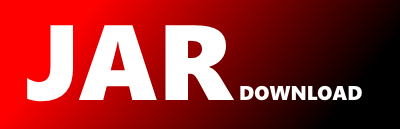
com.github.ibole.infrastructure.cache.redis.RedisSimpleTempalte Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of infrastructure-all Show documentation
Show all versions of infrastructure-all Show documentation
The all in one project of ibole infrastructure
The newest version!
package com.github.ibole.infrastructure.cache.redis;
import java.io.UnsupportedEncodingException;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Set;
import redis.clients.jedis.GeoCoordinate;
import redis.clients.jedis.GeoRadiusResponse;
import redis.clients.jedis.GeoUnit;
import redis.clients.jedis.Jedis;
import redis.clients.jedis.Pipeline;
/*********************************************************************************************
* .
*
*
*
* Copyright 2016, iBole Inc. All rights reserved.
*
*
.
*
*********************************************************************************************/
public class RedisSimpleTempalte extends RedisTemplate {
private String ip;
private int port;
private String password;
// key Prefix to distinguish different domain.
private String namespace = "default";
public RedisSimpleTempalte(String ip, int port, String password) {
this.ip = ip;
this.port = port;
this.password = password;
}
/**
* Constructor.
*
* @param ip IP
* @param port Port
* @param password Password
* @param namespace Name space to distinguish different domain
*/
public RedisSimpleTempalte(String ip, int port, String password, String namespace) {
this.ip = ip;
this.port = port;
this.namespace = namespace;
this.password = password;
}
private String buildKey(String pKey) {
return namespace + "." + pKey;
}
public void changeNamespace(String namespace) {
this.namespace = namespace;
}
public String select(int index) {
return execute(new RedisCallback() {
public String call(Jedis jedis, Object parms) {
return jedis.select(index);
}
}, index);
}
public Set keys(String pattern) {
return execute(new RedisCallback>() {
public Set call(Jedis jedis, Object parms) {
return jedis.keys(buildKey(pattern));
}
}, pattern);
}
public String hget(String key, String field) {
return execute(new RedisCallback() {
public String call(Jedis jedis, Object parms) {
return jedis.hget(buildKey(key), field);
}
}, key, field);
}
public void hset(String key, String field, String value) {
execute(new RedisCallback() {
public String call(Jedis jedis, Object parms) {
// String key = ((Object[]) parms)[0].toString();
// String field = ((Object[]) parms)[1].toString();
// String value = ((Object[]) parms)[2].toString();
jedis.hset(buildKey(key), field, value);
return null;
}
}, key, field, value);
}
public void expire(String key, int seconds) {
execute(new RedisCallback() {
public String call(Jedis jedis, Object parms) {
jedis.expire(buildKey(key), seconds);
return null;
}
}, key, seconds);
}
/**
* @param key String
* @return value String
*/
public String get(String key) {
return execute(new RedisCallback() {
public String call(Jedis jedis, Object parms) {
return jedis.get(buildKey(key));
}
}, key);
}
public String get(String key, LoadData load, boolean isUpdateCache) {
String value = get(key);
if (value == null || value.equals("")) {
value = load.loadDbData();
if ((value == null || "".equals(value)) && isUpdateCache) {
set(key, value);
}
}
return value;
}
public byte[] getByte(String key) {
return execute(new RedisCallback() {
public byte[] call(Jedis jedis, Object parms) {
try {
return jedis.get(buildKey(key).getBytes("UTF-8"));
} catch (UnsupportedEncodingException e) {
logger.error("Error happened when get byte[] by key {}", key, e);
}
return null;
}
}, key);
}
public byte[] getByte(String key, LoadData load, boolean isUpdateCache) {
return execute(new RedisCallback() {
public byte[] call(Jedis jedis, Object parms) {
try {
byte[] b = jedis.get(buildKey(key).getBytes("UTF-8"));
if (b == null || b.length == 0) {
b = load.loadDbData();
if ((b != null && b.length > 0) && isUpdateCache) {
jedis.set(buildKey(key).getBytes("UTF-8"), b);
}
}
return b;
} catch (UnsupportedEncodingException e) {
logger.error("Error happened when get byte[] by key {}", key, e);
}
return null;
}
}, key);
}
public void set(String key, String value) {
execute(new RedisCallback() {
public String call(Jedis jedis, Object parms) {
jedis.set(buildKey(key), value);
return null;
}
}, key, value);
}
public void set(String key, byte[] value) {
execute(new RedisCallback() {
public String call(Jedis jedis, Object parms) {
try {
jedis.set(buildKey(key).getBytes("UTF-8"), value);
} catch (UnsupportedEncodingException e) {
logger.error("Error happened when save byte[] with key {}", key, e);
}
return null;
}
}, key, value);
}
// seconds:过期时间(单位:秒)
public void set(String key, String value, int seconds) {
execute(new RedisCallback() {
public String call(Jedis jedis, Object parms) {
jedis.setex(buildKey(key), seconds, value);
return null;
}
}, key, value, seconds);
}
public void set(String key, byte[] value, int seconds) {
execute(new RedisCallback() {
public String call(Jedis jedis, Object parms) {
try {
jedis.setex(buildKey(key).getBytes("UTF-8"), seconds, value);
} catch (UnsupportedEncodingException e) {
logger.error("Error happened when save byte[] with key {} and expired time {}", key, seconds, e);
}
return null;
}
}, key, value, seconds);
}
public void del(String key) {
execute(new RedisCallback() {
public String call(Jedis jedis, Object parms) {
jedis.del(key);
return null;
}
}, key);
}
public Boolean exists(String key) {
return execute(new RedisCallback() {
public Boolean call(Jedis jedis, Object parms) {
return jedis.exists(buildKey(key));
}
}, key);
}
public String llen(String key) {
return execute(new RedisCallback() {
public String call(Jedis jedis, Object parms) {
return jedis.llen(buildKey(key)) + "";
}
}, key);
}
public void lpush(String key, String value) {
execute(new RedisCallback() {
public String call(Jedis jedis, Object parms) {
jedis.lpush(buildKey(key), value);
return null;
}
}, key, value);
}
public void lpush(String key, byte[] value) {
execute(new RedisCallback() {
public String call(Jedis jedis, Object parms) {
try {
jedis.lpush(buildKey(key).getBytes("UTF-8"), value);
} catch (UnsupportedEncodingException e) {
logger.error("Error happened when save byte[] with key {}", key, e);
}
return null;
}
}, key, value);
}
public void rpush(String key, String value) {
execute(new RedisCallback() {
public String call(Jedis jedis, Object parms) {
jedis.rpush(buildKey(key), value);
return null;
}
}, key, value);
}
public void rpush(String key, byte[] value) {
execute(new RedisCallback() {
public String call(Jedis jedis, Object parms) {
try {
jedis.rpush(buildKey(key).getBytes("UTF-8"), value);
} catch (UnsupportedEncodingException e) {
logger.error("Error happened when save byte[] with key {}", key, e);
}
return null;
}
}, key, value);
}
public void lpushPipeLine(String key, List values) {
execute(new RedisCallback() {
@SuppressWarnings("unchecked")
public String call(Jedis jedis, Object parms) {
Pipeline p = jedis.pipelined();
String buildKey = buildKey(key);
for (String value : values) {
p.lpush(buildKey, value);
}
p.sync();
return null;
}
}, key, values);
}
public void lpushPipeLineByte(String key, List values) {
execute(new RedisCallback() {
@SuppressWarnings("unchecked")
public byte[] call(Jedis jedis, Object parms) {
Pipeline p = jedis.pipelined();
try {
byte[] byteKey = buildKey(key).getBytes("UTF-8");
for (byte[] value : values) {
p.lpush(byteKey, value);
}
p.sync();
} catch (UnsupportedEncodingException e) {
logger.error("Error happened when save byte[] with key {}", key, e);
}
return null;
}
}, key, values);
}
public void rpushPipeLineByte(String key, List values) {
execute(new RedisCallback() {
@SuppressWarnings("unchecked")
public byte[] call(Jedis jedis, Object parms) {
Pipeline p = jedis.pipelined();
try {
byte[] byteKey = buildKey(key).getBytes("UTF-8");
for (byte[] value : values) {
p.rpush(byteKey, value);
}
p.sync();
} catch (UnsupportedEncodingException e) {
logger.error("Error happened when save byte[] with key {}", key, e);
}
return null;
}
}, key, values);
}
public void rpushPipeLine(String key, List values) {
execute(new RedisCallback() {
@SuppressWarnings("unchecked")
public String call(Jedis jedis, Object parms) {
Pipeline p = jedis.pipelined();
String buildKey = buildKey(key);
for (String value : values) {
p.rpush(buildKey, value);
}
p.sync();
return null;
}
}, key, values);
}
public List lrange(String key, long start, long end) {
return execute(new RedisCallback>() {
public List call(Jedis jedis, Object parms) {
return jedis.lrange(buildKey(key), start, end);
}
}, key, start, end);
}
public List lrangeByte(String key, long start, long end) {
return execute(new RedisCallback>() {
public List call(Jedis jedis, Object parms) {
try {
return jedis.lrange(buildKey(key).getBytes("UTF-8"), start, end);
} catch (UnsupportedEncodingException e) {
logger.error("Error happened when save byte[] with key {}", key, e);
}
return null;
}
}, key, start, end);
}
public List lrange(String key, long start, long end, LoadData load, boolean isCache) {
List list = lrange(key, start, end);
if ((list == null || list.isEmpty()) && load != null) {
list = load.loadDbData();
if (!list.isEmpty() && isCache)
lpushPipeLine(key, list);
}
return list;
}
public void incr(String key) {
execute(new RedisCallback() {
public String call(Jedis jedis, Object parms) {
jedis.incr(buildKey(key));
return null;
}
}, key);
}
public void sadd(String key, String value) {
execute(new RedisCallback() {
public String call(Jedis jedis, Object parms) {
jedis.sadd(buildKey(key), value);
return null;
}
}, key, value);
}
public void saddPipeLine(String key, Collection values) {
execute(new RedisCallback() {
public String call(Jedis jedis, Object parms) {
Pipeline p = jedis.pipelined();
String buildKey = buildKey(key);
for (String val : values) {
p.sadd(buildKey, val);
}
p.sync();
return null;
}
}, key, values);
}
public Set smembers(String key) {
return execute(new RedisCallback>() {
public Set call(Jedis jedis, Object parms) {
return jedis.smembers(buildKey(key));
}
}, key);
}
public Set sinter(String... keys) {
return execute(new RedisCallback>() {
public Set call(Jedis jedis, Object parms) {
return jedis.sinter(keys);
}
}, "");
}
public Long sinterstore(String dstkey, String... keys) {
return execute(new RedisCallback() {
public Long call(Jedis jedis, Object parms) {
return jedis.sinterstore(buildKey(dstkey), keys);
}
}, dstkey);
}
public List brpop(String key) {
return execute(new RedisCallback>() {
public List call(Jedis jedis, Object parms) {
return jedis.brpop(0, buildKey(key));
}
}, key);
}
public Long geoadd(String key, double longitude, double latitude, String member) {
return execute(new RedisCallback() {
public Long call(Jedis jedis, Object parms) {
return jedis.geoadd(buildKey(key), longitude, latitude, member);
}
}, key);
}
public void geoaddPipeLine(String key, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy