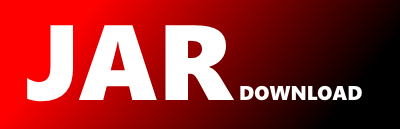
com.github.iintelligas.controller.RoleController Maven / Gradle / Ivy
package com.github.iintelligas.controller;
import com.github.iintelligas.exception.DuplicateDataException;
import com.github.iintelligas.exception.NoDataFoundException;
import com.github.iintelligas.persist.dto.Permission;
import com.github.iintelligas.persist.dto.Role;
import com.github.iintelligas.service.PermissionService;
import com.github.iintelligas.service.RoleService;
import org.apache.commons.collections4.CollectionUtils;
import org.apache.commons.lang3.StringUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.servlet.ModelAndView;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
@Controller
public class RoleController
{
private static final Logger logger = LoggerFactory.getLogger(RoleController.class);
private final RoleService roleService;
private final PermissionService permissionService;
@Autowired
public RoleController(RoleService roleService, PermissionService permissionService) {
this.roleService = roleService;
this.permissionService = permissionService;
}
@GetMapping("/profile-role-list")
public String landing(Model model)
{
populateRoles(model);
return "profile/profile-role-list";
}
private void populateRoles(Model model) {
List roles = roleService.getRoles();
model.addAttribute("roles", roles);
}
@GetMapping("/profile-role-add")
public String roleAdd(Model model)
{
Role role = new Role();
role.setPermissions(permissionService.getPermissions());
model.addAttribute("role", role);
return "profile/profile-role-add";
}
@PostMapping(value = "/profile-role-add-save")
public ModelAndView roleAddSave(@ModelAttribute Role role, @RequestParam String action)
{
if(logger.isDebugEnabled())
{
logger.debug("START - profile-role-add-save");
}
ModelAndView modelAndView ;
if("cancel".equals(action))
{
modelAndView = getModelAndViewForCancelFlow();
return modelAndView;
}
try
{
List selectedPermission = new ArrayList<>();
for(Permission perm : role.getPermissions())
{
if (perm.isSelected())
{
selectedPermission.add(perm);
}
}
role.getPermissions().clear();
if(CollectionUtils.isNotEmpty(selectedPermission)) {
role.setPermissions(selectedPermission);
}
role = roleService.addRole(role);
String message = "Role '" + role.getName() + "' successfully added";
modelAndView = getModelAndViewForCancelFlow();
modelAndView.addObject("successMessage", message);
}
catch (Exception e)
{
modelAndView = new ModelAndView("profile/profile-role-add");
modelAndView.addObject("role", role);
if (e instanceof DuplicateDataException)
{
modelAndView.addObject("errorMessage", e.getMessage());
}
logger.error(e.getMessage(), e);
}
if(logger.isDebugEnabled())
{
logger.debug("END - profile-role-add-save");
}
return modelAndView;
}
@GetMapping("/profile-role-update")
public String roleEdit(Model model, @RequestParam(value = "id") String id)
{
if(StringUtils.isBlank(id))
{
model.addAttribute("errorMessage", "Invalid Role Id. Please provide proper Role Id to Edit.");
return "profile/profile-role-update";
}
Long idLong;
try
{
idLong = Long.valueOf(id);
}
catch (NumberFormatException e)
{
model.addAttribute("errorMessage", "Invalid Role Id. Please provide proper Role Id to Edit.");
return "profile/profile-role-update";
}
Role role = roleService.getRole(idLong);
if(role == null)
{
model.addAttribute("errorMessage", "Could not found Role with Id : "+id);
return "profile/profile-role-update";
}
Map stringPermissionMap = new HashMap<>();
if(CollectionUtils.isNotEmpty(role.getPermissions()))
{
for (Permission selectedPermission : role.getPermissions())
{
stringPermissionMap.put(selectedPermission.getId(), selectedPermission);
}
}
List storedPermissions = permissionService.getPermissions();
List allPermissions = new ArrayList<>(storedPermissions.size());
for(Permission perm : storedPermissions)
{
if (stringPermissionMap.get(perm.getId()) != null)
{
perm.setSelected(true);
allPermissions.add(perm);
}
else
{
perm.setSelected(false);
allPermissions.add(perm);
}
}
role.getPermissions().clear();
role.setPermissions(allPermissions);
model.addAttribute("role", role);
return "profile/profile-role-update";
}
@PostMapping(value = "/profile-role-update-save")
public ModelAndView roleUpdateSave(@ModelAttribute Role role, @RequestParam String action)
{
if(logger.isDebugEnabled())
{
logger.debug("START - profile-role-update-save");
}
ModelAndView modelAndView ;
if("cancel".equals(action))
{
modelAndView = getModelAndViewForCancelFlow();
return modelAndView;
}
try
{
List selectedPermission = new ArrayList<>();
for(Permission perm : role.getPermissions())
{
if (perm.isSelected())
{
selectedPermission.add(perm);
}
}
role.getPermissions().clear();
if(CollectionUtils.isNotEmpty(selectedPermission)) {
role.setPermissions(selectedPermission);
}
role = roleService.updateRole(role);
String message = "Role '" + role.getName() + "' successfully updated";
modelAndView = getModelAndViewForCancelFlow();
modelAndView.addObject("successMessage", message);
}
catch (Exception e)
{
Map params=new HashMap<>();
params.put("id", String.valueOf(role.getId()));
modelAndView = new ModelAndView("profile/profile-role-update", params);
modelAndView.addObject("role", role);
if (e instanceof DuplicateDataException)
{
modelAndView.addObject("errorMessage", e.getMessage());
}
logger.error(e.getMessage(), e);
}
if(logger.isDebugEnabled())
{
logger.debug("END - profile-role-update-save");
}
return modelAndView;
}
@GetMapping("/profile-role-delete")
public String roleDelete(Model model, @RequestParam(value = "id") String id)
{
if(StringUtils.isBlank(id))
{
model.addAttribute("errorMessage", "Invalid Role Id. Please provide proper Role Id to delete.");
populateRoles(model);
return "profile/profile-role-list";
}
Long idLong;
try
{
idLong = Long.valueOf(id);
}
catch (NumberFormatException e)
{
model.addAttribute("errorMessage", "Invalid Role Id. Please provide proper Role Id to delete.");
populateRoles(model);
return "profile/profile-role-list";
}
try {
roleService.deleteRole(idLong);
populateRoles(model);
model.addAttribute("successMessage", "Successfully deleted Role.");
return "profile/profile-role-list";
}
catch (NoDataFoundException e)
{
populateRoles(model);
model.addAttribute("errorMessage", e.getMessage());
return "profile/profile-role-list";
}
}
private ModelAndView getModelAndViewForCancelFlow() {
ModelAndView modelAndView = new ModelAndView("profile/profile-role-list");
List roles = roleService.getRoles();
modelAndView.addObject("roles", roles);
return modelAndView;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy