a {@code float} value
* @return the unbiased exponent of the argument
* @since 1.6
*/
public static int getExponent(float f) {
return Math.getExponent(f);
}
/**
* Returns the unbiased exponent used in the representation of a
* {@code double}. Special cases:
*
*
* - If the argument is NaN or infinite, then the result is
* {@link Double#MAX_EXPONENT} + 1.
*
- If the argument is zero or subnormal, then the result is
* {@link Double#MIN_EXPONENT} -1.
*
*
* @param d
* a {@code double} value
* @return the unbiased exponent of the argument
* @since 1.6
*/
public static int getExponent(double d) {
return Math.getExponent(d);
}
/**
* Returns the floating-point number adjacent to the first argument in the
* direction of the second argument. If both arguments compare as equal the
* second argument is returned.
*
*
* Special cases:
*
* - If either argument is a NaN, then NaN is returned.
*
*
- If both arguments are signed zeros, {@code direction} is returned
* unchanged (as implied by the requirement of returning the second argument
* if the arguments compare as equal).
*
*
- If {@code start} is ±{@link Double#MIN_VALUE} and
* {@code direction} has a value such that the result should have a smaller
* magnitude, then a zero with the same sign as {@code start} is returned.
*
*
- If {@code start} is infinite and {@code direction} has a value such
* that the result should have a smaller magnitude, {@link Double#MAX_VALUE}
* with the same sign as {@code start} is returned.
*
*
- If {@code start} is equal to ± {@link Double#MAX_VALUE} and
* {@code direction} has a value such that the result should have a larger
* magnitude, an infinity with same sign as {@code start} is returned.
*
*
* @param start
* starting floating-point value
* @param direction
* value indicating which of {@code start}'s neighbors or
* {@code start} should be returned
* @return The floating-point number adjacent to {@code start} in the
* direction of {@code direction}.
* @since 1.6
*/
public static double nextAfter(double start, double direction) {
return Math.nextAfter(start, direction);
}
/**
* Returns the floating-point number adjacent to the first argument in the
* direction of the second argument. If both arguments compare as equal a
* value equivalent to the second argument is returned.
*
*
* Special cases:
*
* - If either argument is a NaN, then NaN is returned.
*
*
- If both arguments are signed zeros, a value equivalent to
* {@code direction} is returned.
*
*
- If {@code start} is ±{@link Float#MIN_VALUE} and
* {@code direction} has a value such that the result should have a smaller
* magnitude, then a zero with the same sign as {@code start} is returned.
*
*
- If {@code start} is infinite and {@code direction} has a value such
* that the result should have a smaller magnitude, {@link Float#MAX_VALUE}
* with the same sign as {@code start} is returned.
*
*
- If {@code start} is equal to ± {@link Float#MAX_VALUE} and
* {@code direction} has a value such that the result should have a larger
* magnitude, an infinity with same sign as {@code start} is returned.
*
*
* @param start
* starting floating-point value
* @param direction
* value indicating which of {@code start}'s neighbors or
* {@code start} should be returned
* @return The floating-point number adjacent to {@code start} in the
* direction of {@code direction}.
* @since 1.6
*/
public static float nextAfter(float start, double direction) {
return Math.nextAfter(start, direction);
}
/**
* Returns the floating-point value adjacent to {@code d} in the direction
* of positive infinity. This method is semantically equivalent to
* {@code nextAfter(d,
* Double.POSITIVE_INFINITY)}; however, a {@code nextUp} implementation may
* run faster than its equivalent {@code nextAfter} call.
*
*
* Special Cases:
*
* - If the argument is NaN, the result is NaN.
*
*
- If the argument is positive infinity, the result is positive
* infinity.
*
*
- If the argument is zero, the result is {@link Double#MIN_VALUE}
*
*
*
* @param d
* starting floating-point value
* @return The adjacent floating-point value closer to positive infinity.
* @since 1.6
*/
public static double nextUp(double d) {
return Math.nextUp(d);
}
/**
* Returns the floating-point value adjacent to {@code f} in the direction
* of positive infinity. This method is semantically equivalent to
* {@code nextAfter(f,
* Float.POSITIVE_INFINITY)}; however, a {@code nextUp} implementation may
* run faster than its equivalent {@code nextAfter} call.
*
*
* Special Cases:
*
* - If the argument is NaN, the result is NaN.
*
*
- If the argument is positive infinity, the result is positive
* infinity.
*
*
- If the argument is zero, the result is {@link Float#MIN_VALUE}
*
*
*
* @param f
* starting floating-point value
* @return The adjacent floating-point value closer to positive infinity.
* @since 1.6
*/
public static float nextUp(float f) {
return Math.nextUp(f);
}
/**
* Return {@code d} × 2{@code scaleFactor} rounded as if
* performed by a single correctly rounded floating-point multiply to a
* member of the double value set. See the Java Language Specification for a
* discussion of floating-point value sets. If the exponent of the result is
* between {@link Double#MIN_EXPONENT} and {@link Double#MAX_EXPONENT}, the
* answer is calculated exactly. If the exponent of the result would be
* larger than {@code Double.MAX_EXPONENT}, an infinity is returned. Note
* that if the result is subnormal, precision may be lost; that is, when
* {@code scalb(x, n)} is subnormal, {@code scalb(scalb(x, n), -n)} may not
* equal x. When the result is non-NaN, the result has the same sign
* as {@code d}.
*
*
* Special cases:
*
* - If the first argument is NaN, NaN is returned.
*
- If the first argument is infinite, then an infinity of the same sign
* is returned.
*
- If the first argument is zero, then a zero of the same sign is
* returned.
*
*
* @param d
* number to be scaled by a power of two.
* @param scaleFactor
* power of 2 used to scale {@code d}
* @return {@code d} × 2{@code scaleFactor}
* @since 1.6
*/
public static double scalb(double d, int scaleFactor) {
return Math.scalb(d, scaleFactor);
}
/**
* Return {@code f} × 2{@code scaleFactor} rounded as if
* performed by a single correctly rounded floating-point multiply to a
* member of the float value set. See the Java Language Specification for a
* discussion of floating-point value sets. If the exponent of the result is
* between {@link Float#MIN_EXPONENT} and {@link Float#MAX_EXPONENT}, the
* answer is calculated exactly. If the exponent of the result would be
* larger than {@code Float.MAX_EXPONENT}, an infinity is returned. Note
* that if the result is subnormal, precision may be lost; that is, when
* {@code scalb(x, n)} is subnormal, {@code scalb(scalb(x, n), -n)} may not
* equal x. When the result is non-NaN, the result has the same sign
* as {@code f}.
*
*
* Special cases:
*
* - If the first argument is NaN, NaN is returned.
*
- If the first argument is infinite, then an infinity of the same sign
* is returned.
*
- If the first argument is zero, then a zero of the same sign is
* returned.
*
*
* @param f
* number to be scaled by a power of two.
* @param scaleFactor
* power of 2 used to scale {@code f}
* @return {@code f} × 2{@code scaleFactor}
* @since 1.6
*/
public static float scalb(float f, int scaleFactor) {
return Math.scalb(f, scaleFactor);
}
}