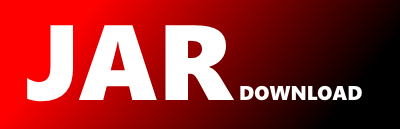
com.github.ibole.infrastructure.cache.session.redis.RedisHttpSession Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of infrastructure-all Show documentation
Show all versions of infrastructure-all Show documentation
The all in one project of ibole infrastructure
The newest version!
package com.github.ibole.infrastructure.cache.session.redis;
import java.io.Serializable;
import java.util.Enumeration;
import java.util.HashMap;
import java.util.Map;
import javax.servlet.ServletContext;
import javax.servlet.http.HttpSession;
import javax.servlet.http.HttpSessionContext;
/*********************************************************************************************
* .
*
*
* Copyright 2016, iBole Inc. All rights reserved.
*
*
*
*********************************************************************************************/
/**
* 实现HttpSession接口,替换原生的Session存取操作.
*
* @author bwang
*
*/
public class RedisHttpSession implements HttpSession, Serializable {
private static final long serialVersionUID = 1L;
protected long creationTime = 0L;
protected long lastAccessedTime = 0L;
protected String id;
protected int maxInactiveInterval;
/**
* Session过期标志.
*/
protected transient boolean expired;
/**
* Session新建标志.
*/
protected transient boolean isNew;
/**
* Session同步标志,用于标记本地进程中的缓存对象与远程Redis中是否一致.
*/
protected transient boolean isDirty;
private transient RedisSessionListener listener;
// 本地Session缓存,需要注意同远程Redis的数据同步.
private Map data;
/**
* Default constructor.
*/
public RedisHttpSession() {
this.expired = false;
this.isNew = false;
this.isDirty = false;
this.data = new HashMap();
}
public void setListener(RedisSessionListener listener) {
this.listener = listener;
}
public long getCreationTime() {
return this.creationTime;
}
public String getId() {
return this.id;
}
public long getLastAccessedTime() {
return this.lastAccessedTime;
}
public void setLastAccessedTime(long lastAccessedTime) {
this.lastAccessedTime = lastAccessedTime;
}
public ServletContext getServletContext() {
return null;
}
public void setMaxInactiveInterval(int i) {
this.maxInactiveInterval = i;
}
public int getMaxInactiveInterval() {
return this.maxInactiveInterval;
}
public HttpSessionContext getSessionContext() {
return null;
}
public Object getAttribute(String key) {
return this.data.get(key);
}
public Object getValue(String key) {
return this.data.get(key);
}
public String[] getValueNames() {
String[] names = new String[this.data.size()];
return ((String[]) this.data.keySet().toArray(names));
}
public void setAttribute(String s, Object o) {
this.data.put(s, o);
this.isDirty = true;
}
public void putValue(String s, Object o) {
this.data.put(s, o);
this.isDirty = true;
}
public void removeAttribute(String s) {
this.data.remove(s);
this.isDirty = true;
}
public void removeValue(String s) {
this.data.remove(s);
this.isDirty = true;
}
/**
* invalidate session.
*/
public void invalidate() {
this.expired = true;
this.isDirty = true;
if (this.listener != null) {
this.listener.onInvalidated(this);
}
}
public boolean isNew() {
return this.isNew;
}
public Enumeration getAttributeNames() {
return new SessionEnumeration(this.data);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy