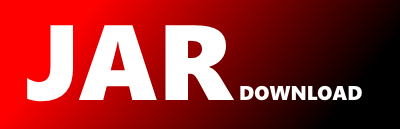
com.github.iintelligas.controller.PermissionRestController Maven / Gradle / Ivy
package com.github.iintelligas.controller;
import com.github.iintelligas.exception.DuplicateDataException;
import com.github.iintelligas.persist.dto.Permission;
import com.github.iintelligas.bean.PermissionWrapper;
import com.github.iintelligas.service.PermissionService;
import org.apache.commons.lang3.StringUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
import java.util.ArrayList;
import java.util.List;
@RestController
@RequestMapping("/admin/rest/profile")
public class PermissionRestController
{
private static final Logger log = LoggerFactory.getLogger(PermissionRestController.class);
private final PermissionService permissionService;
@Autowired
public PermissionRestController(PermissionService permissionService) {
this.permissionService = permissionService;
}
@GetMapping(value = "/findAllPermissions")
public @ResponseBody
ResponseEntity findAllPermissions()
{
try
{
if (log.isDebugEnabled())
{
log.debug("--------------------- #START PermissionRestController.findAllPermissions ----------------");
}
List permissions = permissionService.getPermissions();
if (log.isDebugEnabled())
{
log.debug("Number of permission found : " + permissions.size());
}
if (log.isDebugEnabled())
{
log.debug("--------------------- #END PermissionRestController.findAllPermissions ----------------");
}
return new ResponseEntity<>(new PermissionWrapper(permissions), HttpStatus.OK);
}
catch (Exception ex)
{
log.error("Unwanted exception.", ex);
return new ResponseEntity<>(HttpStatus.EXPECTATION_FAILED);
}
}
@PostMapping(value = "/addPermission")
public @ResponseBody
ResponseEntity addPermission(final @RequestBody Permission permission)
{
if (log.isDebugEnabled())
{
log.debug("--------------------- #START PermissionRestController.addPermission ----------------");
}
PermissionWrapper wrapper = new PermissionWrapper();
try
{
if (!validatePermissionRequest(permission))
{
wrapper.setMessage("Permission name is required.");
return new ResponseEntity<>(wrapper, HttpStatus.BAD_REQUEST);
}
List permissions = new ArrayList<>(1);
permissions.add(permissionService.addPermission(permission));
if (log.isDebugEnabled())
{
log.debug("--------------------- #END PermissionRestController.addPermission ----------------");
}
wrapper.setPermissions(permissions);
wrapper.setMessage("Successfully Added Permission : "+permission.getName());
return new ResponseEntity<>(wrapper, HttpStatus.OK);
}
catch (DuplicateDataException de) {
wrapper.setMessage("Permission "+permission.getName()+" already present. Please change it.");
return new ResponseEntity<>(wrapper, HttpStatus.NOT_ACCEPTABLE);
}
catch (Exception ex)
{
log.error("Unwanted exception.", ex);
return new ResponseEntity<>(HttpStatus.EXPECTATION_FAILED);
}
}
@PutMapping(value = "/updatePermission")
public @ResponseBody
ResponseEntity updatePermission(final @RequestBody Permission permission)
{
if (log.isDebugEnabled())
{
log.debug("--------------------- #START PermissionRestController.updatePermission ----------------");
}
PermissionWrapper wrapper = new PermissionWrapper();
try
{
if (!validatePermissionRequest(permission))
{
wrapper.setMessage("Permission name is required.");
return new ResponseEntity<>(wrapper, HttpStatus.BAD_REQUEST);
}
List permissions = new ArrayList<>(1);
Permission storedPermission = permissionService.getPermission(permission.getName());
if(storedPermission == null) {
wrapper.setMessage("Could not found permission "+permission.getName());
return new ResponseEntity<>(wrapper, HttpStatus.NOT_ACCEPTABLE);
}
storedPermission.setDescription(permission.getDescription());
storedPermission = permissionService.updatePermission(storedPermission);
permissions.add(storedPermission);
if (log.isDebugEnabled())
{
log.debug("--------------------- #END PermissionRestController.updatePermission ----------------");
}
wrapper.setPermissions(permissions);
wrapper.setMessage("Successfully updated Permission : "+permission.getName());
return new ResponseEntity<>(wrapper, HttpStatus.OK);
}
catch (DuplicateDataException de) {
wrapper.setMessage("Permission "+permission.getName()+" already present. Please change it.");
return new ResponseEntity<>(wrapper, HttpStatus.NOT_ACCEPTABLE);
}
catch (Exception ex)
{
log.error("Unwanted exception.", ex);
return new ResponseEntity<>(HttpStatus.EXPECTATION_FAILED);
}
}
@DeleteMapping(value = "/deletePermission")
public @ResponseBody
ResponseEntity deletePermission(final @RequestBody Permission permission)
{
if (log.isDebugEnabled())
{
log.debug("--------------------- #START PermissionRestController.deletePermission ----------------");
}
PermissionWrapper wrapper = new PermissionWrapper();
try
{
if (!validatePermissionRequest(permission))
{
wrapper.setMessage("Permission name is required.");
return new ResponseEntity<>(wrapper, HttpStatus.BAD_REQUEST);
}
Permission storedPermission = permissionService.getPermission(permission.getName());
if(storedPermission == null) {
wrapper.setMessage("Could not found permission "+permission.getName());
return new ResponseEntity<>(wrapper, HttpStatus.NOT_ACCEPTABLE);
}
permissionService.deletePermission(storedPermission.getId());
if (log.isDebugEnabled())
{
log.debug("--------------------- #END PermissionRestController.deletePermission ----------------");
}
wrapper.setMessage("Successfully deleted Permission : "+permission.getName());
return new ResponseEntity<>(wrapper, HttpStatus.OK);
}
catch (DuplicateDataException de) {
wrapper.setMessage("Permission "+permission.getName()+" already present. Please change it.");
return new ResponseEntity<>(wrapper, HttpStatus.NOT_ACCEPTABLE);
}
catch (Exception ex)
{
log.error("Unwanted exception.", ex);
return new ResponseEntity<>(HttpStatus.EXPECTATION_FAILED);
}
}
@PostMapping(value = "/addPermissions")
public @ResponseBody
ResponseEntity addPermissions(final @RequestBody PermissionWrapper permissions)
{
try
{
if (log.isDebugEnabled())
{
log.debug("--------------------- #START PermissionRestController.addPermissions ----------------");
}
if (!validatePermissionWrapperRequest(permissions))
{
permissions.getPermissions().clear();
permissions.setMessage("Permission name is required.");
return new ResponseEntity<>(permissions, HttpStatus.BAD_REQUEST);
}
for(Permission permission : permissions.getPermissions()) {
if(permissionService.getPermission(permission.getName()) != null) { //TODO need to change. Just one query using 'IN' to fetch data in one DB call.
permissions.getPermissions().clear();
permissions.setMessage("Permission "+ permission.getName()+" already present. Please change it.");
return new ResponseEntity<>(permissions, HttpStatus.NOT_ACCEPTABLE);
}
}
permissionService.addPermissions(permissions.getPermissions());
permissions.getPermissions().clear();
permissions.setMessage("Successfully added all permissions.");
if (log.isDebugEnabled())
{
log.debug("--------------------- #END PermissionRestController.addPermissions ----------------");
}
return new ResponseEntity<>(permissions, HttpStatus.OK);
}
catch (Exception ex)
{
log.error("Unwanted exception.", ex);
return new ResponseEntity<>(HttpStatus.EXPECTATION_FAILED);
}
}
private boolean validatePermissionWrapperRequest(PermissionWrapper permissions) {
if(permissions == null || permissions.getPermissions() == null || permissions.getPermissions().size() <= 0) {
return false;
}
for(Permission permission : permissions.getPermissions()) {
if(!validatePermissionRequest(permission)) {
return false;
}
}
return true;
}
private boolean validatePermissionRequest(Permission permission) {
return !(permission == null || StringUtils.isBlank(permission.getName()));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy