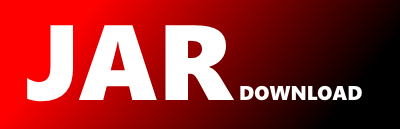
com.github.hypfvieh.config.xml.XmlConfigBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-utils-extended Show documentation
Show all versions of java-utils-extended Show documentation
A extended collection of utils commonly used in my projects.
This library will add additional features to java-utils library but requires third party code as well.
package com.github.hypfvieh.config.xml;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import org.xml.sax.ErrorHandler;
import com.github.hypfvieh.common.SearchOrder;
import com.github.hypfvieh.util.xml.XmlErrorHandlers.XmlErrorHandlerQuiet;
/**
* Builder for {@link XmlConfiguration}.
*
* @author hypfvieh
* @since v1.0.1 - 2018-01-15
*/
public class XmlConfigBuilder {
private InputStream inputStream;
private OutputStream outputStream;
private File outputFile;
private File inputFile;
private ErrorHandler errorHandler = new XmlErrorHandlerQuiet();
private String delimiterKey;
private boolean skipRoot;
private boolean allowOverride;
public XmlConfigBuilder() {
}
/**
* Set {@link InputStream} used to read configuration file.
*
* @param _inputStream
* @return this for chaining
*/
public XmlConfigBuilder setInputStream(InputStream _inputStream) {
if (inputFile != null) {
throw new ConfigurationException("Cannot set inputStream as inputFile is already set.");
}
inputStream = _inputStream;
return this;
}
/**
* Set {@link OutputStream} used to save configuration file.
* @param _outputStream
* @return this for chaining
*/
public XmlConfigBuilder setOutputStream(OutputStream _outputStream) {
if (outputFile != null) {
throw new ConfigurationException("Cannot set outputStream as outputFile is already set.");
}
outputStream = _outputStream;
return this;
}
/**
* Set output file used for saving.
* Note: This cannot be set if outputStream is already set.
*
* @param _outputFile
* @return this for chaining
*/
public XmlConfigBuilder setOutputFile(File _outputFile) {
if (outputStream != null) {
throw new ConfigurationException("Cannot set outputFile as outputStream is already set.");
}
outputFile = _outputFile;
return this;
}
/**
* Set output file used for saving.
* Note: This cannot be set if outputStream is already set.
*
* @param _outputFile
* @return this for chaining
*/
public XmlConfigBuilder setOutputFile(String _outputFile) {
return setOutputFile(new File(_outputFile));
}
/**
* Set input file used for reading configuration.
* Note: This cannot be set if inputStream is already set.
*
* @param _inputFile
* @return this for chaining
*/
public XmlConfigBuilder setInputFile(File _inputFile, SearchOrder... _order) {
if (inputStream != null) {
throw new ConfigurationException("Cannot set inputFile as inputStream is already set.");
}
if (_order == null || _order.length == 0) {
_order = new SearchOrder[] {SearchOrder.CUSTOM_PATH, SearchOrder.CLASS_PATH};
}
InputStream findFile = SearchOrder.findFile(_inputFile.toString(), _order);
inputFile = _inputFile;
inputStream = findFile;
return this;
}
/**
* Set input file used for reading configuration.
* Note: This cannot be set if inputStream is already set.
*
* @param _inputFile
* @return this for chaining
*/
public XmlConfigBuilder setInputFile(String _inputFile, SearchOrder... _order) {
return setInputFile(new File(_inputFile), _order);
}
/**
* Set the {@link ErrorHandler} used by the XML parser to handle XML errors.
* By default a 'quiet' handler is used (suppressing all errors).
* Use null here to disable validation.
*
* @param _xmlErrorHandler
* @return this for chaining
*/
public XmlConfigBuilder setXmlErrorHandler(ErrorHandler _xmlErrorHandler) {
errorHandler = _xmlErrorHandler;
return this;
}
/**
* Set the delimiter used to delimit XML nodes.
* By default "/" is used, which means all pathes have to be expressed like:
* foo/bar/baz
*
* @param _delimiterKey
* @return this for chaining
*/
public XmlConfigBuilder setKeyDelimiter(String _delimiterKey) {
delimiterKey = _delimiterKey;
return this;
}
/**
* Set this to true to ignore the first level of nodes inside the config.
* Usually each XML is starting with a single node (e.g. <Config>) and all
* keys are below that node.
* If this is set to true, you can use e.g. key/subKey instead of Config/key/subKey to address
* your values.
*
* Default is false.
*
* @param _skipRoot
* @return this for chaining
*/
public XmlConfigBuilder setSkipRoot(boolean _skipRoot) {
skipRoot = _skipRoot;
return this;
}
/**
* Set this to true to allow the user to override config parameters using system properties.
* This means, if key foo/bar is available in config and a environment variable of the same name
* exists, the value of the environment variable is used, instead of the value in config.
*
* This allows the user to easily override values by e.g. using -Dfoo/bar=value instead of editing the config file every time.
*
* Default is false
*
* @param _allowOverride
* @return this for chaining
*/
public XmlConfigBuilder setAllowKeyOverrideFromEnvironment(boolean _allowOverride) {
allowOverride = _allowOverride;
return this;
}
/**
* Build the {@link XmlConfiguration} and return it.
* Will create a new {@link XmlConfiguration} on each call.
* @return {@link XmlConfiguration}
*/
public XmlConfiguration build() {
XmlConfiguration xmlConfiguration = new XmlConfiguration(skipRoot);
xmlConfiguration.setKeyDelimiter(delimiterKey);
xmlConfiguration.setXmlErrorHandler(errorHandler);
xmlConfiguration.setAllowOverride(allowOverride);
xmlConfiguration.setInputStream(inputStream);
xmlConfiguration.setOutputStream(outputStream);
xmlConfiguration.setInputFile(inputFile);
xmlConfiguration.setOutputFile(outputFile);
try {
xmlConfiguration.readAndValidate();
} catch (IOException _ex) {
throw new ConfigurationException(_ex);
}
return xmlConfiguration;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy