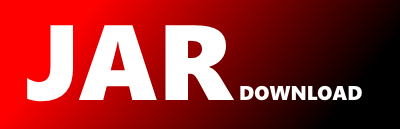
com.github.ibole.infrastructure.common.utils.Couple Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of infrastructure-all Show documentation
Show all versions of infrastructure-all Show documentation
The all in one project of ibole infrastructure
The newest version!
package com.github.ibole.infrastructure.common.utils;
import java.util.Objects;
import java.util.function.BiFunction;
import java.util.function.Function;
/*********************************************************************************************
* .
*
*
*
* Copyright 2016, iBole Inc. All rights reserved.
*
*
*
*********************************************************************************************/
/**
* Couple of Objects.
*
* @param Left Object Type
* @param Right Object Type
*/
public final class Couple {
/**
* Create a new empty Couple.
*
* @param Left Object Type
* @param Right Object Type
*
* @return A new empty Couple
*/
public static Couple empty() {
return new Couple<>(null, null);
}
/**
* Create a new Couple with only the left object set.
*
* @param Left Object Type
* @param Right Object Type
* @param left Left Object
*
* @return A new Couple with only the left object set
*/
public static Couple leftOnly(L left) {
return new Couple<>(left, null);
}
/**
* Create a new Couple with only the right object set.
*
* @param Left Object Type
* @param Right Object Type
* @param right Right Object
*
* @return A new Couple with only the right object set
*/
public static Couple rightOnly(R right) {
return new Couple<>(null, right);
}
/**
* Create a new Couple with both the left and right objects set.
*
* @param Left Object Type
* @param Right Object Type
* @param left Left Object
* @param right Right Object
*
* @return A new Couple with both the left and right objects set
*/
public static Couple of(L left, R right) {
return new Couple<>(left, right);
}
private final L left;
private final R right;
private Couple(L left, R right) {
this.left = left;
this.right = right;
}
/**
* @return Left Object or null if not set
*/
public L left() {
return left;
}
/**
* @return Right Object or null if not set
*/
public R right() {
return right;
}
/**
* @return {@literal true} if Left Object is not null, otherwise return {@literal false}
*/
public boolean hasLeft() {
return left != null;
}
/**
* @return {@literal true} if Right Object is not null, otherwise return {@literal false}
*/
public boolean hasRight() {
return right != null;
}
/**
* Create a new Couple with this Right Object and the given Left Object.
*
* @param Left Object Type
* @param left Left Object
*
* @return A new Couple with this Right Object and the given Left Object
*/
public Couple withLeft(T left) {
return new Couple<>(left, right);
}
/**
* Create a new Couple with this Left Object and the given Right Object.
*
* @param Right Object Type
* @param right Right Object
*
* @return A new Couple with this Left Object and the given Right Object
*/
public Couple withRight(T right) {
return new Couple<>(left, right);
}
/**
* Apply the given function to this Couple.
*
* @param Function Return Type
* @param function Function to apply
*
* @return The result of the function applied to this Couple.
*/
public T apply(Function, T> function) {
return function.apply(this);
}
/**
* Apply the given function to this Couple.
*
* @param Function Return Type
* @param function Function to apply
*
* @return The result of the function applied to this Couple.
*/
public T apply(BiFunction function) {
return function.apply(left, right);
}
@Override
public int hashCode() {
int hash = 5;
hash = 17 * hash + Objects.hashCode(this.left);
hash = 17 * hash + Objects.hashCode(this.right);
return hash;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (getClass() != obj.getClass()) {
return false;
}
final Couple, ?> other = (Couple, ?>) obj;
if (!Objects.equals(this.left, other.left)) {
return false;
}
return Objects.equals(this.right, other.right);
}
@Override
public String toString() {
return "Couple{" + "left=" + left + ", right=" + right + '}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy