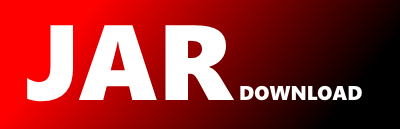
com.feilong.json.builder.SensitiveWordsPropertyNameAndJsonValueProcessorMapBuilder Maven / Gradle / Ivy
/*
* Copyright (C) 2008 feilong
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.feilong.json.builder;
import static com.feilong.core.Validator.isNullOrEmpty;
import static com.feilong.core.lang.ObjectUtil.equalsAny;
import static com.feilong.core.util.CollectionsUtil.addAllIgnoreNull;
import static com.feilong.core.util.CollectionsUtil.newArrayList;
import static com.feilong.core.util.MapUtil.newHashMap;
import static java.util.Collections.emptyList;
import static java.util.Collections.emptyMap;
import java.beans.PropertyDescriptor;
import java.lang.annotation.Annotation;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.feilong.core.Validate;
import com.feilong.core.bean.PropertyUtil;
import com.feilong.core.lang.ClassUtil;
import com.feilong.core.util.MapUtil;
import com.feilong.json.SensitiveWords;
import com.feilong.json.processor.SensitiveWordsJsonValueProcessor;
import com.feilong.lib.json.processors.JsonValueProcessor;
import com.feilong.lib.lang3.reflect.FieldUtils;
import com.feilong.lib.lang3.reflect.MethodUtils;
/**
* 专门用来构造 SensitiveWordsPropertyNameAndJsonValueProcessorMap
的构造器.
*
* @author feilong
* @since 1.11.5
*/
public class SensitiveWordsPropertyNameAndJsonValueProcessorMapBuilder{
/** The Constant log. */
private static final Logger LOGGER = LoggerFactory
.getLogger(SensitiveWordsPropertyNameAndJsonValueProcessorMapBuilder.class);
//---------------------------------------------------------------
/** The cache. */
private static Map, Map> cache = newHashMap();
//---------------------------------------------------------------
/** Don't let anyone instantiate this class. */
private SensitiveWordsPropertyNameAndJsonValueProcessorMapBuilder(){
//AssertionError不是必须的. 但它可以避免不小心在类的内部调用构造器. 保证该类在任何情况下都不会被实例化.
//see 《Effective Java》 2nd
throw new AssertionError("No " + getClass().getName() + " instances for you!");
}
//---------------------------------------------------------------
/**
* Builds the sensitive words property name and json value processor map.
*
* @param javaBean
* the java bean
* @return the map
* @since 1.10.3
*/
static Map build(Object javaBean){
Validate.notNull(javaBean, "javaBean can't be null!");
//---------------------------------------------------------------
if (ClassUtil.isInstance(javaBean, Map.class)){
return parseMap(javaBean);
}
if (ClassUtil.isInstance(javaBean, Iterable.class)){
return parseList(javaBean);
}
return parseBean(javaBean);
}
//---------------------------------------------------------------
/**
* Parses the list.
*
* @param inputMap
* the input map
* @return the map
* @since 1.13.0
*/
private static Map parseList(Object inputMap){
Map propertyNameAndJsonValueProcessorMap = newHashMap();
//---------------------------------------------------------------
Iterable> list = (Iterable>) inputMap;
for (Object bean : list){
if (null != bean){
MapUtil.putAllIfNotNull(propertyNameAndJsonValueProcessorMap, parseBean(bean));
}
}
//---------------------------------------------------------------
return propertyNameAndJsonValueProcessorMap;
}
//---------------------------------------------------------------
/**
* Parses the map.
*
* @param inputMap
* the input map
* @return the map
* @since 1.13.0
*/
private static Map parseMap(Object inputMap){
Map propertyNameAndJsonValueProcessorMap = newHashMap();
//---------------------------------------------------------------
Map, ?> map = (Map, ?>) inputMap;
for (Map.Entry, ?> entry : map.entrySet()){
Object bean = entry.getValue();
if (null != bean){
MapUtil.putAllIfNotNull(propertyNameAndJsonValueProcessorMap, parseBean(bean));
}
}
//---------------------------------------------------------------
return propertyNameAndJsonValueProcessorMap;
}
//---------------------------------------------------------------
/**
* Parses the bean.
*
* @param javaBean
* the java bean
* @return the map
* @since 1.13.0
*/
private static Map parseBean(Object javaBean){
Class> klass = javaBean.getClass();
if (cache.containsKey(klass)){
return cache.get(klass);
}
//---------------------------------------------------------------
Map propertyNameAndJsonValueProcessorMap = buildObject(javaBean);
cache.put(klass, propertyNameAndJsonValueProcessorMap);
return propertyNameAndJsonValueProcessorMap;
}
//---------------------------------------------------------------
/**
* Builds the.
*
* @param klass
* the klass
* @return the map
* @since 1.11.5
*/
private static Map buildObject(Object javaBean){
Class> klass = javaBean.getClass();
if (equalsAny(klass, DontFindAnnotaionHelper.BASE_CLASS_ARRAY)){
return emptyMap();
}
if (equalsAny(klass, DontFindAnnotaionHelper.ARRAY_CLASS_ARRAY)){
return emptyMap();
}
if (ClassUtil.isInstanceAnyClass(javaBean, DontFindAnnotaionHelper.COMMON_CLASS_ARRAY)){
return emptyMap();
}
List list = getWithAnnotationName(klass, SensitiveWords.class);
if (isNullOrEmpty(list)){
return emptyMap();
}
//---------------------------------------------------------------------------------------------------------
//敏感字段
Map propertyNameAndJsonValueProcessorMap = newHashMap();
for (String propertyName : list){
propertyNameAndJsonValueProcessorMap.put(propertyName, SensitiveWordsJsonValueProcessor.INSTANCE);
}
return propertyNameAndJsonValueProcessorMap;
}
//---------------------------------------------------------------
/**
* Gets the with annotation name.
*
* @param
* the generic type
* @param klass
* the klass
* @param annotationCls
* the annotation cls
* @return the with annotation name
* @since 1.11.5
*/
private static List getWithAnnotationName(Class> klass,Class annotationCls){
List list = newArrayList();
addAllIgnoreNull(list, getFiledNamesWithAnnotation(klass, annotationCls));
addAllIgnoreNull(list, getPropertyNamesWithAnnotation(klass, annotationCls));
return list;
}
//---------------------------------------------------------------
/**
* Gets the filed names with annotation.
*
* @param
* the generic type
* @param klass
* the klass
* @param annotationCls
* the annotation cls
* @return the filed names with annotation
* @since 1.11.5
*/
private static List getFiledNamesWithAnnotation(Class> klass,Class annotationCls){
List list = newArrayList();
List fieldsList = FieldUtils.getFieldsListWithAnnotation(klass, annotationCls);
for (Field field : fieldsList){
list.add(field.getName());
}
return list;
}
//---------------------------------------------------------------
/**
* Gets the property names with annotation.
*
* @param
* the generic type
* @param klass
* the klass
* @param annotationCls
* the annotation cls
* @return 如果 klass PropertyDescriptors
是null或者empty,返回 {@link Collections#emptyList()}
* @since 1.11.5
*/
private static List getPropertyNamesWithAnnotation(Class> klass,Class annotationCls){
PropertyDescriptor[] propertyDescriptors = PropertyUtil.getPropertyDescriptors(klass);
if (isNullOrEmpty(propertyDescriptors)){
return emptyList();
}
//---------------------------------------------------------------
List list = newArrayList();
for (PropertyDescriptor propertyDescriptor : propertyDescriptors){
Method readMethod = propertyDescriptor.getReadMethod();
//since 1.12.2
if (null == readMethod){
LOGGER.warn(
"class:[{}],property:[{}],has no ReadMethod!!SKIPPED",
klass.getCanonicalName(),
propertyDescriptor.getDisplayName());
continue;
}
//---------------------------------------------------------------
A sensitiveWords = MethodUtils.getAnnotation(readMethod, annotationCls, true, true);
if (null != sensitiveWords){
list.add(propertyDescriptor.getName());
}
}
return list;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy